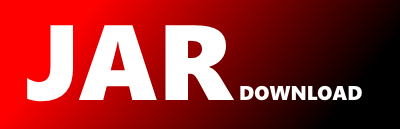
com.zys.mybatis.crud.Query Maven / Gradle / Ivy
package com.zys.mybatis.crud;
import com.zys.mybatis.constant.CharFinal;
import com.zys.mybatis.crud.base.Order;
import com.zys.mybatis.page.BasePage;
import com.zys.mybatis.utils.FieldUtils;
import com.zys.mybatis.utils.LambdaUtils;
import com.zys.mybatis.utils.QueryUtils;
/**
* @author zys
* @version 1.0
* @date 2020/10/22 11:54
*/
public class Query extends AbstractQuery> {
/**
* 条件 , 查询字段 构造器
* @param tClass 返回的实体类
* @param classes 查询字段的类
* @param parentField 是否查询父类字段
*/
public Query(Class tClass, Class> classes, Boolean parentField){
this.table = QueryUtils.getTableName(classes);
this.entity = tClass;
this.field(classes,parentField);
}
public Query(Class tClass){
this(tClass, false);
}
/**
* 条件 , 查询字段 构造器
* @param t 查询字段的类,返回的实体类
* @param parentField 是否查询父类字段
*/
public Query(T t, Boolean parentField){
Class aClass = (Class) t.getClass();
this.table = QueryUtils.getTableName(aClass);
this.entity = aClass;
this.field(aClass,parentField);
}
/**
* 条件 , 查询字段 构造器
* @param classes 查询字段的类,返回的实体类
* @param parentField 是否查询父类字段
*/
public Query(Class classes, Boolean parentField){
this.table = QueryUtils.getTableName(classes);
this.entity = classes;
this.field(classes,parentField);
}
/**
* 自定义字段查询
* 建议替换 Query(Class tClass, SFunction... columns)
*/
@Deprecated
public Query(Class tClass, Object field){
this.table = QueryUtils.getTableName(tClass);
this.entity = tClass;
this.field = field;
}
public Query(Class tClass, SFunction... columns){
this.table = QueryUtils.getTableName(tClass);
this.entity = tClass;
this.field = FieldUtils.getColumn(LambdaUtils.resolve(columns));
}
/**
* 自定义字段查询
* 建议替换 Query(Class tClass, Class tableClass, SFunction... columns)
* @param tClass 返回实体
* @param tableName 表名
* @param field 查询字段,多个用逗号分隔
*/
@Deprecated
public Query(Class tClass, String tableName, Object field){
this.table = tableName;
this.entity = tClass;
this.field = field;
}
/**
* 自定义字段查询
* @param tClass 返回实体
* @param tableClass 实体类class
* @param columns 查询字段
*/
public Query(Class tClass, Class> tableClass, SFunction... columns){
this.table = QueryUtils.getTableName(tableClass);
this.entity = tClass;
this.field = FieldUtils.getColumn(LambdaUtils.resolve(columns));
}
/**
* 查询字段
*/
private Object field;
private void field(Class> classes, Boolean parentField){
this.field = FieldUtils.querySqlField(classes, parentField, 3);
}
public Object getField(){
return this.field;
}
/**
* orderBy(StringUtils.isNoneBlank(sort),sort);
* 排序
* @param sort 排序字段 例:id desc,update_time
* @param condition 是否要排序
* @return
*/
public Query orderBy(boolean condition, String... sort){
if(condition){
this.orderBy = new OrderBy(CharFinal.ORDER_BY, sort);
}
return this;
}
public Query orderBy(Order... order){
assembleGroupBy(order);
return this;
}
public Query orderBy(boolean condition, Order... order){
if (condition) {
assembleGroupBy(order);
}
return this;
}
@SuppressWarnings("unchecked")
private void assembleGroupBy(Order[] order) {
String[] sort = new String[order.length];
for (int i = 0; i < order.length; i++) {
sort[i] = FieldUtils.getColumn(LambdaUtils.resolve(order[i].getColumn()))
+ CharFinal.EMPTY + order[i].getSort();
}
this.orderBy = new OrderBy(CharFinal.ORDER_BY, sort);
}
private OrderBy orderBy;
public OrderBy getOrderBy(){
return this.orderBy;
}
public static class OrderBy {
private OrderBy(String key, String[] orderBy){
this.key = key;
this.orderBy = orderBy;
}
private final String key;
private final String[] orderBy;
public String getKey(){
return this.key;
}
public String[] getOrderBy(){
return this.orderBy;
}
}
/**
* 分页
* @param index 从那个下标开始 可以为空
* @param pageSize 多少条
*/
public Query limit(Integer index, Integer pageSize){
this.page = new Page(index, pageSize);
return this;
}
/**
* 分页 limit pageSize
* @param pageSize 多少条
*/
public Query limit(Integer pageSize){
this.page = new Page(null, pageSize);
return this;
}
/**
* 分页
* @param basePage 分页对象
*/
public Query limit(BasePage basePage){
this.page = new Page(basePage.getIndex(), basePage.getPageSize());
return this;
}
private Page page;
public Page getPage(){
return this.page;
}
public Query setPage(Page page){
this.page = page;
return this;
}
public static class Page {
public Page (Integer index, Integer pageSize){
this.index = index;
this.pageSize = pageSize;
}
private final Integer index;
private final Integer pageSize;
public Integer getIndex(){
return this.index;
}
public Integer getPageSize(){
return this.pageSize;
}
}
/**
* 分组
* @param flag 是否要分组
* @param fields 分组字段集合
*/
public Query groupBy(boolean flag, String... fields){
if (flag) {
this.groupBy = new GroupBy(CharFinal.GROUP_BY, fields);
}
return this;
}
public Query groupBy(SFunction... fields){
this.groupBy = new GroupBy(CharFinal.GROUP_BY, new String[]{FieldUtils.getColumn(LambdaUtils.resolve(fields))});
return this;
}
public Query groupBy(boolean flag, SFunction... fields){
if (flag) {
this.groupBy = new GroupBy(CharFinal.GROUP_BY, new String[]{FieldUtils.getColumn(LambdaUtils.resolve(fields))});
}
return this;
}
private GroupBy groupBy;
public GroupBy getGroupBy() {
return this.groupBy;
}
public static class GroupBy {
private GroupBy(String key, String[] groupBy){
this.key = key;
this.groupBy = groupBy;
}
private final String key;
private final String[] groupBy;
public String getKey(){
return this.key;
}
public String[] getGroupBy(){
return this.groupBy;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy