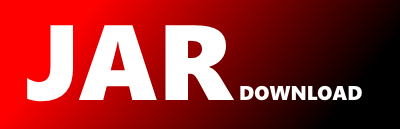
com.zys.mybatis.utils.QueryUtils Maven / Gradle / Ivy
package com.zys.mybatis.utils;
import com.zys.mybatis.annotation.*;
import com.zys.mybatis.condition.ConditionKey;
import com.zys.mybatis.constant.CharFinal;
import com.zys.mybatis.converter.DefaultValue;
import com.zys.mybatis.crud.Config;
import com.zys.mybatis.crud.Query;
import com.zys.mybatis.dao.base.BaseDao;
import com.zys.mybatis.dto.KeyValue;
import com.zys.mybatis.exception.MybatisZysException;
import com.zys.mybatis.page.BasePage;
import lombok.extern.slf4j.Slf4j;
import org.apache.ibatis.reflection.MetaObject;
import org.apache.ibatis.reflection.SystemMetaObject;
import org.springframework.util.CollectionUtils;
import org.springframework.util.ObjectUtils;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Modifier;
import java.util.*;
/**
* @author zys
* @version 1.0
* @date 2021/3/9 15:24
*/
@Slf4j
public class QueryUtils {
/**
* 设置其他字段值 list
* @param tClass 返回对象class
* @param data 数据
* @param 返回实体
*/
public static List setOtherField(Class> tClass, List data){
if (CollectionUtils.isEmpty(data)) {
return null;
}
EnableSetOtherField enableSetOtherField = tClass.getDeclaredAnnotation(EnableSetOtherField.class);
if (enableSetOtherField != null) {
String[] values = enableSetOtherField.value();
for (String value : values) {
try {
SetOtherField setOtherField = tClass.getDeclaredField(value).getDeclaredAnnotation(SetOtherField.class);
if (setOtherField != null) {
BaseDao baseDao = setOtherField.sourceDao().equals(BaseDao.class) ? SpringUtil.getPollBean(setOtherField.sourceDao()) : SpringUtil.getBean(setOtherField.sourceDao());
Object[] objects = data.stream().map(d -> {
MetaObject metaObject = SystemMetaObject.forObject(d);
return metaObject.getValue(setOtherField.targetRuleField());
}
).filter(f -> !ObjectUtils.isEmpty(f)).toArray();
if (ObjectUtils.isEmpty(objects)) {
return data;
}
QueryCondition[] queryConditions = setOtherField.queryConditions();
Map params = getParams(setOtherField.queryCondition(), objects);
if (ArrayUtils.isNotEmpty(queryConditions)) {
for (QueryCondition queryCondition : queryConditions) {
params.put(new ConditionKey(queryCondition.field(), queryCondition.condition()), queryCondition.value());
}
}
List> source = baseDao.queryAll(new Query<>(setOtherField.returnEntity(), String.join(",", setOtherField.queryField()))
.setParams(params));
Map map = new HashMap<>();
if (ArrayUtils.isNotEmpty(setOtherField.keys())) {
for (int i = 0; i < setOtherField.keys().length; i++) {
map.put(setOtherField.keys()[i],setOtherField.values()[i]);
}
} else {
String[] keys = Arrays.stream(setOtherField.queryField())
.filter(field -> !field.equals(setOtherField.sourceRuleField()))
.map(FieldUtils::toHump).toArray(String[]::new);
for (String key : keys) {
map.put(key, key);
}
}
ListUtils.copySpecifiedProperty(data, source, setOtherField.targetRuleField(), setOtherField.sourceRuleField(), map, setOtherField.relation());
}
} catch (NoSuchFieldException e) {
log.error("获取字段失败 {}",value);
}
}
}
return data;
}
private static Map getParams(String condition, Object... objects){
Map params = new LinkedHashMap<>();
params.put(new ConditionKey(condition, CharFinal.IN), objects);
return params;
}
public static Query.Page getPage(Object entity) {
if (entity instanceof BasePage) {
BasePage basePage = (BasePage) entity;
return new Query.Page(basePage.getPage(),basePage.getPageSize());
}
return null;
}
/**
* 获取表名称
* @param classes 指定表名称的class
* @return 表名
*/
public static String getTableName(Class> classes){
Table table = classes.getDeclaredAnnotation(Table.class);
if (table == null) {
throw new MybatisZysException("请指定表名");
}
return table.value();
}
/**
* 根据实体生成条件构造器
* @param entity
* @return
*/
public static Map getParam(Object entity){
Field[] fields = entity.getClass().getDeclaredFields();
MetaObject metaObject = SystemMetaObject.forObject(entity);
Map params = new HashMap<>();
for (Field field : fields) {
//忽略条件字段字段
if (field.getDeclaredAnnotation(Ignore.class) != null) continue;
//忽略静态字段
if (Modifier.isStatic(field.getModifiers())) continue;
String name = field.getName();
Object value = metaObject.getValue(name);
//忽略为空的字段
if (value == null) continue;
String condition = CharFinal.EQ;
Column column = field.getDeclaredAnnotation(Column.class);
if (column != null) {
if (column.notNull()) {
if (value instanceof CharSequence && "".equals(value)) {
continue;
}
}
name = column.value();
condition = column.condition();
String customValue = column.customValue();
if (StringUtils.isNotBlank(customValue)) {
//定制字段不为空
value = String.format(customValue,value);
}
}
params.put(new ConditionKey(column != null ? name : FieldUtils.toUnderline(name), condition), value);
}
return params;
}
/**
* 获取主键name
* @param entity 实体类
* @return 返回主键名称
*/
public static KeyValue getIdName(Object entity){
Field[] fields = entity.getClass().getDeclaredFields();
for (Field field : fields) {
Id id = field.getDeclaredAnnotation(Id.class);
if (id != null) {
MetaObject metaObject = SystemMetaObject.forObject(entity);
Object value = metaObject.getValue(FieldUtils.toHump(id.value()));
return new KeyValue(id.value(),value);
}
}
throw new MybatisZysException("请指定主键id");
}
/**
* 设置默认值
* @param fieldType
* @return
*/
public static Object setDefault(Class> fieldType,String value) {
try {
Constructor> constructor;
if (fieldType.isAssignableFrom(Character.class)) {
constructor = fieldType.getConstructor(char.class);
char[] chars = value.toCharArray();
return chars.length > 1 ? null:constructor.newInstance(chars[0]);
}
if (isBasicType(fieldType)){
constructor = fieldType.getConstructor(String.class);
return constructor.newInstance(value);
}
} catch (NoSuchMethodException | InstantiationException | IllegalAccessException | InvocationTargetException e) {
e.printStackTrace();
}
return null;
}
public static boolean isBasicType(Class> fieldType){
if (CharSequence.class.isAssignableFrom(fieldType)) {
return true;
}
if (fieldType.equals(Byte.class)) {
return true;
}
if (fieldType.equals(Short.class)) {
return true;
}
if (fieldType.equals(Integer.class)) {
return true;
}
if (fieldType.equals(Long.class)) {
return true;
}
if (fieldType.equals(Double.class)) {
return true;
}
if (fieldType.equals(Float.class)) {
return true;
}
if (fieldType.equals(Boolean.class)) {
return true;
}
return fieldType.equals(Character.class);
}
public static Object setDefault(Class> fieldType,String value,Class extends DefaultValue> d){
Object o = QueryUtils.setDefault(fieldType, value);
if (o == null) {
DefaultValue> defaultValue = Config.getDefaultValue(d);
return defaultValue.setDefault(fieldType, value);
}
return o;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy