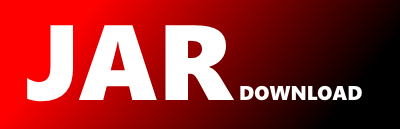
com.github.q120011676.xhttp.HttpRequest Maven / Gradle / Ivy
package com.github.q120011676.xhttp;
import javax.activation.MimetypesFileTypeMap;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLSocketFactory;
import java.io.*;
import java.net.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by say on 1/20/16.
*/
public class HttpRequest implements Request {
private String urlStr;
private Proxy proxy;
private SSLSocketFactory sslsf;
private HostnameVerifier hv;
private Integer connectTimeout;
private Integer readTimeout;
private Boolean followRedirects;
private String character;
private String responseCharacter;
private Map requestHeaders = new HashMap();
private String method = HttpMethod.GET;
private String boundary;
private final static String LOGO = "xhttp";
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy