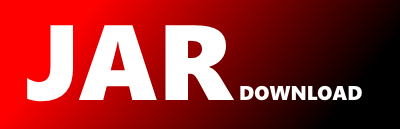
org.zodiac.flowable.engine.model.TenantFlowProcessModel Maven / Gradle / Ivy
package org.zodiac.flowable.engine.model;
import org.flowable.engine.impl.persistence.entity.ProcessDefinitionEntity;
import org.zodiac.flowable.engine.util.ProcessFlowCacheUtil;
import org.zodiac.flowable.engine.util.FlowCategoryNameSupplier;
import java.io.Serializable;
import java.util.Date;
import java.util.Objects;
/**
* TenantFlowProcessModel。
*
*/
public class TenantFlowProcessModel implements Serializable {
private static final long serialVersionUID = -1753967621589101750L;
private String id;
private String tenantId;
private String name;
private String key;
private String category;
private String categoryName;
private Integer version;
private String deploymentId;
private String resourceName;
private String diagramResourceName;
private Integer suspensionState;
private Date deploymentTime;
// public TenantFlowProcessModel(ProcessDefinitionEntityImpl entity) {
// this.id = entity.getId();
// this.tenantId = entity.getTenantId();
// this.name = entity.getName();
// this.key = entity.getKey();
// this.category = entity.getCategory();
// this.categoryName = ProcessFlowCacheUtil.getCategoryName(entity.getCategory());
// this.version = entity.getVersion();
// this.deploymentId = entity.getDeploymentId();
// this.resourceName = entity.getResourceName();
// this.diagramResourceName = entity.getDiagramResourceName();
// this.suspensionState = entity.getSuspensionState();
// }
@Deprecated
public TenantFlowProcessModel(ProcessDefinitionEntity entity) {
this(entity, FlowCategoryNameSupplier.EMPTY_SUPPLIER);
}
public TenantFlowProcessModel(ProcessDefinitionEntity entity, FlowCategoryNameSupplier flowCategoryNameSupplier) {
this.id = entity.getId();
this.tenantId = entity.getTenantId();
this.name = entity.getName();
this.key = entity.getKey();
this.category = entity.getCategory();
this.categoryName = ProcessFlowCacheUtil.getCategoryName(entity.getCategory(), flowCategoryNameSupplier);
this.version = entity.getVersion();
this.deploymentId = entity.getDeploymentId();
this.resourceName = entity.getResourceName();
this.diagramResourceName = entity.getDiagramResourceName();
this.suspensionState = entity.getSuspensionState();
}
public String getId() {
return id;
}
public TenantFlowProcessModel setId(String id) {
this.id = id;
return this;
}
public String getTenantId() {
return tenantId;
}
public TenantFlowProcessModel setTenantId(String tenantId) {
this.tenantId = tenantId;
return this;
}
public String getName() {
return name;
}
public TenantFlowProcessModel setName(String name) {
this.name = name;
return this;
}
public String getKey() {
return key;
}
public TenantFlowProcessModel setKey(String key) {
this.key = key;
return this;
}
public String getCategory() {
return category;
}
public TenantFlowProcessModel setCategory(String category) {
this.category = category;
return this;
}
public String getCategoryName() {
return categoryName;
}
public TenantFlowProcessModel setCategoryName(String categoryName) {
this.categoryName = categoryName;
return this;
}
public Integer getVersion() {
return version;
}
public TenantFlowProcessModel setVersion(Integer version) {
this.version = version;
return this;
}
public String getDeploymentId() {
return deploymentId;
}
public TenantFlowProcessModel setDeploymentId(String deploymentId) {
this.deploymentId = deploymentId;
return this;
}
public String getResourceName() {
return resourceName;
}
public TenantFlowProcessModel setResourceName(String resourceName) {
this.resourceName = resourceName;
return this;
}
public String getDiagramResourceName() {
return diagramResourceName;
}
public TenantFlowProcessModel setDiagramResourceName(String diagramResourceName) {
this.diagramResourceName = diagramResourceName;
return this;
}
public Integer getSuspensionState() {
return suspensionState;
}
public TenantFlowProcessModel setSuspensionState(Integer suspensionState) {
this.suspensionState = suspensionState;
return this;
}
public Date getDeploymentTime() {
return deploymentTime;
}
public TenantFlowProcessModel setDeploymentTime(Date deploymentTime) {
this.deploymentTime = deploymentTime;
return this;
}
@Override
public int hashCode() {
return Objects.hash(category, categoryName, deploymentId, deploymentTime, diagramResourceName, id, key, name,
resourceName, suspensionState, tenantId, version);
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
TenantFlowProcessModel other = (TenantFlowProcessModel)obj;
return Objects.equals(category, other.category) && Objects.equals(categoryName, other.categoryName)
&& Objects.equals(deploymentId, other.deploymentId) && Objects.equals(deploymentTime, other.deploymentTime)
&& Objects.equals(diagramResourceName, other.diagramResourceName) && Objects.equals(id, other.id)
&& Objects.equals(key, other.key) && Objects.equals(name, other.name)
&& Objects.equals(resourceName, other.resourceName)
&& Objects.equals(suspensionState, other.suspensionState) && Objects.equals(tenantId, other.tenantId)
&& Objects.equals(version, other.version);
}
@Override
public String toString() {
return "TenantFlowProcessModel [id=" + id + ", tenantId=" + tenantId + ", name=" + name + ", key=" + key + ", category="
+ category + ", categoryName=" + categoryName + ", version=" + version + ", deploymentId=" + deploymentId
+ ", resourceName=" + resourceName + ", diagramResourceName=" + diagramResourceName + ", suspensionState="
+ suspensionState + ", deploymentTime=" + deploymentTime + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy