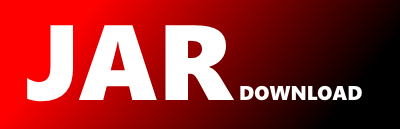
org.zodiac.scaff.crud.web.SaveController Maven / Gradle / Ivy
package org.zodiac.scaff.crud.web;
import io.swagger.annotations.ApiOperation;
import org.zodiac.authorization.api.Authentication;
import org.zodiac.authorization.api.annotation.Authorize;
import org.zodiac.authorization.api.annotation.SaveAction;
//import io.swagger.v3.oas.annotations.Operation;
import org.zodiac.fastorm.rdb.mapping.SyncRepository;
import org.zodiac.fastorm.rdb.mapping.defaults.SaveResult;
import org.zodiac.scaff.api.crud.entity.RecordCreationEntity;
import org.zodiac.scaff.api.crud.entity.RecordModificationEntity;
import org.springframework.web.bind.annotation.PatchMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import java.util.List;
public interface SaveController {
@Authorize(ignore = true)
SyncRepository getRepository();
@Authorize(ignore = true)
default E applyCreationEntity(Authentication authentication, E entity) {
RecordCreationEntity creationEntity = ((RecordCreationEntity) entity);
creationEntity.setCreateTimeNow();
creationEntity.setCreatorId(authentication.getUser().getId());
creationEntity.setCreatorName(authentication.getUser().getName());
return entity;
}
@Authorize(ignore = true)
default E applyModifierEntity(Authentication authentication, E entity) {
RecordModificationEntity modifierEntity = ((RecordModificationEntity) entity);
modifierEntity.setModifyTimeNow();
modifierEntity.setModifierId(authentication.getUser().getId());
modifierEntity.setModifierName(authentication.getUser().getName());
return entity;
}
@Authorize(ignore = true)
default E applyAuthentication(E entity, Authentication authentication) {
if (entity instanceof RecordCreationEntity) {
entity = applyCreationEntity(authentication, entity);
}
if (entity instanceof RecordModificationEntity) {
entity = applyModifierEntity(authentication, entity);
}
return entity;
}
@PatchMapping
@SaveAction
//@Operation(summary = "Save the data.", description = "If an ID is passed in and the corresponding data exists, try to overwrite it, and add a new one if it does not exist.")
@ApiOperation(value = "Save the data.", notes = "If an ID is passed in and the corresponding data exists, try to overwrite it, and add a new one if it does not exist.")
default SaveResult save(@RequestBody List payload) {
return getRepository()
.save(Authentication
.current()
.map(auth -> {
for (E e : payload) {
applyAuthentication(e, auth);
}
return payload;
})
.orElse(payload)
);
}
@PostMapping("/_batch")
@SaveAction
//@Operation(summary = "Add data in batches.")
@ApiOperation(value = "Add data in batches.")
default int add(@RequestBody List payload) {
return getRepository()
.insertBatch(Authentication
.current()
.map(auth -> {
for (E e : payload) {
applyAuthentication(e, auth);
}
return payload;
})
.orElse(payload)
);
}
@PostMapping
@SaveAction
//@Operation(summary = "Add a single data and return the new data.")
@ApiOperation(value = "Add a single data and return the new data.")
default E add(@RequestBody E payload) {
this.getRepository()
.insert(Authentication
.current()
.map(auth -> applyAuthentication(payload, auth))
.orElse(payload));
return payload;
}
@PutMapping("/{id}")
@SaveAction
//@Operation(summary = "Modify the data according to the ID.")
@ApiOperation(value = "Modify the data according to the ID.")
default boolean update(@PathVariable K id, @RequestBody E payload) {
return getRepository()
.updateById(id, Authentication
.current()
.map(auth -> applyAuthentication(payload, auth))
.orElse(payload))
> 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy