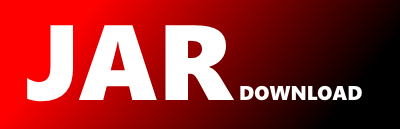
org.zodiac.scaff.crud.web.ServiceQueryController Maven / Gradle / Ivy
package org.zodiac.scaff.crud.web;
//import io.swagger.v3.oas.annotations.Operation;
//import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.annotations.ApiOperation;
import io.swagger.annotations.ApiParam;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.zodiac.authorization.api.annotation.Authorize;
import org.zodiac.authorization.api.annotation.QueryAction;
import org.zodiac.commons.exception.HttpNotFoundException;
import org.zodiac.scaff.api.crud.entity.PagerResult;
import org.zodiac.scaff.api.crud.entity.QueryNoPagingOperation;
import org.zodiac.scaff.api.crud.entity.QueryOperation;
import org.zodiac.scaff.api.crud.entity.QueryParamEntity;
import org.zodiac.scaff.crud.service.CrudService;
import java.util.Collections;
import java.util.List;
/**
* Query controller based on {@link CrudService}.
*
* @param Entity type.
* @param Primary key type.
* @see CrudService
*/
public interface ServiceQueryController {
@Authorize(ignore = true)
CrudService getService();
/**
* Query but do not return paginated result.
*
* GET /_query/no-paging?pageIndex=0&pageSize=20&where=name is Mike&orderBy=id desc
*
* @param query Dynamic query condition.
* @return The result.
* @see QueryParamEntity
*/
@GetMapping("/_query/no-paging")
@QueryAction
@QueryOperation(summary = "Use GET to pagination for dynamic query (no total number is returned).",
description = "This action does not return the total number of paginations. If you want to get all the data, set the parameter paging=false.")
default List query(/*@Parameter(hidden = true)*/ @ApiParam(hidden = true) QueryParamEntity query) {
return getService()
.createQuery()
.setParam(query)
.fetch();
}
/**
* The POST query does not return pagination results.
*
*
* POST /_query/no-paging
*
* {
* "pageIndex":0,
* "pageSize":20,
* "where":"name like Mi%",
* "orderBy":"id desc",
* "terms":[ //Advanced conditions.
* {
* "column":"name",
* "termType":"like",
* "value":"Mi%"
* }
* ]
* }
*
*
* @param query Dynamic query condition.
* @return The result.
* @see QueryParamEntity
*/
@PostMapping("/_query/no-paging")
@QueryAction
//@Operation(summary = "Use the POST method to perform a dynamic query in pagination (the total number is not returned).", description = "This action does not return the total number of paginations. If you want to get all the data, set the parameter paging=false.")
@ApiOperation(value = "Use the POST method to perform a dynamic query in pagination (the total number is not returned).",
notes = "This action does not return the total number of paginations. If you want to get all the data, set the parameter paging=false.")
default List postQuery(@RequestBody QueryParamEntity query) {
return this.query(query);
}
/**
* GET paginated query.
*
* GET /_query/no-paging?pageIndex=0&pageSize=20&where=name is Mike&orderBy=id desc
*
* @param query Dynamic query condition.
* @return The result.
* @see PagerResult
*/
@GetMapping("/_query")
@QueryAction
@QueryOperation(summary = "Use GET to perform dynamic queries in pagination.")
default PagerResult queryPager(/*@Parameter(hidden = true)*/ @ApiParam(hidden = true) QueryParamEntity query) {
if (query.getTotal() != null) {
return PagerResult
.of(query.getTotal(),
getService()
.createQuery()
.setParam(query.rePaging(query.getTotal()))
.fetch(), query)
;
}
int total = getService().createQuery().setParam(query.clone()).count();
if (total == 0) {
return PagerResult.of(0, Collections.emptyList(), query);
}
return PagerResult
.of(total,
getService()
.createQuery()
.setParam(query.rePaging(total))
.fetch(), query);
}
@PostMapping("/_query")
@QueryAction
//@Operation(summary = "Use the POST method to dynamically query the page.")
@ApiOperation(value = "Use the POST method to dynamically query the page.")
default PagerResult postQueryPager(@RequestBody QueryParamEntity query) {
return queryPager(query);
}
@PostMapping("/_count")
@QueryAction
//@Operation(summary = "Use the POST operation to query the total number.")
@ApiOperation(value = "Use the POST operation to query the total number.")
default int postCount(@RequestBody QueryParamEntity query) {
return this.count(query);
}
/**
* Statistical query.
*
* GET /_count
*
* @param query Dynamic query condition.
* @return The result.
*/
@GetMapping("/_count")
@QueryAction
@QueryNoPagingOperation(summary = "Use the GET operation to query the total number.")
default int count(/*@Parameter(hidden = true)*/ @ApiParam(hidden = true) QueryParamEntity query) {
return getService()
.createQuery()
.setParam(query)
.count();
}
@GetMapping("/{id:.+}")
@QueryAction
//@Operation(summary = "Query by ID.")
@ApiOperation(value = "Query by ID.")
default E getById(@PathVariable K id) {
return getService()
.findById(id)
.orElseThrow(HttpNotFoundException::new);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy