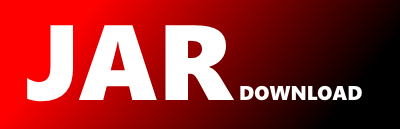
jdbi_modules.internal.RowView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
An Api for modular SQL-Querys and Mapping in java
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy