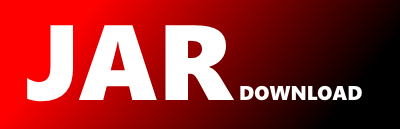
org.zodiac.reactor.util.FluxStreamUtil Maven / Gradle / Ivy
The newest version!
package org.zodiac.reactor.util;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import reactor.util.context.Context;
import java.io.BufferedOutputStream;
import java.io.Closeable;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Arrays;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public abstract class FluxStreamUtil {
private FluxStreamUtil() {
super();
}
private static Logger log = LoggerFactory.getLogger(FluxStreamUtil.class);
public static Flux buffer(int buffer, Function> streamConsumer) {
return Flux.create(sink -> {
OutputStream stream = new BufferedOutputStream(new OutputStream() {
@Override
public void write(byte[] b, int off, int len) {
if (len == b.length) {
sink.next(b);
} else {
sink.next(Arrays.copyOfRange(b, off, off + len));
}
}
@Override
public void write(byte[] b) {
sink.next(b);
}
@Override
public void write(int b) {
sink.next(new byte[]{(byte) b});
}
}, buffer) {
@Override
public void close() throws IOException {
try {
super.close();
} finally {
sink.complete();
}
}
};
/*
sink.onDispose(
streamConsumer
.apply(stream)
.subscribe(ignore -> {
},
sink::error,
() -> {
},
Context.of(sink.contextView())));
*/
sink.onDispose(
streamConsumer
.apply(stream)
.subscribe(ignore -> {
},
sink::error,
() -> {
},
Context.of(sink.currentContext().stream().collect(Collectors.toMap(
Entry::getKey, it -> Optional.ofNullable(it.getValue()).orElse(""),
(oldData, newData) -> newData)
))));
});
}
public static void safeClose(Closeable closeable) {
try {
closeable.close();
} catch (Throwable err) {
log.warn(err.getMessage(), err);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy