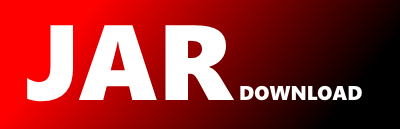
org.zodiac.reactor.lock.config.ReactiveLockInfo Maven / Gradle / Ivy
The newest version!
package org.zodiac.reactor.lock.config;
import java.time.Duration;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
public class ReactiveLockInfo {
private boolean enabled = false;
/**
* global registry key prefix
*/
private String registryKeyPrefix;
/**
* max lock expire duration default is PT3M
*/
private Duration expireAfter = Duration.ofMinutes(3);
/**
* expire evict idle duration default is PT1M
*/
private Duration expireEvictIdle = Duration.ofMinutes(1);
/**
* lock type
*/
private Set type = new HashSet<>();
public ReactiveLockInfo() {
super();
}
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public String getRegistryKeyPrefix() {
return registryKeyPrefix;
}
public void setRegistryKeyPrefix(String registryKeyPrefix) {
this.registryKeyPrefix = registryKeyPrefix;
}
public Duration getExpireAfter() {
return expireAfter;
}
public void setExpireAfter(Duration expireAfter) {
this.expireAfter = expireAfter;
}
public Duration getExpireEvictIdle() {
return expireEvictIdle;
}
public void setExpireEvictIdle(Duration expireEvictIdle) {
this.expireEvictIdle = expireEvictIdle;
}
public Set getType() {
return type;
}
public void setType(Set type) {
this.type = type;
}
public void init() {
if (type.isEmpty()) {
type.add(ReactiveLockType.DEFAULT);
}
}
@Override
public int hashCode() {
return Objects.hash(enabled, expireAfter, expireEvictIdle, registryKeyPrefix, type);
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ReactiveLockInfo other = (ReactiveLockInfo)obj;
return enabled == other.enabled && Objects.equals(expireAfter, other.expireAfter)
&& Objects.equals(expireEvictIdle, other.expireEvictIdle)
&& Objects.equals(registryKeyPrefix, other.registryKeyPrefix) && Objects.equals(type, other.type);
}
@Override
public String toString() {
return "[enabled=" + enabled + ", registryKeyPrefix=" + registryKeyPrefix + ", expireAfter="
+ expireAfter + ", expireEvictIdle=" + expireEvictIdle + ", type=" + type + "]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy