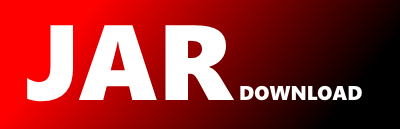
org.zodiac.flowable.engine.model.FlowExecution Maven / Gradle / Ivy
package org.zodiac.flowable.engine.model;
import java.io.Serializable;
import java.util.Date;
import java.util.Objects;
/**
* 运行实体类。
*
*/
public class FlowExecution implements Serializable {
private static final long serialVersionUID = -532257571855324459L;
private String id;
private String name;
private String startUserId;
private String startUser;
private Date startTime;
private String taskDefinitionId;
private String taskDefinitionKey;
private String category;
private String categoryName;
private String processInstanceId;
private String processDefinitionId;
private String processDefinitionKey;
private String activityId;
private int suspensionState;
private String executionId;
public FlowExecution() {
super();
}
public String getId() {
return id;
}
public FlowExecution setId(String id) {
this.id = id;
return this;
}
public String getName() {
return name;
}
public FlowExecution setName(String name) {
this.name = name;
return this;
}
public String getStartUserId() {
return startUserId;
}
public FlowExecution setStartUserId(String startUserId) {
this.startUserId = startUserId;
return this;
}
public String getStartUser() {
return startUser;
}
public FlowExecution setStartUser(String startUser) {
this.startUser = startUser;
return this;
}
public Date getStartTime() {
return startTime;
}
public FlowExecution setStartTime(Date startTime) {
this.startTime = startTime;
return this;
}
public String getTaskDefinitionId() {
return taskDefinitionId;
}
public FlowExecution setTaskDefinitionId(String taskDefinitionId) {
this.taskDefinitionId = taskDefinitionId;
return this;
}
public String getTaskDefinitionKey() {
return taskDefinitionKey;
}
public FlowExecution setTaskDefinitionKey(String taskDefinitionKey) {
this.taskDefinitionKey = taskDefinitionKey;
return this;
}
public String getCategory() {
return category;
}
public FlowExecution setCategory(String category) {
this.category = category;
return this;
}
public String getCategoryName() {
return categoryName;
}
public FlowExecution setCategoryName(String categoryName) {
this.categoryName = categoryName;
return this;
}
public String getProcessInstanceId() {
return processInstanceId;
}
public FlowExecution setProcessInstanceId(String processInstanceId) {
this.processInstanceId = processInstanceId;
return this;
}
public String getProcessDefinitionId() {
return processDefinitionId;
}
public FlowExecution setProcessDefinitionId(String processDefinitionId) {
this.processDefinitionId = processDefinitionId;
return this;
}
public String getProcessDefinitionKey() {
return processDefinitionKey;
}
public FlowExecution setProcessDefinitionKey(String processDefinitionKey) {
this.processDefinitionKey = processDefinitionKey;
return this;
}
public String getActivityId() {
return activityId;
}
public FlowExecution setActivityId(String activityId) {
this.activityId = activityId;
return this;
}
public int getSuspensionState() {
return suspensionState;
}
public FlowExecution setSuspensionState(int suspensionState) {
this.suspensionState = suspensionState;
return this;
}
public String getExecutionId() {
return executionId;
}
public FlowExecution setExecutionId(String executionId) {
this.executionId = executionId;
return this;
}
@Override
public int hashCode() {
return Objects.hash(activityId, category, categoryName, executionId, id, name, processDefinitionId,
processDefinitionKey, processInstanceId, startTime, startUser, startUserId, suspensionState,
taskDefinitionId, taskDefinitionKey);
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
FlowExecution other = (FlowExecution)obj;
return Objects.equals(activityId, other.activityId) && Objects.equals(category, other.category)
&& Objects.equals(categoryName, other.categoryName) && Objects.equals(executionId, other.executionId)
&& Objects.equals(id, other.id) && Objects.equals(name, other.name)
&& Objects.equals(processDefinitionId, other.processDefinitionId)
&& Objects.equals(processDefinitionKey, other.processDefinitionKey)
&& Objects.equals(processInstanceId, other.processInstanceId) && Objects.equals(startTime, other.startTime)
&& Objects.equals(startUser, other.startUser) && Objects.equals(startUserId, other.startUserId)
&& suspensionState == other.suspensionState && Objects.equals(taskDefinitionId, other.taskDefinitionId)
&& Objects.equals(taskDefinitionKey, other.taskDefinitionKey);
}
@Override
public String toString() {
return "FlowExecution [id=" + id + ", name=" + name + ", startUserId=" + startUserId + ", startUser="
+ startUser + ", startTime=" + startTime + ", taskDefinitionId=" + taskDefinitionId + ", taskDefinitionKey="
+ taskDefinitionKey + ", category=" + category + ", categoryName=" + categoryName + ", processInstanceId="
+ processInstanceId + ", processDefinitionId=" + processDefinitionId + ", processDefinitionKey="
+ processDefinitionKey + ", activityId=" + activityId + ", suspensionState=" + suspensionState
+ ", executionId=" + executionId + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy