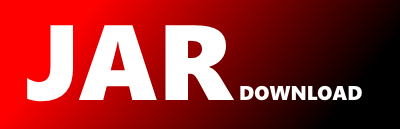
org.zodiac.autoconfigure.feign.dubbo.context.DubboRpcContextHolderAutoConfiguration Maven / Gradle / Ivy
package org.zodiac.autoconfigure.feign.dubbo.context;
import java.lang.reflect.Method;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
import org.springframework.boot.SpringBootConfiguration;
import org.springframework.boot.autoconfigure.condition.ConditionalOnClass;
import org.springframework.context.annotation.Bean;
import org.zodiac.autoconfigure.feign.condition.ConditionalOnDubboEnabled;
import org.zodiac.autoconfigure.feign.condition.ConditionalOnFeignDubboEnabled;
import org.zodiac.commons.util.Classes;
import org.zodiac.commons.util.Reflections;
@SpringBootConfiguration
@ConditionalOnDubboEnabled
@ConditionalOnFeignDubboEnabled
@ConditionalOnClass(value = {org.apache.dubbo.rpc.RpcContext.class, org.apache.dubbo.spring.boot.autoconfigure.DubboAutoConfiguration.class, feign.Client.class, org.zodiac.feign.core.FeignConsumerClientProvider.class, org.zodiac.feign.dubbo.FeignDubbo.class})
public class DubboRpcContextHolderAutoConfiguration {
public DubboRpcContextHolderAutoConfiguration() {
super();
}
@Bean
protected org.zodiac.feign.core.context.FeignRpcContextHolder feignDubboRpcContextHolder() {
return new FeignDubboRpcContextHolder();
}
static class FeignDubboRpcContextHolder extends org.zodiac.feign.core.context.FeignRpcContextHolder {
/*Compatibility FIXED: apache-dubbo-2.7.x => getContext() and getServerContext()*/
/*dubbo-2.7.x:org.apache.dubbo.rpc.RpcContext*/
private static final @Nullable Class> APACHE_DUBBO27_RPCCONTEXT_CLASS = Classes.resolveClassNameNullable(
"org.apache.dubbo.rpc.RpcContext");
/*dubbo-2.6.x:getContext(),dubbo-2.7.x:getServerContext()*/
private static final @Nullable Method APACHE_DUBBO27_GETSERVERCONTEXT_METHOD = Reflections.findMethodNullable(
APACHE_DUBBO27_RPCCONTEXT_CLASS, "getServerContext");
/*dubbo-2.6.x:getContext(),dubbo-2.7.x:getServerContext()*/
private static final @Nullable Method APACHE_DUBBO27_REMOVSERVERCONTEXT_METHOD = Reflections.findMethodNullable(
APACHE_DUBBO27_RPCCONTEXT_CLASS, "removeServerContext");
@Override
public String getAttachment(String key) {
throw new IllegalStateException();
}
@Override
public Map getAttachments() {
throw new IllegalStateException();
}
@Override
public void setAttachment(String key, String value) {
throw new IllegalStateException();
}
@Override
public void removeAttachment(String key) {
throw new IllegalStateException();
}
@Override
public void clearAttachments() {
throw new IllegalStateException();
}
@Override
protected org.zodiac.feign.core.context.FeignRpcContextHolder getContext0() {
return DEFAULT_DUBBO_RPC_CONTEXT;
}
@Override
protected org.zodiac.feign.core.context.FeignRpcContextHolder getServerContext0() {
return DEFAULT_DUBBO_SERVER_RPC_CONTEXT;
}
@Override
protected void removeContext0() {
org.apache.dubbo.rpc.RpcContext.removeContext();
}
@Override
protected void removeServerContext0() {
/*For compatibility dubbo-2.6/dubbo-2.7 .*/
if (Objects.nonNull(APACHE_DUBBO27_REMOVSERVERCONTEXT_METHOD)) {
try {
APACHE_DUBBO27_REMOVSERVERCONTEXT_METHOD.invoke(null);
} catch (Exception e) {
throw new IllegalStateException(e);
}
} else {
/*Fallback .*/
org.apache.dubbo.rpc.RpcContext.removeContext();
}
}
private static final org.zodiac.feign.core.context.FeignRpcContextHolder DEFAULT_DUBBO_SERVER_RPC_CONTEXT = new org.zodiac.feign.core.context.FeignRpcContextHolder() {
@Override
public String getAttachment(String key) {
if (Objects.nonNull(APACHE_DUBBO27_GETSERVERCONTEXT_METHOD)) {
try {
org.apache.dubbo.rpc.RpcContext rpcContext = (org.apache.dubbo.rpc.RpcContext) APACHE_DUBBO27_GETSERVERCONTEXT_METHOD.invoke(null);
return rpcContext.getAttachment(key);
} catch (Exception e) {
throw new IllegalStateException(e);
}
}
/*Fallback .*/
return org.apache.dubbo.rpc.RpcContext.getContext().getAttachment(key);
}
@Override
public Map getAttachments() {
if (Objects.nonNull(APACHE_DUBBO27_GETSERVERCONTEXT_METHOD)) {
try {
org.apache.dubbo.rpc.RpcContext rpcContext = (org.apache.dubbo.rpc.RpcContext) APACHE_DUBBO27_GETSERVERCONTEXT_METHOD.invoke(null);
return rpcContext.getAttachments();
} catch (Exception e) {
throw new IllegalStateException(e);
}
}
/*Fallback .*/
return org.apache.dubbo.rpc.RpcContext.getContext().getAttachments();
}
@Override
public void setAttachment(String key, String value) {
if (Objects.nonNull(APACHE_DUBBO27_GETSERVERCONTEXT_METHOD)) {
try {
org.apache.dubbo.rpc.RpcContext rpcContext = (org.apache.dubbo.rpc.RpcContext) APACHE_DUBBO27_GETSERVERCONTEXT_METHOD.invoke(null);
rpcContext.setAttachment(key, value);
} catch (Exception e) {
throw new IllegalStateException(e);
}
}
}
@Override
public void removeAttachment(String key) {
if (Objects.nonNull(APACHE_DUBBO27_GETSERVERCONTEXT_METHOD)) {
try {
org.apache.dubbo.rpc.RpcContext rpcContext = (org.apache.dubbo.rpc.RpcContext) APACHE_DUBBO27_GETSERVERCONTEXT_METHOD.invoke(null);
rpcContext.removeAttachment(key);
} catch (Exception e) {
throw new IllegalStateException(e);
}
} else {
/*Fallback .*/
org.apache.dubbo.rpc.RpcContext.getContext().removeAttachment(key);
}
}
@Override
public void clearAttachments() {
if (Objects.nonNull(APACHE_DUBBO27_GETSERVERCONTEXT_METHOD)) {
try {
org.apache.dubbo.rpc.RpcContext rpcContext = (org.apache.dubbo.rpc.RpcContext) APACHE_DUBBO27_GETSERVERCONTEXT_METHOD.invoke(null);
rpcContext.clearAttachments();
} catch (Exception e) {
throw new IllegalStateException(e);
}
} else {
/*Fallback .*/
org.apache.dubbo.rpc.RpcContext.getContext().clearAttachments();
}
}
@Override
protected org.zodiac.feign.core.context.FeignRpcContextHolder getContext0() {
return DEFAULT_DUBBO_RPC_CONTEXT;
}
@Override
protected org.zodiac.feign.core.context.FeignRpcContextHolder getServerContext0() {
return this;
}
@Override
protected void removeContext0() {
throw new IllegalStateException();
}
@Override
protected void removeServerContext0() {
throw new IllegalStateException();
}
};
private static final org.zodiac.feign.core.context.FeignRpcContextHolder DEFAULT_DUBBO_RPC_CONTEXT = new org.zodiac.feign.core.context.FeignRpcContextHolder() {
@Override
public String getAttachment(String key) {
return org.apache.dubbo.rpc.RpcContext.getContext().getAttachment(key);
}
@Override
public Map getAttachments() {
return org.apache.dubbo.rpc.RpcContext.getContext().getAttachments();
}
@Override
public void setAttachment(String key, String value) {
org.apache.dubbo.rpc.RpcContext.getContext().setAttachment(key, value);
}
@Override
public void removeAttachment(String key) {
org.apache.dubbo.rpc.RpcContext.getContext().removeAttachment(key);
}
@Override
public void clearAttachments() {
org.apache.dubbo.rpc.RpcContext.getContext().clearAttachments();
}
@Override
protected org.zodiac.feign.core.context.FeignRpcContextHolder getContext0() {
return this;
}
@Override
protected org.zodiac.feign.core.context.FeignRpcContextHolder getServerContext0() {
return DEFAULT_DUBBO_SERVER_RPC_CONTEXT;
}
@Override
protected void removeContext0() {
throw new IllegalStateException();
}
@Override
protected void removeServerContext0() {
throw new IllegalStateException();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy