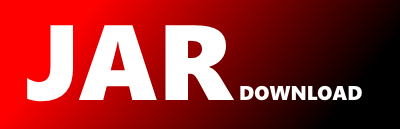
org.zodiac.netty.marshallers.NettyContentMarshallers Maven / Gradle / Ivy
package org.zodiac.netty.marshallers;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.zodiac.sdk.toolkit.marshallers.ContentMarshallers;
import org.zodiac.sdk.toolkit.marshallers.Marshaller;
import org.zodiac.sdk.mime.MimeType;
import io.netty.buffer.ByteBuf;
import io.netty.util.CharsetUtil;
import java.awt.image.RenderedImage;
import java.io.InputStream;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.util.List;
import java.util.Map;
/**
* Registry of interpreters that can read and write objects into Netty ByteBuf
* instances.
*
*/
public final class NettyContentMarshallers extends ContentMarshallers {
public NettyContentMarshallers() {
add(ByteBuf.class, new ByteBufMarshaller())
.add(ByteBuffer.class, new ByteBufferMarshaller())
.add(byte[].class, new ByteArrayInterpreter());
}
/**
* Adds a built-in marshaller for byte arrays.
*
* @return this
*/
public NettyContentMarshallers withByteArrays() {
return add(byte[].class, new ByteArrayInterpreter());
}
/**
* Adds a built-in marshaller for Strings. The charset may be included in
* the hints, either as a Charset or MediaType instance.
*
* @return this
*/
public NettyContentMarshallers withStrings() {
return add(String.class, new StringMarshaller());
}
/**
* Adds a built-in marshaller for BufferedImage / RenderedImage. A MIME type
* or format name (recognized by ImageIO) will be used if passed as a hint;
* in the event of an unsupported format, jpeg is used.
*
* @return this
*/
public NettyContentMarshallers withImages() {
return add(RenderedImage.class, new ImageStreamInterpreter());
}
/**
* Adds a built-in marshaller for InputStreams.
*
* @return this
*/
public NettyContentMarshallers withInputStreams() {
return add(InputStream.class, new InputStreamInterpreter());
}
/**
* Adds a built-in marshaller for CharSequences. The charset may be included
* in the hints, either as a Charset or MediaType instance.
*
* @return this
*/
public NettyContentMarshallers withCharSequences() {
return add(CharSequence.class, new CharSequenceInterperter());
}
/**
* Adds a built-in marshaller for Maps to JSON using Jackson.
*
* @param mapper A Jackson ObjectMapper for JSON conversion
* @return this
*/
@SuppressWarnings("unchecked")
public NettyContentMarshallers withJsonMaps(ObjectMapper mapper) {
return add(Map.class, (Marshaller) new JsonMapMarshaller(mapper));
}
/**
* Adds a built-in marshaller for Lists to JSON using Jackson.
*
* @param mapper A Jackson ObjectMapper for JSON conversion
* @return this
*/
@SuppressWarnings("unchecked")
public NettyContentMarshallers withJsonLists(ObjectMapper mapper) {
return add(List.class, (Marshaller) new JsonListMarshaller(mapper));
}
/**
* Adds a built-in marshaller for Object to JSON using Jackson - read
* objects will use whatever default type the ObjectMapper is configured to
* use (for example, a double[] might be returned as a double[] or a
* List<Double>.
*
* @param mapper A Jackson ObjectMapper for JSON conversion
* @return this
*/
@SuppressWarnings("unchecked")
public NettyContentMarshallers withJsonObjects(ObjectMapper mapper) {
return add(Object.class, (Marshaller) new JsonObjectMarshaller(mapper));
}
/**
* Get a default content marshaller which includes all of the built in
* marshallers.
*
* @param mapper A Jackson ObjectMapper for JSON conversion
* @return this
*/
public static NettyContentMarshallers getDefault(ObjectMapper mapper) {
return new NettyContentMarshallers().withByteArrays().withImages().withInputStreams().withJsonMaps(mapper).withStrings()
.withJsonLists(mapper).withCharSequences().withJsonObjects(mapper);
}
static T findHint(Class type, Object[] hints, T defaultValue) {
for (Object h : hints) {
if (type.isInstance(h)) {
return type.cast(h);
}
}
return defaultValue;
}
static Charset findCharset(Object[] hints) {
MimeType type = findHint(MimeType.class, hints, null);
Charset charset = type == null ? null : type.charset().isPresent() ? type.charset().get() : null;
if (charset == null) {
charset = findHint(Charset.class, hints, CharsetUtil.UTF_8);
}
return charset;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy