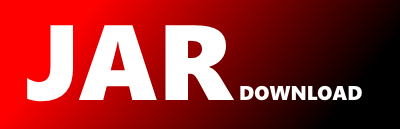
org.zodic.kubernetes.confcenter.ConfigMapPropertySourceLocator Maven / Gradle / Ivy
The newest version!
package org.zodic.kubernetes.confcenter;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import io.fabric8.kubernetes.client.KubernetesClient;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.annotation.Order;
import org.springframework.core.env.CompositePropertySource;
import org.springframework.core.env.ConfigurableEnvironment;
import org.springframework.core.env.Environment;
import org.springframework.core.env.MapPropertySource;
import org.springframework.core.env.PropertySource;
import org.zodiac.core.bootstrap.config.AppPropertySourceLocator;
import org.zodiac.core.constants.AppOrderConstants;
import org.zodiac.sdk.toolkit.util.collection.CollUtil;
import org.zodic.kubernetes.confcenter.ConfigMapConfigInfo.NormalizedSource;
@Order(value = AppOrderConstants.AppPropertySourceLocatorOrder.KUBERNETES)
public class ConfigMapPropertySourceLocator implements AppPropertySourceLocator {
private static final Logger LOG = LoggerFactory.getLogger(ConfigMapPropertySourceLocator.class);
private final KubernetesClient client;
private final ConfigMapConfigInfo configMapConfigInfo;
public ConfigMapPropertySourceLocator(KubernetesClient client, ConfigMapConfigInfo configMapConfigInfo) {
this.client = client;
this.configMapConfigInfo = configMapConfigInfo;
}
@Override
public PropertySource> locatePropertySource(Environment environment) {
if (environment instanceof ConfigurableEnvironment) {
ConfigurableEnvironment env = (ConfigurableEnvironment)environment;
List sources = this.configMapConfigInfo.determineSources();
CompositePropertySource composite = new CompositePropertySource("composite-configmap");
if (this.configMapConfigInfo.isEnableApi()) {
sources.forEach(s -> composite.addFirstPropertySource(getMapPropertySourceForSingleConfigMap(env, s)));
}
addPropertySourcesFromPaths(environment, composite);
return composite;
}
return null;
}
@Override
public int getPriority() {
return AppOrderConstants.AppPropertySourceLocatorOrder.KUBERNETES;
}
private MapPropertySource getMapPropertySourceForSingleConfigMap(ConfigurableEnvironment environment,
NormalizedSource normalizedSource) {
String configurationTarget = this.configMapConfigInfo.getConfigurationTarget();
return new ConfigMapPropertySource(this.client,
ConfigUtils.getApplicationName(environment, normalizedSource.getName(), configurationTarget),
ConfigUtils.getApplicationNamespace(environment, this.client, normalizedSource.getNamespace(), configurationTarget), environment);
}
private void addPropertySourcesFromPaths(Environment environment, CompositePropertySource composite) {
this.configMapConfigInfo.getPaths().stream().map(Paths::get).peek(p -> {
if (!Files.exists(p)) {
LOG.warn(
"Configured input path: {} will be ignored because it does not exist on the file system.");
}
}).filter(Files::exists).peek(p -> {
if (!Files.isRegularFile(p)) {
LOG.warn("Configured input path: {} will be ignored because it is not a regular file.", p);
}
}).filter(Files::isRegularFile).forEach(p -> {
try {
String content = new String(Files.readAllBytes(p)).trim();
String filename = p.getFileName().toString().toLowerCase();
if (filename.endsWith(".properties")) {
addPropertySourceIfNeeded(c -> PropertySourceUtil.PROPERTIES_TO_MAP.apply(PropertySourceUtil.KEY_VALUE_TO_PROPERTIES.apply(c)), content,
filename, composite);
} else if (filename.endsWith(".yml") || filename.endsWith(".yaml")) {
addPropertySourceIfNeeded(c -> PropertySourceUtil.PROPERTIES_TO_MAP.apply(PropertySourceUtil.yamlParserGenerator(environment).apply(c)),
content, filename, composite);
}
} catch (IOException e) {
LOG.warn("Error reading input file", e);
}
});
}
private void addPropertySourceIfNeeded(Function> contentToMapFunction, String content,
String name, CompositePropertySource composite) {
Map map = CollUtil.map();
map.putAll(contentToMapFunction.apply(content));
if (map.isEmpty()) {
LOG.warn("Property source: " + name + "will be ignored because no configMapConfigInfo could be found");
} else {
composite.addFirstPropertySource(new MapPropertySource(name, map));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy