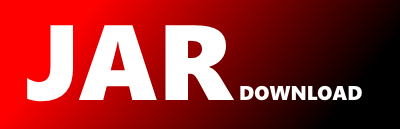
com.github.andyshao.context.CommonContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.context;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import java.util.function.Supplier;
/**
*
*
* Title:
* Descript:
* Copyright: Copryright(c) Jun 11, 2019
* Encoding: UNIX UTF-8
*
* @author Andy.Shao
*
*/
public interface CommonContext extends Map {
/**
* Set value by key name
* @param key {@link ContextKey}
* @param value the value which should be setted
* @return the previous value of the {@link CommonContext}
* @param data type
*/
@SuppressWarnings("unchecked")
default T setByKey(ContextKey key, T value) {
return (T) put(key.keyName() , value);
}
/**
* Set if the key is absent, at same time
* the new value will be set in the {@link CommonContext}
* @param key {@link ContextKey}
* @param function the value generation {@link Function}
* @return the previous value
* @param data type
*/
@SuppressWarnings("unchecked")
default T computeIfAbsent(ContextKey key, Function , T> function) {
return (T) computeIfAbsent(key.keyName() , k -> function.apply(key));
}
/**
* Does {@link CommonContext} contains the key
* @param key {@link ContextKey}
* @return if the {@link CommonContext} contains the key,
* then return true. Else return false.
* @param data type
*/
default boolean containsKey(ContextKey key) {
return containsKey(key.keyName());
}
/**
* Get value by {@link ContextKey}
* @param key {@link ContextKey}
* @return the value which is stored in {@link CommonContext}
* @param data type
*/
@SuppressWarnings("unchecked")
default T getByKey(ContextKey key) {
return (T) get(key.keyName());
}
/**
* Get value by key.
* If the key does not exist in the {@link CommonContext},
* then return the default value
* @param key {@link ContextKey}
* @param defaultValue the default value
* @return the value
* @param data type
*/
default T getOrDefaultByKey(ContextKey key, T defaultValue) {
return getOpByKey(key)
.orElse(defaultValue);
}
/**
* Get value by key.
* If the key does not exist, then throw exception
* @param key {@link ContextKey}
* @param exception the exception which maybe threw
* @return the value
* @param the data type
* @param the exception type
* @throws X the exception
*/
default T getOrThrow(ContextKey key, Supplier extends X> exception) throws X {
return getOpByKey(key)
.orElseThrow(exception);
}
/**
* Get the value by key.
* If the key does not exist, then generate one.
* But the generated value does not be stored in the {@link CommonContext}
* @param key {@link ContextKey}
* @param function the value generation function
* @return the value
* @param data type
*/
default T computeIfAbsence(ContextKey key, Function, T> function) {
return getOpByKey(key)
.orElseGet(() -> function.apply(key));
}
/**
* Get a optional value
* @param key {@link ContextKey}
* @return a optional value
* @param data type
*/
default Optional getOpByKey(ContextKey key) {
return Optional.ofNullable(getByKey(key));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy