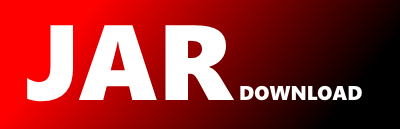
org.zodiac.mybatisplus.binding.binder.parallel.ParallelBindingManager Maven / Gradle / Ivy
package org.zodiac.mybatisplus.binding.binder.parallel;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.scheduling.annotation.Async;
import org.zodiac.commons.util.lang.Strings;
import org.zodiac.core.exception.IllegalUsageException;
import org.zodiac.mybatisplus.base.DictionaryServiceExtProvider;
import org.zodiac.mybatisplus.binding.annotation.*;
import org.zodiac.mybatisplus.binding.binder.*;
import org.zodiac.mybatisplus.binding.parser.ConditionManager;
import org.zodiac.mybatisplus.binding.parser.FieldAnnotation;
import org.zodiac.sdk.toolkit.util.lang.StrUtil;
import java.util.List;
import java.util.concurrent.CompletableFuture;
/**
* 并行绑定Manager。
*
*/
//@Component
public class ParallelBindingManager {
private static final Logger log = LoggerFactory.getLogger(ParallelBindingManager.class);
//@Autowired(required = false)
private DictionaryServiceExtProvider dictionaryServiceExtProvider;
public ParallelBindingManager(DictionaryServiceExtProvider dictionaryServiceExtProvider) {
this.dictionaryServiceExtProvider = dictionaryServiceExtProvider;
}
/**
* 绑定字典。
*
* @param voList VO对象列表
* @param fieldAnno 注解的包装对象
* @return {@link CompletableFuture}并行处理对象
*/
@Async
public CompletableFuture doBindingDict(List voList, FieldAnnotation fieldAnno) {
if (dictionaryServiceExtProvider != null) {
BindDict annotation = (BindDict)fieldAnno.getAnnotation();
String dictValueField = annotation.field();
if (StrUtil.isBlank(dictValueField)) {
dictValueField = Strings.replace(fieldAnno.getFieldName(), "Label", "");
log.debug("BindDict未指定field,将默认取值为: {}", dictValueField);
}
/*字典绑定接口化。*/
dictionaryServiceExtProvider.bindItemLabel(voList, fieldAnno.getFieldName(), dictValueField,
annotation.type());
} else {
throw new IllegalUsageException("BindDictService未实现,无法使用BindDict注解!");
}
return CompletableFuture.completedFuture(true);
}
/***
* 绑定Field。
*
* @param voList VO对象列表
* @param fieldAnnotations 注解的包装对象列表
* @return {@link CompletableFuture}并行处理对象
*/
@Async
public CompletableFuture doBindingField(List voList, List fieldAnnotations) {
BindField bindAnnotation = (BindField)fieldAnnotations.get(0).getAnnotation();
FieldBinder binder = new FieldBinder(bindAnnotation, voList);
for (FieldAnnotation anno : fieldAnnotations) {
BindField bindField = (BindField)anno.getAnnotation();
binder.link(bindField.field(), anno.getFieldName());
}
/*解析条件并且执行绑定*/
return doBinding(binder, bindAnnotation.condition());
}
/***
* 绑定FieldList。
*
* @param voList VO对象列表
* @param fieldAnnotations 注解的包装对象列表
* @return {@link CompletableFuture}并行处理对象
*/
@Async
public CompletableFuture doBindingFieldList(List voList, List fieldAnnotations) {
BindFieldList bindAnnotation = (BindFieldList)fieldAnnotations.get(0).getAnnotation();
FieldListBinder binder = new FieldListBinder(bindAnnotation, voList);
for (FieldAnnotation anno : fieldAnnotations) {
BindFieldList bindField = (BindFieldList)anno.getAnnotation();
binder.link(bindField.field(), anno.getFieldName());
}
/*解析条件并且执行绑定。*/
return doBinding(binder, bindAnnotation.condition());
}
/***
* 绑定Entity。
*
* @param voList VO对象列表
* @param fieldAnnotation 注解的包装对象
* @return {@link CompletableFuture}并行处理对象
*/
@Async
public CompletableFuture doBindingEntity(List voList, FieldAnnotation fieldAnnotation) {
BindEntity annotation = (BindEntity)fieldAnnotation.getAnnotation();
/*绑定关联对象entity。*/
EntityBinder binder = new EntityBinder(annotation, voList);
/*构建binder*/
binder.set(fieldAnnotation.getFieldName(), fieldAnnotation.getFieldClass());
/*解析条件并且执行绑定。*/
return doBinding(binder, annotation.condition());
}
/***
* 绑定EntityList
*
* @param voList VO对象列表
* @param fieldAnnotation 注解的包装对象
* @return {@link CompletableFuture}并行处理对象
*/
@Async
public CompletableFuture doBindingEntityList(List voList, FieldAnnotation fieldAnnotation) {
BindEntityList annotation = (BindEntityList)fieldAnnotation.getAnnotation();
/*构建binder。*/
EntityListBinder binder = new EntityListBinder(annotation, voList);
binder.set(fieldAnnotation.getFieldName(), fieldAnnotation.getFieldClass());
/*解析条件并且执行绑定。*/
return doBinding(binder, annotation.condition());
}
/***
* 绑定count计数。
*
* @param voList VO对象列表
* @param fieldAnnotation 注解的包装对象
* @return {@link CompletableFuture}并行处理对象
*/
@Async
public CompletableFuture doBindingCount(List voList, FieldAnnotation fieldAnnotation) {
BindCount annotation = (BindCount)fieldAnnotation.getAnnotation();
/*绑定关联对象entity。*/
CountBinder binder = new CountBinder(annotation, voList);
/*构建binder。*/
binder.set(fieldAnnotation.getFieldName(), fieldAnnotation.getFieldClass());
/*解析条件并且执行绑定。*/
return doBinding(binder, annotation.condition());
}
/**
* 绑定表关联数据。
*
* @param binder 绑定器
* @param condition 条件
* @return {@link CompletableFuture}并行处理对象
*/
private CompletableFuture doBinding(BaseBinder binder, String condition) {
ConditionManager.parseConditions(condition, binder);
binder.bind();
return CompletableFuture.completedFuture(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy