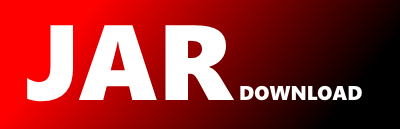
org.zodiac.webui.controller.UiController Maven / Gradle / Ivy
package org.zodiac.webui.controller;
import java.security.Principal;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import javax.annotation.Nullable;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import org.springframework.boot.context.properties.ConstructorBinding;
import org.springframework.util.Assert;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.util.UriComponents;
import org.springframework.web.util.UriComponentsBuilder;
import org.zodiac.webui.WebUiController;
import org.zodiac.webui.extension.UiExtension;
import org.zodiac.webui.extension.UiExtensions;
@WebUiController
public class UiController {
private final String publicUrl;
private final UiExtensions uiExtensions;
private final Settings uiSettings;
public UiController(String publicUrl, UiExtensions uiExtensions, Settings uiSettings) {
this.publicUrl = publicUrl;
this.uiExtensions = uiExtensions;
this.uiSettings = uiSettings;
}
@ModelAttribute(value = "baseUrl", binding = false)
public String getBaseUrl(UriComponentsBuilder uriBuilder) {
UriComponents publicComponents = UriComponentsBuilder.fromUriString(this.publicUrl).build();
if (publicComponents.getScheme() != null) {
uriBuilder.scheme(publicComponents.getScheme());
}
if (publicComponents.getHost() != null) {
uriBuilder.host(publicComponents.getHost());
}
if (publicComponents.getPort() != -1) {
uriBuilder.port(publicComponents.getPort());
}
if (publicComponents.getPath() != null) {
uriBuilder.path(publicComponents.getPath());
}
return uriBuilder.path("/").toUriString();
}
@ModelAttribute(value = "uiSettings", binding = false)
public Settings getUiSettings() {
return this.uiSettings;
}
@ModelAttribute(value = "cssExtensions", binding = false)
public List getCssExtensions() {
return this.uiExtensions.getCssExtensions();
}
@ModelAttribute(value = "jsExtensions", binding = false)
public List getJsExtensions() {
return this.uiExtensions.getJsExtensions();
}
// @ModelAttribute(value = "user", binding = false)
public Map getUser(@Nullable Principal principal) {
if (principal != null) {
return Collections.singletonMap("name", principal.getName());
}
return Collections.emptyMap();
}
// @GetMapping(path = StringPool.SLASH, produces = MediaType.TEXT_HTML_VALUE)
public String index() {
return "index";
}
@GetMapping(path = "/ui-settings.js", produces = "application/javascript")
public String uiSettings() {
return "ui-settings.js";
}
// @GetMapping(path = "/login", produces = MediaType.TEXT_HTML_VALUE)
public String login() {
return "login";
}
public static class Settings {
private final String title;
private final String brand;
private final String loginIcon;
private final String favicon;
private final String faviconDanger;
private final boolean notificationFilterEnabled;
private final boolean rememberMeEnabled;
private final List availableLanguages;
private final List routes;
private final List externalViews;
public Settings() {
this(null, null, null, null, null, false, false, null, null, null);
}
public Settings(String title, String brand, String loginIcon, String favicon, String faviconDanger,
boolean notificationFilterEnabled, boolean rememberMeEnabled, List availableLanguages,
List routes, List externalViews) {
this.title = title;
this.brand = brand;
this.loginIcon = loginIcon;
this.favicon = favicon;
this.faviconDanger = faviconDanger;
this.notificationFilterEnabled = notificationFilterEnabled;
this.rememberMeEnabled = rememberMeEnabled;
this.availableLanguages = availableLanguages;
this.routes = routes;
this.externalViews = externalViews;
}
public String getTitle() {
return title;
}
public String getBrand() {
return brand;
}
public String getLoginIcon() {
return loginIcon;
}
public String getFavicon() {
return favicon;
}
public String getFaviconDanger() {
return faviconDanger;
}
public boolean isNotificationFilterEnabled() {
return notificationFilterEnabled;
}
public boolean isRememberMeEnabled() {
return rememberMeEnabled;
}
public List getAvailableLanguages() {
return availableLanguages;
}
public List getRoutes() {
return routes;
}
public List getExternalViews() {
return externalViews;
}
}
@JsonInclude(Include.NON_EMPTY)
@ConstructorBinding
public static class ExternalView {
/**
* Label to be shown in the navbar.
*/
private final String label;
/**
* Url for the external view to be linked
*/
private final String url;
/**
* Order in the navbar.
*/
private final Integer order;
/**
* Should the page shown as an iframe or open in a new window.
*/
private final boolean iframe;
public ExternalView(String label, String url, Integer order, boolean iframe) {
Assert.hasText(label, "'label' must not be empty");
Assert.hasText(url, "'url' must not be empty");
this.label = label;
this.url = url;
this.order = order;
this.iframe = iframe;
}
public String getLabel() {
return label;
}
public String getUrl() {
return url;
}
public Integer getOrder() {
return order;
}
public boolean isIframe() {
return iframe;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy