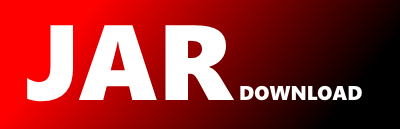
org.zodiac.actuate.bootstrap.registry.AppRegistryEndpoint Maven / Gradle / Ivy
package org.zodiac.actuate.bootstrap.registry;
import org.springframework.boot.actuate.endpoint.annotation.Endpoint;
import org.springframework.boot.actuate.endpoint.annotation.ReadOperation;
import org.springframework.boot.actuate.endpoint.annotation.WriteOperation;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.util.Assert;
import org.zodiac.core.bootstrap.registry.AppRegistration;
import org.zodiac.core.bootstrap.registry.AppRegistry;
@SuppressWarnings("unchecked")
@Endpoint(id = AppRegistryEndpoint.ENDPOINT_NAME)
public class AppRegistryEndpoint {
public static final String ENDPOINT_NAME = "app-registry";
private final AppRegistry appServiceRegistry;
private AppRegistration appRegistration;
public AppRegistryEndpoint(AppRegistry> serviceRegistry) {
this.appServiceRegistry = serviceRegistry;
}
public void setRegistration(AppRegistration registration) {
this.appRegistration = registration;
}
@WriteOperation
public ResponseEntity> setStatus(String status) {
Assert.notNull(status, "Status may not by null.");
if (this.appRegistration == null) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("No application service registration found.");
}
this.appServiceRegistry.setStatus(this.appRegistration, status);
return ResponseEntity.ok().build();
}
@ReadOperation
public ResponseEntity
© 2015 - 2024 Weber Informatics LLC | Privacy Policy