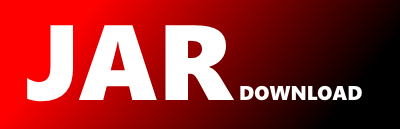
com.gitee.qdbp.staticize.parse.ExpParser Maven / Gradle / Ivy
package com.gitee.qdbp.staticize.parse;
import com.gitee.qdbp.staticize.exception.TagException;
import com.gitee.qdbp.staticize.utils.TagUtils;
/**
* 表达式解析器
*
* @author zhaohuihua
* @version 20200930
*/
public class ExpParser {
public static Object parse(String string) throws TagException {
if (string == null || string.isEmpty()) {
return string;
}
char[] chars = string.toCharArray();
ExpCollector exp = null;
ExpItems items = new ExpItems();
int index = 0;
int size = chars.length;
boolean evaluatable = false;
boolean escapable = false;
for (int i = 0; i < size; i++) {
char c = chars[i];
// 正在取表达式
if (exp != null) {
if (exp.isStringStatus() || c != '}') {
exp.parse(c);
continue;
}
}
// 表达式标签开始
if (c == '{') {
// 当前字符是{, 上一字符是#$, 表示遇到一个表达式了
char last = i > 0 ? chars[i - 1] : 0;
if (last == '#' || last == '$') {
// 上上一字符如果是\, 作为转义字符处理
if (i > 1 && chars[i - 2] == '\\') {
escapable = true;
if (index < i - 2) {
// 处理斜杠\前面的所有字符, i - 2 跳过斜杠
String s = TagUtils.substring(chars, index, i - 2);
items.add(new ExpItem(s, false));
}
index = i - 1; // 从上一字符开始
} else {
exp = new ExpCollector();
exp.parse(last);
exp.parse(c);
if (index < i - 1) {
String s = TagUtils.substring(chars, index, i - 1);
items.add(new ExpItem(s, false));
}
// 从上一字符开始, 都要算在表达式内, 所以-1
index = i - 1;
}
}
}
// 表达式标签结束
else if (c == '}') {
// 正在取表达式, 遇到}, 表示表达式结束了
if (exp != null) {
exp.parse(c);
// 创建一个表达式标签
items.add(new ExpItem(exp.getContent(), evaluatable = true));
// 当前字符已处理完, index从下一字符开始, 所以+1
index = i + 1;
exp = null;
}
}
}
if (exp != null) {
throw new TagException("The expression is not properly close: " + exp.getContent());
}
if (index == 0 || (!evaluatable && !escapable)) {
// 没有表达式且没有转义符
return string;
}
if (index < size) {
String s = TagUtils.substring(chars, index, size);
items.add(new ExpItem(s, false));
}
if (!evaluatable) {
// 没有表达式
return items.toString();
}
if (items.size() == 1) {
return items.get(0);
} else {
return items;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy