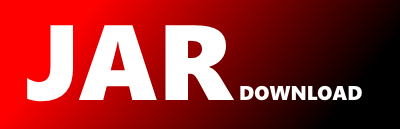
com.zabbix4j.image.Image Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Zabbix4j Show documentation
Show all versions of Zabbix4j Show documentation
Zabbix API library for the Java language.
package com.zabbix4j.image;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.zabbix4j.ZabbixApiException;
import com.zabbix4j.ZabbixApiMethod;
/**
* @author Suguru Yajima
*/
public class Image extends ZabbixApiMethod {
public Image(String apiUrl, String auth) {
super(apiUrl, auth);
}
public ImageCreateResponse create(ImageCreateRequest request) throws ZabbixApiException {
ImageCreateResponse response = null;
request.setAuth(auth);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String requestJson = gson.toJson(request);
try {
String responseJson = sendRequest(requestJson);
response = gson.fromJson(responseJson, ImageCreateResponse.class);
} catch (ZabbixApiException e) {
throw new ZabbixApiException(e);
}
return response;
}
public ImageDeleteResponse delete(ImageDeleteRequest request) throws ZabbixApiException {
ImageDeleteResponse response = null;
request.setAuth(auth);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String requestJson = gson.toJson(request);
try {
String responseJson = sendRequest(requestJson);
response = gson.fromJson(responseJson, ImageDeleteResponse.class);
} catch (ZabbixApiException e) {
throw new ZabbixApiException(e);
}
return response;
}
public ImageUpdateResponse update(ImageUpdateRequest request) throws ZabbixApiException {
ImageUpdateResponse response = null;
request.setAuth(auth);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String requestJson = gson.toJson(request);
try {
String responseJson = sendRequest(requestJson);
response = gson.fromJson(responseJson, ImageUpdateResponse.class);
} catch (ZabbixApiException e) {
throw new ZabbixApiException(e);
}
return response;
}
public ImageGetResponse get(ImageGetRequest request) throws ZabbixApiException {
ImageGetResponse response = null;
request.setAuth(auth);
Gson gson = new GsonBuilder().setPrettyPrinting().create();
String requestJson = gson.toJson(request);
try {
String responseJson = sendRequest(requestJson);
response = gson.fromJson(responseJson, ImageGetResponse.class);
} catch (ZabbixApiException e) {
throw new ZabbixApiException(e);
}
return response;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy