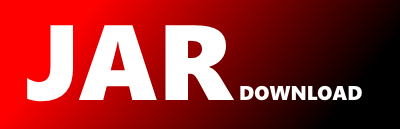
com.zys.mybatis.crud.Update Maven / Gradle / Ivy
package com.zys.mybatis.crud;
import com.zys.mybatis.annotation.*;
import com.zys.mybatis.dto.UpdateDto;
import com.zys.mybatis.utils.FieldUtils;
import com.zys.mybatis.utils.QueryUtils;
import com.zys.mybatis.utils.StringUtils;
import org.apache.ibatis.reflection.MetaObject;
import org.apache.ibatis.reflection.SystemMetaObject;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.HashMap;
import java.util.Map;
/**
* @author zys
* @version 1.0
* @date 2021/1/26 10:06
*/
public class Update extends AbstractQuery> {
/**
* 需要修改的map
* key 字段名
* value 修改的值
*/
private Map updateMap = new HashMap<>();
Update(){}
public Update(T entity){
this.table = QueryUtils.getTableName(entity.getClass());
this.update(entity);
}
public Map getUpdateMap(){
return updateMap;
}
private void update(T entity) {
Class> aClass = entity.getClass();
MetaObject metaObject = SystemMetaObject.forObject(entity);
while(!aClass.isAssignableFrom(Object.class)){
for (Field field : aClass.getDeclaredFields()) {
if (Modifier.isStatic(field.getModifiers())) {
//跳过静态字段
continue;
}
Id id = field.getDeclaredAnnotation(Id.class);
if (id != null) {
//跳过主键
continue;
}
Ignore ignore = field.getDeclaredAnnotation(Ignore.class);
if (ignore != null) {
continue;
}
Default aDefault = field.getDeclaredAnnotation(Default.class);
Object value = metaObject.getValue(field.getName());
if(aDefault != null){
boolean b = FillEnum.UPDATE.equals(aDefault.fill()) || FillEnum.INSERT_UPDATE.equals(aDefault.fill());
if (b) {
updateMap.put(new UpdateDto(FieldUtils.toUnderline(field.getName()), true), value == null ? QueryUtils.setDefault(metaObject.getGetterType(field.getName()), aDefault.value(),aDefault.defaultValue()) : value);
}
if (value != null) {
updateMap.put(new UpdateDto(FieldUtils.toUnderline(field.getName()), true), value);
}
continue;
}
Column column = field.getDeclaredAnnotation(Column.class);
if (column == null && value != null) {
notNullUpdate(FieldUtils.toUnderline(field.getName()),value,true);
} else if (column != null) {
String fieldName = StringUtils.isNotBlank(column.value()) ? column.value() : FieldUtils.toUnderline(field.getName());
boolean update = StringUtils.isNotBlank(column.update());
if (!column.notNull()) {
//可以为空
updateMap.put(new UpdateDto(fieldName, !update),update ? String.format(column.update(),fieldName,value) : value);
continue;
}
if(update){
if (value != null) {
notNullUpdate(fieldName,String.format(column.update(),fieldName,value),false);
}
continue;
}
if(value != null){
updateMap.put(new UpdateDto(fieldName),value);
}
}
}
aClass = aClass.getSuperclass();
}
}
/**
//先判断是否设置了默认,如果设置了默认值,再判断原始值是不是null是null就是使用默认值,否则使用设置的值.没有设置默认值,使用原始值
StringUtils.isNotBlank(column.defaultValue()) ? (
value == null?setDefault(metaObject.getGetterType(field.getName()),column.defaultValue()):value
) : value
*/
/**
* 设置不能为空字段
* @param fieldName 字段名称
* @param value 值
* @param isPoundSign true #{} ,false ${}
*/
public void notNullUpdate(String fieldName,Object value,boolean isPoundSign){
if (value instanceof CharSequence) {
CharSequence fieldValue = (CharSequence) value;
if (StringUtils.isNotBlank(fieldValue)) {
updateMap.put(new UpdateDto(fieldName,isPoundSign),fieldValue);
}
} else {
updateMap.put(new UpdateDto(fieldName,isPoundSign),value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy