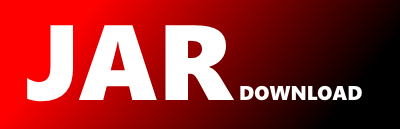
com.gitee.qdbp.staticize.publish.BasePublisher Maven / Gradle / Ivy
package com.gitee.qdbp.staticize.publish;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.gitee.qdbp.staticize.common.IContext;
import com.gitee.qdbp.staticize.common.IMetaData;
import com.gitee.qdbp.staticize.common.NodeMetaData;
import com.gitee.qdbp.staticize.exception.TagException;
import com.gitee.qdbp.staticize.parse.AttrData;
import com.gitee.qdbp.staticize.parse.AttrSetter;
import com.gitee.qdbp.staticize.tags.base.ITag;
import com.gitee.qdbp.staticize.tags.base.NextStep;
import com.gitee.qdbp.staticize.tags.base.Taglib;
/**
* 根据标签数据发布一个页面
* 不支持多线程
*
* @author zhaohuihua
* @version 20200816
*/
public abstract class BasePublisher {
/** 解析后的标签元数据的根节点 **/
private final IMetaData root;
/** 当前处理节点 **/
private NodeMetaData curr;
/**
* 构造函数
*
* @param root 根节点
*/
public BasePublisher(IMetaData root) {
this.root = root;
}
/** 标签元数据的根节点 **/
public IMetaData getRootNode() {
return root;
}
/** 标签元数据的当前节点 **/
public NodeMetaData getCurrentNode() {
return curr;
}
/**
* 根据标签数据发布一个页面
*
* @param context 上下文对象
* @throws TagException 标签错误
* @throws IOException 写文件失败
*/
protected void publish(IContext context) throws TagException, IOException {
// 给context设置Taglib, 以便通过Taglib获取配置信息
Taglib taglib = root.getTaglib();
if (context instanceof Taglib.Aware) {
((Taglib.Aware) context).setTaglib(taglib);
}
// 循环处理ROOT标签下的子标签
List children = root.getChildren();
// root
Class extends ITag> clazz = root.getTagClass();
ITag tag = taglib.newTagInstance(clazz);
try {
if (children != null && !children.isEmpty()) {
for (NodeMetaData i : children) {
publish(context, i, tag);
}
}
} catch (TagException e) {
if (this.curr != null) {
e.prependMessage(this.curr.getRealPath() + ' ');
}
throw e;
}
}
protected void publish(IContext context, NodeMetaData metadata, ITag parent) throws TagException {
this.curr = metadata;
int row = metadata.getRow();
int column = metadata.getColumn();
String name = metadata.getName();
// 创建标签处理对象
Class extends ITag> tagType = metadata.getTagClass();
Taglib taglib = root.getTaglib();
ITag tag = taglib.newTagInstance(tagType);
// 设置基础数据
tag.doSetBaseData(parent, context);
// 给标签设置属性
List attrs = metadata.getAttributes();
Map map = new HashMap<>();
for (AttrData entry : attrs) {
// 字段名
String fieldName = entry.getKey();
// 字段值: ExpItem/ExpItems/其他
Object fieldValue = entry.getValue();
// 计算字段值
Object result = doEvalValue(tag, metadata, fieldName, fieldValue);
// 给标签设置属性值
doSetValue(tag, metadata, fieldName, result);
// 记录下全部属性值, 用于检验和设置动态参数
map.put(fieldName, result);
}
// 设置动态参数
String paramName = AttrSetter.instance.getDynamicName(tagType);
if (paramName != null) {
tag.stack().put(paramName, map);
}
// 调用标签的校验方法
try {
tag.doValidate(metadata);
} catch (TagException e) {
e.prependMessage(String.format("%s:%s, <%s>, ", row, column, name));
throw e;
}
NextStep next;
do {
// 调用标签的内容处理方法
try {
next = tag.doHandle();
} catch (TagException e) {
e.prependMessage(String.format("%s:%s, <%s>, ", row, column, name));
throw e;
} catch (Exception e) {
String msg = String.format("%s:%s, <%s>, tag execute error.", row, column, name);
throw new TagException(msg, e);
}
// 递归处理子标签
if (next == NextStep.EVAL_BODY || next == NextStep.LOOP_BODY) {
List children = metadata.getChildren();
if (children != null && !children.isEmpty()) {
for (NodeMetaData i : children) {
publish(context, i, tag);
}
}
}
// 调用标签的结束处理方法
try {
tag.doEnded(next);
} catch (TagException e) {
e.prependMessage(String.format("%s:%s, %s>, ", row, column, name));
throw e;
} catch (Exception e) {
String msg = String.format("%s:%s, %s>, tag execute error.", row, column, name);
throw new TagException(msg, e);
}
} while (next == NextStep.LOOP_BODY); // 循环标签
}
protected Object doEvalValue(ITag tag, NodeMetaData metadata, String fieldName, Object fieldValue)
throws TagException {
if (fieldValue == null) {
return null;
}
try {
return tag.doEvalValue(fieldName, fieldValue);
} catch (TagException e) {
int row = metadata.getRow();
int col = metadata.getColumn();
String name = metadata.getName();
String exp = fieldValue.toString();
e.prependMessage(String.format("%s:%s, <%s>, %s=\"%s\", ", row, col, name, fieldName, exp));
throw e;
}
}
protected void doSetValue(ITag tag, NodeMetaData data, String field, Object value) throws TagException {
try {
AttrSetter.instance.setAttrValue(tag, field, value);
} catch (TagException e) {
int row = data.getRow();
int col = data.getColumn();
String name = data.getName();
e.prependMessage(String.format("%s:%s, <%s>, ", row, col, name));
throw e;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy