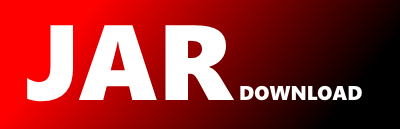
org.zodiac.flowable.engine.model.entity.TenantFlowModelEntity Maven / Gradle / Ivy
package org.zodiac.flowable.engine.model.entity;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Date;
import java.util.Objects;
/**
* 流程模型。
*
*/
public class TenantFlowModelEntity implements Serializable {
private static final long serialVersionUID = 1090642891755912121L;
public static final int MODEL_TYPE_BPMN = 0;
public static final int MODEL_TYPE_FORM = 2;
public static final int MODEL_TYPE_APP = 3;
public static final int MODEL_TYPE_DECISION_TABLE = 4;
public static final int MODEL_TYPE_CMMN = 5;
private String id;
private String name;
private String modelKey;
private String description;
private Date created;
private Date lastUpdated;
private String createdBy;
private String lastUpdatedBy;
private Integer version;
private String modelEditorJson;
private String modelComment;
private Integer modelType;
private String tenantId;
private byte[] thumbnail;
public TenantFlowModelEntity() {
super();
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getModelKey() {
return modelKey;
}
public void setModelKey(String modelKey) {
this.modelKey = modelKey;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Date getCreated() {
return created;
}
public void setCreated(Date created) {
this.created = created;
}
public Date getLastUpdated() {
return lastUpdated;
}
public void setLastUpdated(Date lastUpdated) {
this.lastUpdated = lastUpdated;
}
public String getCreatedBy() {
return createdBy;
}
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
public String getLastUpdatedBy() {
return lastUpdatedBy;
}
public void setLastUpdatedBy(String lastUpdatedBy) {
this.lastUpdatedBy = lastUpdatedBy;
}
public Integer getVersion() {
return version;
}
public void setVersion(Integer version) {
this.version = version;
}
public String getModelEditorJson() {
return modelEditorJson;
}
public void setModelEditorJson(String modelEditorJson) {
this.modelEditorJson = modelEditorJson;
}
public String getModelComment() {
return modelComment;
}
public void setModelComment(String modelComment) {
this.modelComment = modelComment;
}
public Integer getModelType() {
return modelType;
}
public void setModelType(Integer modelType) {
this.modelType = modelType;
}
public String getTenantId() {
return tenantId;
}
public void setTenantId(String tenantId) {
this.tenantId = tenantId;
}
public byte[] getThumbnail() {
return thumbnail;
}
public void setThumbnail(byte[] thumbnail) {
this.thumbnail = thumbnail;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + Arrays.hashCode(thumbnail);
result = prime * result + Objects.hash(created, createdBy, description, id, lastUpdated, lastUpdatedBy,
modelComment, modelEditorJson, modelKey, modelType, name, tenantId, version);
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
TenantFlowModelEntity other = (TenantFlowModelEntity)obj;
return Objects.equals(created, other.created) && Objects.equals(createdBy, other.createdBy)
&& Objects.equals(description, other.description) && Objects.equals(id, other.id)
&& Objects.equals(lastUpdated, other.lastUpdated) && Objects.equals(lastUpdatedBy, other.lastUpdatedBy)
&& Objects.equals(modelComment, other.modelComment)
&& Objects.equals(modelEditorJson, other.modelEditorJson) && Objects.equals(modelKey, other.modelKey)
&& Objects.equals(modelType, other.modelType) && Objects.equals(name, other.name)
&& Objects.equals(tenantId, other.tenantId) && Arrays.equals(thumbnail, other.thumbnail)
&& Objects.equals(version, other.version);
}
@Override
public String toString() {
return "TenantFlowModelEntity [id=" + id + ", name=" + name + ", modelKey=" + modelKey + ", description=" + description
+ ", created=" + created + ", lastUpdated=" + lastUpdated + ", createdBy=" + createdBy + ", lastUpdatedBy="
+ lastUpdatedBy + ", version=" + version + ", modelEditorJson=" + modelEditorJson + ", modelComment="
+ modelComment + ", modelType=" + modelType + ", tenantId=" + tenantId + ", thumbnail="
+ Arrays.toString(thumbnail) + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy