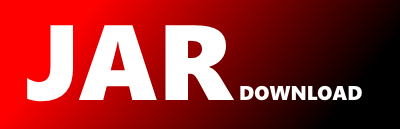
org.zodiac.reactor.event.AsyncEventHooks Maven / Gradle / Ivy
The newest version!
package org.zodiac.reactor.event;
import reactor.core.publisher.Mono;
import java.util.LinkedList;
public class AsyncEventHooks {
// private static final FastThreadLocal> HOOKS = new FastThreadLocal>() {
// @Override
// protected LinkedList initialValue() {
// return new LinkedList<>();
// }
// };
private final ThreadLocal> hooks = new ThreadLocal>() {
@Override
protected LinkedList initialValue() {
return new LinkedList<>();
}
};
protected AsyncEventHooks() {
super();
}
protected LinkedList getHooksList() {
return hooks.get();
}
protected LinkedList getHooksListIfExists() {
return getHooksList();
}
protected AutoUnbindable bindHook(AsyncEventHook hook) {
LinkedList list = getHooksList();
list.add(hook);
return () -> list.removeLastOccurrence(hook);
}
protected Mono> hookToFirst(AsyncEvent event, Mono> publisher) {
LinkedList hooksList = getHooksListIfExists();
if (hooksList == null) {
return publisher;
}
for (AsyncEventHook asyncEventHook : hooksList) {
publisher = asyncEventHook.hookFirst(event, publisher);
}
return publisher;
}
protected Mono> hookToAsync(AsyncEvent event, Mono> publisher) {
LinkedList hooksList = getHooksListIfExists();
if (hooksList == null) {
return publisher;
}
for (AsyncEventHook asyncEventHook : hooksList) {
publisher = asyncEventHook.hookAsync(event, publisher);
}
return publisher;
}
public static AutoUnbindable bind(AsyncEventHook hook) {
return getInstance().bindHook(hook);
}
static Mono> hookFirst(AsyncEvent event, Mono> publisher) {
return getInstance().hookToFirst(event, publisher);
}
static Mono> hookAsync(AsyncEvent event, Mono> publisher) {
return getInstance().hookToAsync(event, publisher);
}
private static AsyncEventHooks getInstance() {
return AsyncEventHooksHolder.INSTANCE;
}
public interface AutoUnbindable extends AutoCloseable {
@Override
void close();
}
public interface AsyncEventHook {
default Mono> hookAsync(AsyncEvent event, Mono> publisher) {
return publisher;
}
default Mono> hookFirst(AsyncEvent event, Mono> publisher) {
return publisher;
}
}
private static class AsyncEventHooksHolder {
private static final AsyncEventHooks INSTANCE = new AsyncEventHooks();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy