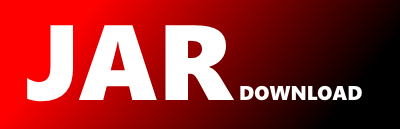
com.gitee.qdbp.staticize.task.StaticizeTask Maven / Gradle / Ivy
package com.gitee.qdbp.staticize.task;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import com.gitee.qdbp.staticize.io.IReaderCreator;
import com.gitee.qdbp.staticize.io.IWriterCreator;
import com.gitee.qdbp.staticize.tags.base.Taglib;
/**
* 静态化任务
*
* @author zhaohuihua
* @version 140722
*/
public class StaticizeTask {
/** 最多线程数 **/
protected int thmax = 10;
/** 最多积压数, 超过这个数则增加线程 **/
protected int szmax = 50;
/** 任务ID序列号 **/
private static int id;
/** 任务ID **/
private final String taskId;
/** 是否取消(用户主动取消) **/
boolean cancel;
/** 数据是否加载完毕(如果是, 表示不会再有新的数据进来了) **/
boolean done;
/** 是否结束, 所有线程全部执行结束 **/
private boolean stoped;
/** 是否已经开始 **/
private boolean started;
/** 当前已经完成的数量 **/
int finished;
/** 当前失败的数量 **/
int failed;
/** 当前的任务总数 **/
private int total;
/** 从上次增加线程以来新增加的任务 **/
private int newtask;
/** 线程池 **/
List threads;
/** 任务池 **/
private final LinkedList list;
/** 监听器容器 **/
private final List listeners;
protected final Taglib taglib;
protected final IReaderCreator input;
protected final IWriterCreator output;
protected final TemplateCache cache;
/**
* 静态化任务构造函数, 线程数
*
* @param taglib 标签库
* @param input 模板读取接口
* @param output 输出流构建接口
*/
public StaticizeTask(Taglib taglib, IReaderCreator input, IWriterCreator output) {
done = false;
started = false;
stoped = false;
list = new LinkedList();
threads = new ArrayList();
listeners = new ArrayList();
this.taglib = taglib;
this.input = input;
this.output = output;
this.cache = new TemplateCache(this.taglib, this.input);
this.taskId = String.valueOf(++id);
TaskThread item = new TaskThread(this);
threads.add(item);
item.setName("Staticize-" + taskId + "-" + threads.size());
}
/**
* 获取任务ID
*
* @return 任务ID
*/
public String getId() {
return taskId;
}
/**
* 启动任务
*
* @author zhaohuihua
*/
public void start() {
synchronized (this) {
if (this.started) {
throw new IllegalStateException("Publish task has started.");
}
this.started = true;
}
for (TaskThread thread : threads) {
thread.start();
}
}
/**
* 结束任务(由TaskThread执行结束时调用)
*
* @author zhaohuihua
*/
synchronized void stop(TaskThread thread) {
threads.remove(thread);
// 所有线程已经全部结束了
if (threads.isEmpty()) {
// 通知监听器
for (StaticizeListener listener : listeners) {
listener.over();
}
// 设置结束标志
this.stoped = true;
}
}
/**
* 取消任务
*
* @author zhaohuihua
*/
public void cancel() {
synchronized (this) {
if (!this.started) {
throw new IllegalStateException("Publish task is unstart.");
}
this.cancel = true;
}
notifys();
}
/**
* 数据加载完毕(如果是, 表示不会再有新的数据进来了)
*
* @author zhaohuihua
*/
public void done() {
this.done = true;
notifys();
}
/**
* 获取当前的任务总数
*
* @return 总数
*/
public int getTotal() {
return total;
}
/**
* 获取当前已经完成的数量
*
* @return 完成数
*/
public int getFinished() {
return finished;
}
/**
* 获取当前失败的数量
*
* @return 失败数
*/
public int getFailed() {
return failed;
}
/**
* 增加任务监听器
*
* @param listener 监听器
*/
public void addListener(StaticizeListener listener) {
this.listeners.add(listener);
}
/**
* 增加任务
*
* @param name 任务名称
* @param template 模板路径
* @param out 输出路径
*/
public void add(String name, String template, String out) {
add(name, null, template, out);
}
/**
* 增加任务
*
* @param name 任务名称
* @param preset 预置数据
* @param template 模板路径
* @param output 输出路径
*/
public void add(String name, Map preset, String template, String output) {
if (stoped) {
throw new IllegalStateException("Staticize task has stoped.");
}
TaskData data = new TaskData(name);
data.setPreset(preset);
data.setTemplate(template);
data.setOutput(output);
synchronized (this) {
total++;
newtask++;
list.addLast(data);
// 未达到最大线程数, 已达到最大积压数
// 且距离上次增加线程之后又至少增加了一定数量
if (threads.size() < thmax && list.size() >= szmax && newtask >= szmax) {
newtask = 0;
// 增加新的处理线程
TaskThread item = new TaskThread(this);
threads.add(item);
item.setName("Staticize-" + taskId + "-" + threads.size());
// 其他线程已经启动了, 则新增加的线程立即启动
if (this.started) {
item.start();
}
}
}
notifys();
}
/** 获取最老的一条数据, 由TaskThread调用 **/
synchronized TaskData pop() {
return list.isEmpty() ? null : list.removeFirst();
}
/** 唤醒所有线程 **/
private synchronized void notifys() {
for (TaskThread thread : threads) {
synchronized (thread) {
thread.notify();
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy