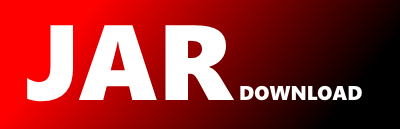
com.gitee.sergius.apitool.web.html.HtmlOperater Maven / Gradle / Ivy
package com.gitee.sergius.apitool.web.html;
import com.gitee.sergius.apitool.constant.Constant;
import com.gitee.sergius.apitool.entity.RequestParamEntity;
import com.gitee.sergius.apitool.entity.ResponseParamEntity;
import com.gitee.sergius.apitool.entity.StatusCodeEntity;
import com.gitee.sergius.apitool.constant.DataType;
import com.gitee.sergius.apitool.entity.SubEntity;
import org.springframework.util.CollectionUtils;
import org.springframework.util.StringUtils;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
/**
* @author shawn yang
* @version 1.0.0
* 用来生成API展示页面html
*
*/
public class HtmlOperater {
/**
* 获取style部分
*/
public static String getStylePart(){
return "";
}
/**
* 获取script部分
*/
public static String getScriptPart(){
return "";
}
/**
* 获取body部分
* @param subEntity 接口封装实体
*/
public static String getBodyPart(SubEntity subEntity){
StringBuilder result = new StringBuilder();
result.append(getUrlPart(subEntity));
result.append(" ");
return result.toString();
}
/**
* 异常说明
* @param subEntity 接口封装实体
*/
private static StringBuilder getExceptionPart(SubEntity subEntity){
StringBuilder reqHtml = new StringBuilder();
List statusCodeEntityList = subEntity.getStatusCodeEntityList();
if(!CollectionUtils.isEmpty(statusCodeEntityList)){
reqHtml.append(" 返回码说明 ")
.append(" ")
.append(" 返回码 说明 ")
.append(" ");
for(StatusCodeEntity statusCodeEntity: statusCodeEntityList){
reqHtml.append(" "+statusCodeEntity.getCode()+" "+statusCodeEntity.getDesc()+" ");
}
reqHtml.append("
");
}
return reqHtml;
}
/**
* 出参示例
* @param subEntity 接口封装实体
*/
private static StringBuilder getOutExamplePart(SubEntity subEntity){
StringBuilder resHtml = new StringBuilder();
List responseParamEntityList = subEntity.getResponseParamEntityList();
if(!CollectionUtils.isEmpty(responseParamEntityList)){
boolean isBaseReturn = Constant.DEFAULT_UNDEFINE_NAME.equals(responseParamEntityList.get(0).getName());
resHtml.append(" 出参示例 ");
resHtml.append(" ");
if(!isBaseReturn){
resHtml.append(" {
");
}
resHtml.append(getOutExampleRowPart(responseParamEntityList,isBaseReturn));
if(!isBaseReturn) {
resHtml.append(" }
");
}
resHtml.append(" ");
}
return resHtml;
}
private static StringBuilder getOutExampleRowPart(List responseParamEntityList,boolean isBaseReturn){
StringBuilder html = new StringBuilder();
if(!CollectionUtils.isEmpty(responseParamEntityList)) {
for(int i=0,len = responseParamEntityList.size();i" + nameHtml + getExampleHtml(type,example,entity.isArray()));
}else{
if(!entity.isArray()){
html.append(" " + nameHtml + "{" + "
");
html.append(getOutExampleRowPart(entity.getChildList(),false));
html.append(" }");
}else{
html.append("
" + nameHtml + "[{" + "
");
html.append(getOutExampleRowPart(entity.getChildList(),false));
html.append(" },{...}]");
}
}
if(i==len-1){
html.append( "
");
}else{
html.append( ", ");
}
}
}
return html;
}
/**
* 入参示例
* @param subEntity
* @return
*/
private static StringBuilder getInExamplePart(SubEntity subEntity){
StringBuilder reqHtml = new StringBuilder();
List requestParamEntityList = subEntity.getRequestParamEntityList();
if(!CollectionUtils.isEmpty(requestParamEntityList)){
boolean isBaseReturn = Constant.DEFAULT_UNDEFINE_NAME.equals(requestParamEntityList.get(0).getName());
reqHtml.append(" 入参示例 ");
reqHtml.append(" ");
if(!isBaseReturn){
reqHtml.append(" {
");
}
reqHtml.append(getInExampleRowPart(requestParamEntityList,isBaseReturn));
if(!isBaseReturn) {
reqHtml.append(" }
");
}
reqHtml.append(" ");
}
return reqHtml;
}
private static StringBuilder getInExampleRowPart(List requestParamEntityList,boolean isBaseReturn){
StringBuilder html = new StringBuilder();
if(!CollectionUtils.isEmpty(requestParamEntityList)) {
for(int i=0,len = requestParamEntityList.size();i" + nameHtml + getExampleHtml(type,example,entity.isArray()));
}else{
if(!entity.isArray()){
html.append(" " + nameHtml + "{" + "
");
html.append(getInExampleRowPart(entity.getChildList(),false));
html.append(" }");
}else{
html.append("
" + nameHtml + "[{" + "
");
html.append(getInExampleRowPart(entity.getChildList(),false));
html.append(" },{...}]");
}
}
if(i==len-1){
html.append( "
");
}else{
html.append( ", ");
}
}
}
return html;
}
/**
* 基础类型html
* @param type
* @param example
* @param isArray
*/
private static String getExampleHtml(String type,String example,boolean isArray){
String result ;
DataType[] dataTypes = DataType.values();
DataType exampleType = DataType.OTHER;
for(DataType dataType : dataTypes){
if(dataType.getName().equals(type)){
exampleType = dataType;
}
}
if(StringUtils.isEmpty(example)){
example = exampleType.getDefaultHtml();
}
if(exampleType.isWithQuotation()){
example = "\"" + example + "\"";
}
if(!isArray){
result = example;
}else{
result = "["+example+",...]";
}
return result;
}
/**
* 出参说明
* @param subEntity
* @return
*/
private static StringBuilder getOutParamPart(SubEntity subEntity){
StringBuilder reqHtml = new StringBuilder();
List responseParamEntityList = subEntity.getResponseParamEntityList();
if(!CollectionUtils.isEmpty(responseParamEntityList)){
reqHtml.append(" 出参说明 ");
reqHtml.append(" ");
reqHtml.append(" 参数名 类型 必填 说明 ");
reqHtml.append(getOutParamTrPart(responseParamEntityList));
reqHtml.append("
");
}
return reqHtml;
}
private static StringBuilder getOutParamTrPart(List responseParamEntityList){
StringBuilder html = new StringBuilder();
if(!CollectionUtils.isEmpty(responseParamEntityList)) {
for (ResponseParamEntity entity : responseParamEntityList) {
String style = "style='text-indent: " + entity.getLevel()*2 + "em;'";
String name = entity.getName();
String type = entity.getType() + (entity.isArray() ? "[]" : "");
String isRequired = (entity.isRequired() ? "是" : "否");
String desc = entity.getDesc();
if(Constant.DEFAULT_UNDEFINE_NAME.equals(name)){
name = "";
desc = "";
}
html.append(" " + name + " " + type + " " + isRequired + " " + desc + " ");
if (entity.isHasChild()) {
html.append(getOutParamTrPart(entity.getChildList()));
}
}
}
return html;
}
/**
* 入参说明
* @param subEntity
* @return
*/
private static StringBuilder getInParamPart(SubEntity subEntity){
StringBuilder reqHtml = new StringBuilder();
List requestParamEntityList = subEntity.getRequestParamEntityList();
if(!CollectionUtils.isEmpty(requestParamEntityList)){
reqHtml.append(" 入参说明 ");
reqHtml.append(" ");
reqHtml.append(" 参数名 类型 必填 说明 ");
reqHtml.append(getInParamTrPart(requestParamEntityList));
reqHtml.append("
");
}
return reqHtml;
}
private static StringBuilder getInParamTrPart(List requestParamEntityList){
StringBuilder html = new StringBuilder();
if(!CollectionUtils.isEmpty(requestParamEntityList)) {
for (RequestParamEntity entity : requestParamEntityList) {
String style = "style='text-indent: " + entity.getLevel()*2 + "em;'";
String name = entity.getName();
String type = entity.getType() + (entity.isArray() ? "[]" : "");
String isRequired = (entity.isRequired() ? "是" : "否");
String desc = entity.getDesc();
if(Constant.DEFAULT_UNDEFINE_NAME.equals(name)){
name = "";
desc = "";
}
html.append(" " + name + " " + type + " " + isRequired + " " + desc + " ");
if (entity.isHasChild()) {
html.append(getInParamTrPart(entity.getChildList()));
}
}
}
return html;
}
/**
* 接口描述部分
* @param subEntity
* @return
*/
private static StringBuilder getDescPart(SubEntity subEntity){
StringBuilder reqHtml = new StringBuilder();
Long generateTimeLong = subEntity.getGenerateTime();
Date generateTime = new Date(generateTimeLong);
String generateTimeFormated = new SimpleDateFormat(("yyyy-MM-dd HH:mm")).format(generateTime);
reqHtml.append(" 请求地址 ");
reqHtml.append(" ");
reqHtml.append(" 生成时间 "+generateTimeFormated+" ");
reqHtml.append(" 数据格式 "+subEntity.getReqContentType()+" ");
reqHtml.append(" 请求地址 "+subEntity.getUrl()+" ");
reqHtml.append("
");
return reqHtml;
}
/**
* 接口头部分
* @param subEntity
* @return
*/
private static StringBuilder getUrlPart(SubEntity subEntity){
StringBuilder reqHtml = new StringBuilder();
reqHtml.append(" " )
.append(" ")
.append(" ");
String method = subEntity.getMethod();
String btnStyle = "btn-default";
if("GET".equals(subEntity.getMethod())){
btnStyle = "btn-success";
}else if("POST".equals(subEntity.getMethod())){
btnStyle = "btn-info";
}
reqHtml.append(" ");
reqHtml.append(" "+subEntity.getUrl()+" ")
.append(" "+subEntity.getDesc()+" ")
.append(" ");
return reqHtml;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy