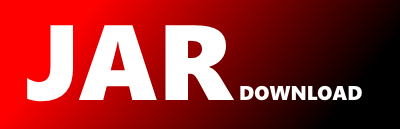
types.TrigCosNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tx2ex Show documentation
Show all versions of tx2ex Show documentation
A library that parses and evaluates mathematical formulas in textual format.
The newest version!
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package types;
import core.NodeRecognizedInfo;
import core.VariablesManager;
import exceptions.NonIntegralIndexException;
import java.util.ArrayList;
import java.util.List;
import core.Token;
import core.Utils;
import exceptions.EvaluationException;
import exceptions.InvalidFormatException;
/**
*
* @author haitham
*/
public class TrigCosNode extends AbstractNode {
public TrigCosNode(AbstractNode parent, List allTokens) {
super(parent, allTokens);
}
@Override
public double evaluate() throws EvaluationException {
return Math.cos(getChild(0).evaluate());
}
public static NodeRecognizedInfo findLastOccurence(
List targetTokens,
List allTtokens,
AbstractNode parent,
VariablesManager vm) throws InvalidFormatException {
// Loop over all tokens from the end to the beginning
for (int i = targetTokens.size() - 2; i >= 0; i--) {
// Check if the token is enclosed in brackets, braces or parentheses
if (!Utils.surrounded(targetTokens.get(i), targetTokens)) {
// Try to match the operator
if (targetTokens.get(i).getString().equalsIgnoreCase("cos")
&& targetTokens.get(i + 1).getString().equals("(")) {
// Look for ")"
int j = i;
int last = -1;
while (j < targetTokens.size()) {
if (targetTokens.get(j).getString().equals(")")) {
last = j;
}
j++;
}
// Now (last) points to the location of the ")" token
if (last == -1) {
// This means that the closing ")" is missing
throw new InvalidFormatException(
targetTokens.get(i),
allTtokens,
"Un-balanced parentheses, Cosine closing bracket ')' is missing.");
} else {
// List of lists of tokens (will only contain one
// list here as TrigCosNode has only one child)
List> childrenTokensLists = new ArrayList<>();
// Create the tokens of the single child of the this operator
List childTokens = new ArrayList<>();
for (int k = i + 2; k < last; k++) {
childTokens.add(targetTokens.get(k));
}
childrenTokensLists.add(childTokens);
// Return
return new NodeRecognizedInfo(
i,
last,
new TrigCosNode(parent, allTtokens),
childrenTokensLists);
}
}
}
}
// Return null if the operator was not found
return null;
}
@Override
public String toString() {
return String.format("cos(%s)", getChild(0).toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy