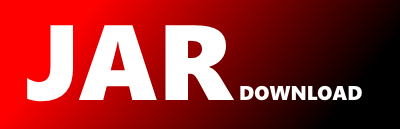
org.zodiac.mybatisplus.binding.helper.ResultAssembler Maven / Gradle / Ivy
package org.zodiac.mybatisplus.binding.helper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.BeanWrapper;
import org.zodiac.commons.support.SpringContextHolder;
import org.zodiac.commons.util.BeanUtil;
import org.zodiac.commons.util.Colls;
import org.zodiac.commons.util.lang.Strings;
import org.zodiac.sdk.toolkit.util.lang.StrUtil;
import java.util.*;
/**
* 绑定关联数据组装器。
*
*/
public class ResultAssembler {
private static Logger log = LoggerFactory.getLogger(ResultAssembler.class);
/***
* 从对象集合提取某个属性值到list中。
*
* @param setterFieldName set字段名
* @param fromList 源列表
* @param getterFields get字段列表
* @param valueMatchMap 配置值的map
* @param splitBy 分隔符
* @param 类型
*/
public static void bindPropValue(String setterFieldName, List fromList, String[] getterFields,
Map valueMatchMap, String splitBy) {
if (Colls.emptyColl(fromList) || Colls.emptyMap(valueMatchMap)) {
return;
}
try {
for (E object : fromList) {
List matchKeys = null;
BeanWrapper beanWrapper = SpringContextHolder.getBeanWrapper(object);
for (int i = 0; i < getterFields.length; i++) {
Object fieldValueObj = BeanUtil.getProperty(object, getterFields[i]);
if (fieldValueObj == null) {
continue;
}
if (fieldValueObj instanceof Collection) {
if (matchKeys == null) {
matchKeys = new ArrayList();
}
matchKeys.addAll((Collection)fieldValueObj);
} else {
if (matchKeys == null) {
matchKeys = new ArrayList(getterFields.length);
}
matchKeys.add(StrUtil.cleanNonConsts(StrUtil.stringValueOf(fieldValueObj)));
}
}
if (matchKeys == null) {
continue;
}
/*查找匹配Key。*/
String matchKey = Strings.join(matchKeys);
if (valueMatchMap.containsKey(matchKey)) {
/*赋值。*/
beanWrapper.setPropertyValue(setterFieldName, valueMatchMap.get(matchKey));
} else {
if (matchKeys.size() == 1 && StrUtil.isNotBlank(splitBy) && getterFields.length == 1
&& matchKey.contains(splitBy)) {
matchKeys = StrUtil.splitToList(matchKey, splitBy);
}
List matchedValues = new ArrayList(matchKeys.size());
for (Object key : matchKeys) {
Object value = valueMatchMap.get(StrUtil.stringValueOf(key));
if (value != null) {
if (value instanceof Collection) {
Collection valueList = (Collection)value;
for (Object obj : valueList) {
if (!matchedValues.contains(obj)) {
matchedValues.add(obj);
}
}
} else {
if (!matchedValues.contains(value)) {
matchedValues.add(value);
}
}
}
}
/*赋值。*/
beanWrapper.setPropertyValue(setterFieldName, matchedValues);
}
}
} catch (Exception e) {
log.warn("设置属性值异常, setterFieldName=" + setterFieldName, e);
}
}
/***
* 从对象集合提取某个属性值到list中。
*
* @param setterFieldName set字段名
* @param fromList 源列表
* @param trunkObjColMapping 对象列map
* @param valueMatchMap 配置值的map
* @param col2FieldMapping 列到字段的映射map
* @param 类型
*/
public static void bindEntityPropValue(String setterFieldName, List fromList,
Map trunkObjColMapping, Map valueMatchMap, Map col2FieldMapping) {
if (Colls.emptyColl(fromList) || Colls.emptyMap(valueMatchMap)) {
return;
}
StringBuilder sb = new StringBuilder();
try {
for (E object : fromList) {
boolean appendComma = false;
sb.setLength(0);
for (Map.Entry entry : trunkObjColMapping.entrySet()) {
/*转换为字段名。*/
String getterField = col2FieldMapping.get(entry.getKey());
if (getterField == null) {
getterField = StrUtil.toLowerCaseCamel(entry.getKey());
}
String fieldValue = BeanUtil.getStringProperty(object, getterField);
if (appendComma) {
sb.append(Strings.COMMA);
}
sb.append(fieldValue);
if (appendComma == false) {
appendComma = true;
}
}
/*查找匹配Key。*/
String matchKey = sb.toString();
if (valueMatchMap.containsKey(matchKey)) {
/*赋值。*/
BeanUtil.setProperty(object, setterFieldName, valueMatchMap.get(matchKey));
}
}
sb.setLength(0);
} catch (Exception e) {
log.warn("设置属性值异常, setterFieldName=" + setterFieldName, e);
}
}
public static void bindFieldListPropValue(List fromList, String[] getterFields,
Map valueMatchMap, List annoObjSetterPropNameList, List refGetterFieldNameList,
String splitBy) {
if (Colls.emptyColl(fromList) || Colls.emptyMap(valueMatchMap)) {
return;
}
try {
for (E object : fromList) {
List matchKeys = null;
for (int i = 0; i < getterFields.length; i++) {
Object fieldValueObj = BeanUtil.getProperty(object, getterFields[i]);
if (fieldValueObj == null) {
continue;
}
if (fieldValueObj instanceof Collection) {
if (matchKeys == null) {
matchKeys = new ArrayList();
}
matchKeys.addAll((Collection)fieldValueObj);
} else {
if (matchKeys == null) {
matchKeys = new ArrayList(getterFields.length);
}
matchKeys.add(StrUtil.cleanNonConsts(StrUtil.stringValueOf(fieldValueObj)));
}
}
/*无有效值,跳过。*/
if (matchKeys == null) {
continue;
}
/*查找匹配Key。*/
String matchKey = Strings.join(matchKeys);
List entityList = valueMatchMap.get(matchKey);
if (entityList == null) {
if (matchKeys.size() == 1 && StrUtil.isNotBlank(splitBy) && matchKey.contains(splitBy)) {
matchKeys = StrUtil.splitToList(matchKey, splitBy);
}
List matchedValues = new ArrayList(matchKeys.size());
for (Object key : matchKeys) {
Object value = valueMatchMap.get(StrUtil.stringValueOf(key));
if (value != null) {
if (value instanceof Collection) {
Collection valueList = (Collection)value;
for (Object obj : valueList) {
if (!matchedValues.contains(obj)) {
matchedValues.add(obj);
}
}
} else {
if (!matchedValues.contains(value)) {
matchedValues.add(value);
}
}
}
}
if (matchedValues != null) {
entityList = matchedValues;
}
}
/*赋值。*/
BeanWrapper beanWrapper = SpringContextHolder.getBeanWrapper(object);
for (int i = 0; i < annoObjSetterPropNameList.size(); i++) {
List valObjList = BeanUtil.collectToList(entityList, refGetterFieldNameList.get(i));
beanWrapper.setPropertyValue(annoObjSetterPropNameList.get(i), valObjList);
}
}
} catch (Exception e) {
log.warn("设置属性值异常", e);
}
}
/**
* 合并为1-1的map结果。
*
* @param resultSetMapList 结果集map列表
* @param trunkObjColMapping 主干对象列映射map
* @param branchObjColMapping 分支对象列映射map
* @param 类型
* @return 结果
*/
public static Map convertToOneToOneResult(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy