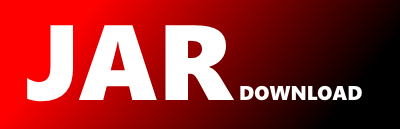
org.zodiac.mybatisplus.binding.parser.EntityInfoCache Maven / Gradle / Ivy
package org.zodiac.mybatisplus.binding.parser;
import com.baomidou.mybatisplus.annotation.TableName;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.baomidou.mybatisplus.extension.service.IService;
import org.springframework.core.annotation.AnnotationUtils;
import org.zodiac.commons.support.SpringContextHolder;
import org.zodiac.mybatisplus.binding.cache.BindingCacheManager;
import org.zodiac.sdk.toolkit.util.lang.StrUtil;
import java.io.Serializable;
/**
* Entity相关信息缓存。
*
*/
public class EntityInfoCache implements Serializable {
private static final long serialVersionUID = 1799419175720868754L;
private String tableName;
private String entityClassName;
/**
* 属性信息
*/
private PropInfo propInfo;
/**
* 表对应的entity类
*/
private Class> entityClass;
/**
* service 实例名
*/
private String serviceBeanName;
/**
* 表对应的mapper类
*/
private Class extends BaseMapper> mapperClass;
public EntityInfoCache(Class> entityClass, String serviceBeanName) {
this.entityClass = entityClass;
this.entityClassName = entityClass.getName();
/*初始化字段-列名的映射。*/
this.propInfo = BindingCacheManager.getPropInfoByClass(entityClass);
/*初始化tableName。*/
TableName tableNameAnno = AnnotationUtils.findAnnotation(entityClass, TableName.class);
if (tableNameAnno != null && StrUtil.isNotBlank(tableNameAnno.value())) {
this.tableName = tableNameAnno.value();
} else {
this.tableName = StrUtil.toSnakeCase(entityClass.getSimpleName());
}
/*设置当前service实例名。*/
this.serviceBeanName = serviceBeanName;
}
public String getTableName() {
return tableName;
}
public void setTableName(String tableName) {
this.tableName = tableName;
}
public String getEntityClassName() {
return entityClassName;
}
public void setEntityClassName(String entityClassName) {
this.entityClassName = entityClassName;
}
public PropInfo getPropInfo() {
return propInfo;
}
public void setPropInfo(PropInfo propInfo) {
this.propInfo = propInfo;
}
public Class> getEntityClass() {
return entityClass;
}
public void setEntityClass(Class> entityClass) {
this.entityClass = entityClass;
}
public String getServiceBeanName() {
return serviceBeanName;
}
public void setServiceBeanName(String serviceBeanName) {
this.serviceBeanName = serviceBeanName;
}
public Class extends BaseMapper> getMapperClass() {
return mapperClass;
}
public void setMapperClass(Class extends BaseMapper> mapperClass) {
this.mapperClass = mapperClass;
}
public void setService(String serviceBeanName) {
this.serviceBeanName = serviceBeanName;
}
public IService getService() {
return this.serviceBeanName == null ? null
: (IService)SpringContextHolder.getBean(this.serviceBeanName);
}
public void setBaseMapper(Class extends BaseMapper> mapper) {
this.mapperClass = mapper;
}
public BaseMapper getBaseMapper() {
return mapperClass == null ? getService().getBaseMapper() : SpringContextHolder.getBean(this.mapperClass);
}
/**
* 根据列名获取字段。
*
* @param columnName 列名
* @return 结果
*/
public String getFieldByColumn(String columnName) {
if (this.propInfo == null) {
return null;
}
return this.propInfo.getFieldByColumn(columnName);
}
/**
* 根据列名获取字段。
*
* @param fieldName 字段名
* @return 结果
*/
public String getColumnByField(String fieldName) {
if (this.propInfo == null) {
return null;
}
return this.propInfo.getColumnByField(fieldName);
}
/**
* 获取ID列。
*
* @return 结果
*/
public String getIdColumn() {
if (this.propInfo == null) {
return null;
}
return this.propInfo.getIdColumn();
}
/**
* 获取逻辑删除标记列。
*
* @return 结果
*/
public String getDeletedColumn() {
if (this.propInfo == null) {
return null;
}
return this.propInfo.getDeletedColumn();
}
/**
* 是否包含某字段。
*
* @param column 列名
* @return 结果
*/
public boolean containsColumn(String column) {
return this.propInfo.getColumns().contains(column);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy