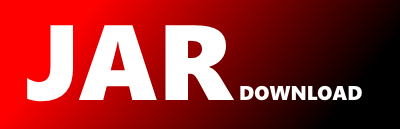
org.zodiac.mybatisplus.binding.parser.BindAnnotationGroup Maven / Gradle / Ivy
package org.zodiac.mybatisplus.binding.parser;
import java.lang.annotation.Annotation;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.zodiac.mybatisplus.binding.annotation.*;
import org.zodiac.sdk.toolkit.util.lang.ObjUtil;
/**
* VO绑定注解的归类分组,用于缓存解析后的结果。
*
*/
public class BindAnnotationGroup {
private Logger log = LoggerFactory.getLogger(getClass());
/**
* Dictionary注解
*/
private List bindDictAnnotations;
/**
* BindField分组Map
*/
private Map> bindFieldGroupMap;
/**
* 实体关联注解
*/
private List bindEntityAnnotations;
/**
* 实体集合关联注解
*/
private List bindEntityListAnnotations;
/**
* BindFieldList分组Map
*/
private Map> bindFieldListGroupMap;
/**
* count计数关联注解
*/
private List bindCountAnnotations;
/**
* 深度绑定实体
*/
private List deepBindEntityAnnotations;
/**
* 深度绑定实体
*/
private List deepBindEntityListAnnotations;
/**
* 需要序列
*/
private boolean requireSequential = false;
public BindAnnotationGroup() {
super();
}
/**
* 添加注解。
*
* @param fieldName 字段名
* @param fieldClass 字段类型
* @param annotation 注解对象
*/
public void addBindAnnotation(String fieldName, Class> fieldClass, Annotation annotation) {
FieldAnnotation fieldAnnotation = new FieldAnnotation(fieldName, fieldClass, annotation);
if (annotation instanceof BindDict) {
if (bindDictAnnotations == null) {
bindDictAnnotations = new ArrayList<>(4);
}
bindDictAnnotations.add(fieldAnnotation);
if (!requireSequential && bindFieldGroupMap != null) {
/*是否存在重复的字段。任意一个符合条件,即可结束遍历。*/
requireSequential = bindFieldGroupMap.values().stream().anyMatch(list -> list.stream().anyMatch(
item -> item.getFieldName().equals(fieldName) && item.getFieldClass().equals(fieldClass)));
}
return;
}
String key = null;
try {
if (annotation instanceof BindField) {
BindField bindField = (BindField)annotation;
key = bindField.entity().getName() + ":" + bindField.condition();
} else if (annotation instanceof BindFieldList) {
BindFieldList bindField = (BindFieldList)annotation;
key = bindField.entity().getName() + ":" + bindField.condition() + ":" + bindField.orderBy();
} else if (annotation instanceof BindCount) {
BindCount bindCount = (BindCount)annotation;
key = bindCount.entity().getName() + ":" + bindCount.condition();
} else if (annotation instanceof BindEntity) {
BindEntity bindEntity = (BindEntity)annotation;
key = bindEntity.entity().getName();
} else if (annotation instanceof BindEntityList) {
BindEntityList bindEntity = (BindEntityList)annotation;
key = bindEntity.entity().getName();
}
} catch (Exception e) {
log.warn("获取绑定信息异常", e);
return;
}
if (annotation instanceof BindField) {
if (bindFieldGroupMap == null) {
bindFieldGroupMap = new HashMap<>(4);
}
List list = bindFieldGroupMap.computeIfAbsent(key, k -> new ArrayList<>(4));
list.add(fieldAnnotation);
if (!requireSequential && bindDictAnnotations != null) {
/*是否存在重复的字段*/
requireSequential = bindDictAnnotations.stream()
.anyMatch(item -> item.getFieldName().equals(fieldName) && item.getFieldClass().equals(fieldClass));
}
} else if (annotation instanceof BindEntity) {
if (bindEntityAnnotations == null) {
bindEntityAnnotations = new ArrayList<>(4);
}
bindEntityAnnotations.add(fieldAnnotation);
if (((BindEntity)annotation).deepBind()) {
if (deepBindEntityAnnotations == null) {
deepBindEntityAnnotations = new ArrayList<>(4);
}
deepBindEntityAnnotations.add(fieldAnnotation);
}
} else if (annotation instanceof BindEntityList) {
if (bindEntityListAnnotations == null) {
bindEntityListAnnotations = new ArrayList<>(4);
}
bindEntityListAnnotations.add(fieldAnnotation);
if (((BindEntityList)annotation).deepBind()) {
if (deepBindEntityListAnnotations == null) {
deepBindEntityListAnnotations = new ArrayList<>(4);
}
deepBindEntityListAnnotations.add(fieldAnnotation);
}
} else if (annotation instanceof BindFieldList) {
/*多个字段,合并查询,以减少SQL数*/
if (bindFieldListGroupMap == null) {
bindFieldListGroupMap = new HashMap<>(4);
}
List list = bindFieldListGroupMap.computeIfAbsent(key, k -> new ArrayList<>(4));
list.add(fieldAnnotation);
} else if (annotation instanceof BindCount) {
if (bindCountAnnotations == null) {
bindCountAnnotations = new ArrayList<>(4);
}
bindCountAnnotations.add(fieldAnnotation);
}
}
public List getBindDictAnnotations() {
return bindDictAnnotations;
}
public List getBindEntityAnnotations() {
return bindEntityAnnotations;
}
public List getBindEntityListAnnotations() {
return bindEntityListAnnotations;
}
public Map> getBindFieldGroupMap() {
return bindFieldGroupMap;
}
public Map> getBindFieldListGroupMap() {
return bindFieldListGroupMap;
}
public List getBindCountAnnotations() {
return bindCountAnnotations;
}
public List getDeepBindEntityAnnotations() {
return deepBindEntityAnnotations;
}
public List getDeepBindEntityListAnnotations() {
return deepBindEntityListAnnotations;
}
public boolean isRequireSequential() {
return requireSequential;
}
public boolean isEmpty() {
return ObjUtil.isEmptyObjects(bindDictAnnotations, bindFieldGroupMap, bindEntityAnnotations, bindEntityListAnnotations,
bindFieldListGroupMap, bindCountAnnotations);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy