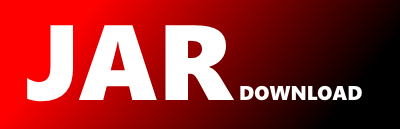
org.zodiac.eureka.client.EurekaAutoAppRegistration Maven / Gradle / Ivy
package org.zodiac.eureka.client;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicInteger;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.boot.web.context.WebServerInitializedEvent;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationEvent;
import org.springframework.context.SmartLifecycle;
import org.springframework.context.event.ContextClosedEvent;
import org.springframework.context.event.SmartApplicationListener;
import org.springframework.core.Ordered;
import org.zodiac.core.bootstrap.registry.AutoAppRegistration;
import org.zodiac.core.event.discovery.AppInstanceRegisteredEvent;
public class EurekaAutoAppRegistration implements AutoAppRegistration, SmartLifecycle, Ordered, SmartApplicationListener {
private static final Logger log = LoggerFactory.getLogger(EurekaAutoAppRegistration.class);
private AtomicBoolean running = new AtomicBoolean(false);
private int order = 0;
private AtomicInteger port = new AtomicInteger(0);
private ApplicationContext context;
private EurekaAppServiceRegistry serviceRegistry;
private EurekaAppRegistration registration;
public EurekaAutoAppRegistration(ApplicationContext context,
EurekaAppServiceRegistry serviceRegistry, EurekaAppRegistration registration) {
this.context = context;
this.serviceRegistry = serviceRegistry;
this.registration = registration;
}
@Override
public void start() {
/*only initialize if nonSecurePort is greater than 0 and it isn't already running because of containerPortInitializer below*/
if (!this.running.get() && this.registration.getNonSecurePort() > 0) {
this.serviceRegistry.register(this.registration);
this.context.publishEvent(new AppInstanceRegisteredEvent<>(this,
this.registration.getInstanceConfig()));
this.running.set(true);
}
}
@Override
public void stop() {
this.serviceRegistry.deregister(this.registration);
this.running.set(false);
}
@Override
public boolean isRunning() {
return this.running.get();
}
@Override
public void onApplicationEvent(ApplicationEvent event) {
if (event instanceof WebServerInitializedEvent) {
onApplicationEvent((WebServerInitializedEvent) event);
} else if (event instanceof ContextClosedEvent) {
onApplicationEvent((ContextClosedEvent) event);
}
}
@Override
public boolean supportsEventType(Class extends ApplicationEvent> eventType) {
return WebServerInitializedEvent.class.isAssignableFrom(eventType)
|| ContextClosedEvent.class.isAssignableFrom(eventType);
}
@Override
public int getOrder() {
return order;
}
public void onApplicationEvent(WebServerInitializedEvent event) {
// TODO: take SSL into account
String contextName = event.getApplicationContext().getServerNamespace();
if (contextName == null || !contextName.equals("management")) {
int localPort = event.getWebServer().getPort();
if (this.port.get() == 0) {
log.info("Updating port to " + localPort);
this.port.compareAndSet(0, localPort);
start();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy