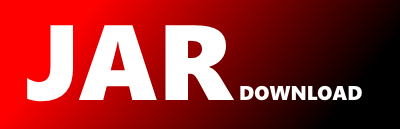
org.zodiac.webui.extension.UiExtensionsScanner Maven / Gradle / Ivy
package org.zodiac.webui.extension;
import java.io.IOException;
import java.util.Arrays;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import javax.annotation.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.ResourcePatternResolver;
import org.zodiac.commons.util.Colls;
public class UiExtensionsScanner {
private static final Logger LOG = LoggerFactory.getLogger(UiExtensionsScanner.class);
private final ResourcePatternResolver resolver;
public UiExtensionsScanner(ResourcePatternResolver resolver) {
this.resolver = resolver;
}
public UiExtensions scan(String... locations) throws IOException {
List extensions = Colls.list();
for (String location : locations) {
for (Resource resource : resolveAssets(location)) {
String resourcePath = this.getResourcePath(location, resource);
if (resourcePath != null && resource.isReadable()) {
UiExtension extension = new UiExtension(resourcePath, location + resourcePath);
LOG.debug("Found Web ui extension {}", extension);
extensions.add(extension);
}
}
}
return new UiExtensions(extensions);
}
private List resolveAssets(String location) throws IOException {
String widerLocation = toPattern(location);
return Stream.concat(Arrays.stream(this.resolver.getResources(widerLocation + "**/*.js")),
Arrays.stream(this.resolver.getResources(widerLocation + "**/*.css"))).collect(Collectors.toList());
}
private String toPattern(String location) {
/*replace the classpath pattern to search all locations and not just the first*/
return location.replace("classpath:", "classpath*:");
}
@Nullable
private String getResourcePath(String location, Resource resource) throws IOException {
String locationWithoutPrefix = location.replaceFirst("^[^:]+:", "");
Matcher m =
Pattern.compile(Pattern.quote(locationWithoutPrefix) + "(.+)$").matcher(resource.getURI().toString());
if (m.find()) {
return m.group(1);
} else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy