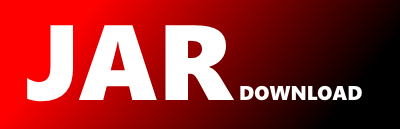
org.zodiac.mybatisplus.binding.RelationsBinder Maven / Gradle / Ivy
package org.zodiac.mybatisplus.binding;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.context.request.RequestContextHolder;
import org.zodiac.commons.support.SpringContextHolder;
import org.zodiac.commons.util.BeanUtil;
import org.zodiac.commons.util.Colls;
import org.zodiac.mybatisplus.binding.binder.parallel.ParallelBindingManager;
import org.zodiac.mybatisplus.binding.helper.DeepRelationsBinder;
import org.zodiac.mybatisplus.binding.parser.BindAnnotationGroup;
import org.zodiac.mybatisplus.binding.parser.FieldAnnotation;
import org.zodiac.mybatisplus.binding.parser.ParserCache;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
/**
* 关联关系绑定管理器。
*
*/
public class RelationsBinder {
private static final Logger log = LoggerFactory.getLogger(RelationsBinder.class);
private RelationsBinder() {
super();
}
/**
* 自动转换和绑定单个VO中的注解关联(禁止循环调用,多个对象请调用convertAndBind(voList, voClass))。
*
* @param 实体类型
* @param VO类型
* @param entity 源实体对象
* @param voClass 需要转换的VO class
* @return VO对象
*/
public static VO convertAndBind(E entity, Class voClass) {
/*转换为VO列表*/
VO vo = BeanUtil.convert(entity, voClass);
/*自动绑定关联对象*/
bind(vo);
return vo;
}
/**
* 自动转换和绑定多个VO中的注解关联。
*
* @param 实体类型
* @param VO类型
* @param entityList 需要转换的VO list
* @param voClass VO class
* @return VO对象列表
*/
public static List convertAndBind(List entityList, Class voClass) {
/*转换为VO列表*/
List voList = BeanUtil.convertList(entityList, voClass);
/*自动绑定关联对象*/
bind(voList);
return voList;
}
/**
* 自动绑定单个VO的关联对象(禁止循环调用,多个对象请调用bind(voList))。
*
* @param VO类型
* @param vo 需要注解绑定的对象
*/
public static void bind(VO vo) {
bind(Collections.singletonList(vo));
}
/**
* 自动绑定多个VO集合的关联对象。
*
* @param VO类型
* @param voList 需要注解绑定的对象集合
*/
public static void bind(List voList) {
bind(voList, true);
}
/**
* 自动绑定多个VO集合的关联对象。
*
* @param VO类型
* @param voList 需要注解绑定的对象集合
* @param enableDeepBind 是否深度绑定
* @param parallelBindingManager 并行绑定管理器对象
*/
public static void bind(List voList, boolean enableDeepBind,
ParallelBindingManager parallelBindingManager) {
if (Colls.emptyColl(voList) || null == parallelBindingManager) {
return;
}
/*获取VO类。*/
Class> voClass = voList.get(0).getClass();
BindAnnotationGroup bindAnnotationGroup = ParserCache.getBindAnnotationGroup(voClass);
if (bindAnnotationGroup.isEmpty()) {
return;
}
RequestContextHolder.setRequestAttributes(RequestContextHolder.getRequestAttributes(), true);
/*不可能出现的错误,但是编译器需要。*/
assert parallelBindingManager != null;
List> binderFutures = new ArrayList<>();
/*绑定Field字段名*/
Map> bindFieldGroupMap = bindAnnotationGroup.getBindFieldGroupMap();
if (bindFieldGroupMap != null) {
for (Map.Entry> entry : bindFieldGroupMap.entrySet()) {
CompletableFuture bindFieldFuture =
parallelBindingManager.doBindingField(voList, entry.getValue());
binderFutures.add(bindFieldFuture);
}
}
/*绑定数据字典。*/
List dictAnnoList = bindAnnotationGroup.getBindDictAnnotations();
if (dictAnnoList != null) {
if (bindAnnotationGroup.isRequireSequential()) {
CompletableFuture.allOf(binderFutures.toArray(new CompletableFuture[0])).join();
}
for (FieldAnnotation annotation : dictAnnoList) {
CompletableFuture bindDictFuture = parallelBindingManager.doBindingDict(voList, annotation);
binderFutures.add(bindDictFuture);
}
}
/*绑定Entity实体。*/
List entityAnnoList = bindAnnotationGroup.getBindEntityAnnotations();
if (entityAnnoList != null) {
for (FieldAnnotation anno : entityAnnoList) {
/*绑定关联对象entity。*/
CompletableFuture bindEntFuture = parallelBindingManager.doBindingEntity(voList, anno);
binderFutures.add(bindEntFuture);
}
}
/*绑定Entity实体List。*/
List entitiesAnnoList = bindAnnotationGroup.getBindEntityListAnnotations();
if (entitiesAnnoList != null) {
for (FieldAnnotation anno : entitiesAnnoList) {
/*绑定关联对象entity。*/
CompletableFuture bindEntFuture = parallelBindingManager.doBindingEntityList(voList, anno);
binderFutures.add(bindEntFuture);
}
}
/*绑定Entity field List*/
Map> bindFieldListGroupMap = bindAnnotationGroup.getBindFieldListGroupMap();
if (bindFieldListGroupMap != null) {
/*解析条件并且执行绑定。*/
for (Map.Entry> entry : bindFieldListGroupMap.entrySet()) {
CompletableFuture bindFieldFuture =
parallelBindingManager.doBindingFieldList(voList, entry.getValue());
binderFutures.add(bindFieldFuture);
}
}
/*I绑定count子项计数。*/
List countAnnoList = bindAnnotationGroup.getBindCountAnnotations();
if (countAnnoList != null) {
for (FieldAnnotation anno : countAnnoList) {
/*绑定关联对象count计数。*/
CompletableFuture bindCountFuture = parallelBindingManager.doBindingCount(voList, anno);
binderFutures.add(bindCountFuture);
}
}
/*执行绑定*/
CompletableFuture.allOf(binderFutures.toArray(new CompletableFuture[0])).join();
/*深度绑定*/
if (enableDeepBind) {
List deepBindEntityAnnoList = bindAnnotationGroup.getDeepBindEntityAnnotations();
List deepBindEntitiesAnnoList = bindAnnotationGroup.getDeepBindEntityListAnnotations();
if (deepBindEntityAnnoList != null || deepBindEntitiesAnnoList != null) {
if (Colls.notEmptyColl(deepBindEntityAnnoList)) {
FieldAnnotation firstAnnotation = deepBindEntityAnnoList.get(0);
log.debug("执行深度绑定: {}({}) for field {}",
firstAnnotation.getAnnotation().annotationType().getSimpleName(),
firstAnnotation.getFieldClass().getSimpleName(), firstAnnotation.getFieldName());
}
if (deepBindEntitiesAnnoList != null) {
FieldAnnotation firstAnnotation = deepBindEntitiesAnnoList.get(0);
log.debug("执行深度绑定: {}({}) for field {}",
firstAnnotation.getAnnotation().annotationType().getSimpleName(),
firstAnnotation.getFieldClass().getSimpleName(), firstAnnotation.getFieldName());
}
DeepRelationsBinder.deepBind(voList, deepBindEntityAnnoList, deepBindEntitiesAnnoList);
}
}
}
/**
* 自动绑定多个VO集合的关联对象。
*
* @param VO类型
* @param voList 需要注解绑定的对象集合
* @param enableDeepBind 是否深度绑定
*/
public static void bind(List voList, boolean enableDeepBind) {
ParallelBindingManager parallelBindingManager = SpringContextHolder.getBean(ParallelBindingManager.class);
bind(voList, enableDeepBind, parallelBindingManager);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy