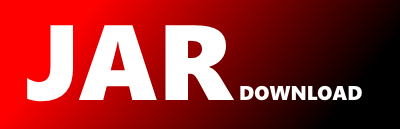
com.gitee.summer9102.develop.common.util.RandomUtil Maven / Gradle / Ivy
package com.gitee.summer9102.develop.common.util;
import com.gitee.summer9102.develop.common.consts.RandomType;
import java.nio.charset.StandardCharsets;
import java.util.List;
import java.util.concurrent.ThreadLocalRandom;
public class RandomUtil {
/**
* 随机字符串因子
* copy from mica:https://github.com/lets-mica/mica/blob/master/mica-core/src/main/java/net/dreamlu/mica/core/utils/StringUtil.java#L45
*/
private static final String INT_STR = "0123456789";
private static final String STR_STR = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
private static final String ALL_STR = INT_STR + STR_STR;
/**
* 随机数生成
* copy from mica:https://github.com/lets-mica/mica/blob/master/mica-core/src/main/java/net/dreamlu/mica/core/utils/StringUtil.java#L409
*
* @param count 字符长度
* @return 随机数
*/
public static String random(int count) {
return random(count, RandomType.ALL);
}
/**
* 随机数生成
* copy from mica:https://github.com/lets-mica/mica/blob/master/mica-core/src/main/java/net/dreamlu/mica/core/utils/StringUtil.java#L420
*
* @param count 字符长度
* @param randomType 随机数类别
* @return 随机数
*/
public static String random(int count, RandomType randomType) {
if (count <= 0) {
return "";
}
final ThreadLocalRandom random = ThreadLocalRandom.current();
char[] buffer = new char[count];
for (int i = 0; i < count; i++) {
if (RandomType.INT == randomType) {
buffer[i] = INT_STR.charAt(random.nextInt(INT_STR.length()));
} else if (RandomType.STRING == randomType) {
buffer[i] = STR_STR.charAt(random.nextInt(STR_STR.length()));
} else {
buffer[i] = ALL_STR.charAt(random.nextInt(ALL_STR.length()));
}
}
return new String(buffer);
}
/**
* 范围随机数
*
* @param min 最小
* @param max 最大(不包含)
* @return 随机值
*/
public static int random(int min, int max) {
int diff = max - min;
if (diff <= 0) {
return 0;
} else if (diff == 1) {
return min;
} else {
final ThreadLocalRandom random = ThreadLocalRandom.current();
return random.nextInt(diff) + min;
}
}
/**
* 生成uuid,采用 jdk 9 的形式,优化性能
* copy from mica:https://github.com/lets-mica/mica/blob/master/mica-core/src/main/java/net/dreamlu/mica/core/utils/StringUtil.java#L335
* 关于mica uuid生成方式的压测结果,可以参考:https://github.com/lets-mica/mica-jmh/wiki/uuid
*
* @return UUID
*/
public static String getUUID() {
ThreadLocalRandom random = ThreadLocalRandom.current();
long lsb = random.nextLong();
long msb = random.nextLong();
byte[] buf = new byte[32];
formatUnsignedLong(lsb, buf, 20, 12);
formatUnsignedLong(lsb >>> 48, buf, 16, 4);
formatUnsignedLong(msb, buf, 12, 4);
formatUnsignedLong(msb >>> 16, buf, 8, 4);
formatUnsignedLong(msb >>> 32, buf, 0, 8);
return new String(buf, StandardCharsets.UTF_8);
}
/**
* copy from mica:https://github.com/lets-mica/mica/blob/master/mica-core/src/main/java/net/dreamlu/mica/core/utils/StringUtil.java#L348
*/
private static void formatUnsignedLong(long val, byte[] buf, int offset, int len) {
int charPos = offset + len;
int radix = 1 << 4;
int mask = radix - 1;
do {
buf[--charPos] = DIGITS[((int) val) & mask];
val >>>= 4;
} while (charPos > offset);
}
/**
* All possible chars for representing a number as a String
* copy from mica:https://github.com/lets-mica/mica/blob/master/mica-core/src/main/java/net/dreamlu/mica/core/utils/NumberUtil.java#L113
*/
private final static byte[] DIGITS = {
'0', '1', '2', '3', '4', '5', '6', '7', '8', '9',
'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j',
'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't',
'u', 'v', 'w', 'x', 'y', 'z',
'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J',
'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T',
'U', 'V', 'W', 'X', 'Y', 'Z'
};
/**
* 随机取集合中的一个元素
*
* @param list
* @param
* @return
*/
public static T oneByList(List list) {
if (list == null || list.size() == 0) {
return null;
} else if (list.size() == 1) {
return list.get(0);
} else {
final ThreadLocalRandom random = ThreadLocalRandom.current();
return list.get(random.nextInt(list.size()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy