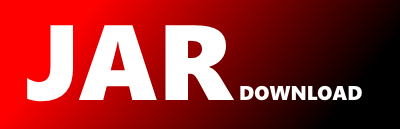
com.gitee.wenbo0.DictTextResultSetHandlerPlugin Maven / Gradle / Ivy
package com.gitee.wenbo0;
import com.baomidou.mybatisplus.core.toolkit.StringUtils;
import com.gitee.wenbo0.annotation.DictText;
import org.apache.ibatis.executor.resultset.DefaultResultSetHandler;
import org.apache.ibatis.executor.resultset.ResultSetHandler;
import org.apache.ibatis.mapping.MappedStatement;
import org.apache.ibatis.plugin.Interceptor;
import org.apache.ibatis.plugin.Intercepts;
import org.apache.ibatis.plugin.Invocation;
import org.apache.ibatis.plugin.Signature;
import org.apache.ibatis.session.Configuration;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.lang.reflect.Field;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.Statement;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
/**
* @author wenbo
* @since 2022/7/1 15:31
*/
@Intercepts({@Signature(type = ResultSetHandler.class, method = "handleResultSets", args = {Statement.class})})
public class DictTextResultSetHandlerPlugin implements Interceptor {
private final String DICT_SQL = "select %s from %s where %s = '%s' ";
private final String OTHER_WHERE = "and %s = '%s' ";
private final String COMMASEPARATE_SQL = "select group_concat(%s) from %s where %s in ('%s') ";
@Override
public Object intercept(Invocation invocation) throws Throwable {
Object proceed = invocation.proceed();
Object drsh = invocation.getTarget();
if (proceed != null && drsh instanceof DefaultResultSetHandler) {
if (!(proceed instanceof List))
return proceed;
DefaultResultSetHandler defaultResultSetHandler = (DefaultResultSetHandler) drsh;
MappedStatement ms = (MappedStatement) getFieldValueByObject(defaultResultSetHandler, "mappedStatement");
if (ms == null || ms.getResultMaps().size() != 1)
return proceed;
Class> type = ms.getResultMaps().get(0).getType();
for (Object obj : (List) proceed) {
if (type.isInstance(obj)) {
Field[] fields = type.getDeclaredFields();
for (Field field : fields) {
field.setAccessible(true);
Object value = field.get(obj);
if (value == null)
continue;
if (field.getAnnotation(DictText.class) != null) {
if (handleDictField(defaultResultSetHandler, obj, fields, field, value)) continue;
}
field.setAccessible(false);
}
}
}
}
return proceed;
}
private boolean handleDictField(DefaultResultSetHandler defaultResultSetHandler, Object obj, Field[] fields, Field field, Object value) throws Exception {
DictText dictText = field.getAnnotation(DictText.class);
String tableName = dictText.tableName();
String keyColumn = dictText.keyColumn();
String textColumn = dictText.textColumn();
String target = dictText.target();
if (StringUtils.isBlank(tableName) || StringUtils.isBlank(target))
return true;
String otherColumn = dictText.otherColumn();
String otherValue = dictText.otherValue();
boolean commaSeparate = dictText.commaSeparate();
Configuration configuration = (Configuration) getFieldValueByObject(defaultResultSetHandler, "configuration");
if (configuration == null)
return true;
SqlSessionFactoryBuilder sqlSessionFactoryBuilder = new SqlSessionFactoryBuilder();
SqlSessionFactory sqlSessionFactory = sqlSessionFactoryBuilder.build(configuration);
try (SqlSession session = sqlSessionFactory.openSession()) {
Connection conn = session.getConnection();
String foreignText, sql;
//不需要其他字段条件时
if (StringUtils.isBlank(otherValue)) {
//有逗号分割时
if (commaSeparate) {
String replace = value.toString().replace(",", "','");
sql = String.format(COMMASEPARATE_SQL, textColumn, tableName, keyColumn, replace);
} else {
sql = String.format(DICT_SQL, textColumn, tableName, keyColumn, value);
}
} else {
//有逗号分割时
if (commaSeparate) {
String replace = value.toString().replace(",", "','");
sql = String.format(COMMASEPARATE_SQL + OTHER_WHERE, textColumn,
tableName, keyColumn, replace, otherColumn, otherValue);
} else {
sql = String.format(DICT_SQL + OTHER_WHERE, textColumn, tableName,
keyColumn, value, otherColumn, otherValue);
}
}
ResultSet rs = conn.prepareStatement(sql).executeQuery();
if (rs.next()) {
foreignText = rs.getString(1);
} else {
foreignText = null;
}
List collect = Arrays.stream(fields).filter(field1 -> field1.getName().equals(target)).collect(Collectors.toList());
if (collect.size() > 0) {
Field targetField = collect.get(0);
targetField.setAccessible(true);
targetField.set(obj, foreignText);
targetField.setAccessible(false);
}
} catch (IllegalAccessException e) {
e.printStackTrace();
throw new IllegalAccessException("无权设置指定反射字段的值");
}
return false;
}
private static Object getFieldValueByObject(Object object, String targetFieldName) throws Exception {
// 获取该对象的Class
Class objClass = object.getClass();
// 初始化返回值
Object result;
// 获取所有的属性数组
Field[] fields = objClass.getDeclaredFields();
for (Field field : fields) {
try {
if (field.getName().equals(targetFieldName)) {
field.setAccessible(true);
result = field.get(object);
return result; // 通过反射拿到该属性在此对象中的值(也可能是个对象)
}
} catch (SecurityException e) {
// 安全性异常
e.printStackTrace();
} catch (IllegalArgumentException e) {
// 非法参数
e.printStackTrace();
} catch (IllegalAccessException e) {
// 无访问权限
e.printStackTrace();
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy