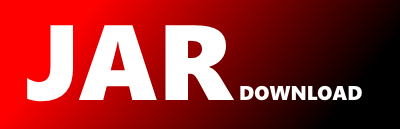
com.github.andyshao.data.structure.CycleLinked Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.data.structure;
import java.io.Serial;
import java.io.Serializable;
import java.util.ConcurrentModificationException;
import java.util.Iterator;
import java.util.function.Function;
/**
*
* Title: Cycle Linked interface
* Descript:
* Copyright: Copryright(c) Feb 9, 2015
* Encoding:UNIX UTF-8
*
* @author Andy.Shao
*
* @param data
*/
public class CycleLinked implements Linked> {
@Serial
private static final long serialVersionUID = -1242792864988506815L;
/**
* Default Iterator
*/
class MyIterator implements Iterator, Serializable {
@Serial
private static final long serialVersionUID = -4771582456239944351L;
private final long actionCount = CycleLinked.this.actionAccount;
private volatile CycleLinkedElmt index = CycleLinked.this.head();
private volatile int position = 0;
@Override
public boolean hasNext() {
if (this.actionCount != CycleLinked.this.actionAccount) throw new ConcurrentModificationException();
return this.position < CycleLinked.this.size();
}
@Override
public DATA next() {
CycleLinkedElmt result = this.index;
this.index = this.index.next();
this.position++;
return result.data();
}
}
/**
* Get default {@link CycleLinked}
* @return {@link CycleLinked}
* @param data type
*/
public static CycleLinked defaultCycleLinked() {
return CycleLinked.defaultCycleLinked(CycleLinkedElmt::defaultElmt);
}
/**
* Get default {@link CycleLinked}
* @param elmtFactory {@link CycleLinkedElmt} factory
* @return {@link CycleLinked}
* @param data type
*/
public static CycleLinked defaultCycleLinked(Function> elmtFactory) {
return new CycleLinked(elmtFactory);
}
/**action account*/
private long actionAccount = 0;
/**element factory*/
private final Function> elmtFactory;
/**head of the elements*/
private CycleLinkedElmt head;
/**size*/
private int size;
/**
* Build {@link CycleLinked}
* @param elmtFactory {@link CycleLinkedElmt} factory
*/
public CycleLinked(Function> elmtFactory) {
this.elmtFactory = elmtFactory;
}
@Override
public void clear() {
do
if (this.size == 0) return;
else if (this.size == 1) {
this.head.free();
this.head = null;
this.size = 0;
return;
} else this.remNext(this.head);
while (this.size != 0);
}
@Override
public Function> getElmtFactory() {
return this.elmtFactory;
}
@Override
public CycleLinkedElmt head() {
return this.head;
}
@Override
public void insNext(CycleLinkedElmt element , final DATA data) {
CycleLinkedElmt new_element = this.getElmtFactory().apply(data);
if (this.size == 0) {
//Handle insertion when the list is empty.
new_element.setNext(new_element);
this.head = new_element;
} else if (element == null) {
new_element.setNext(this.head);
this.head = new_element;
} else {
//Handle insertion when the list is not empty.
new_element.setNext(element.next());
element.setNext(new_element);
}
//Adjust the size of the list to account for the inserted element.
this.size++;
this.actionAccount++;
}
@Override
public Iterator iterator() {
return new MyIterator();
}
@Override
public DATA remNext(CycleLinkedElmt element) {
CycleLinkedElmt old_element = this.getElmtFactory().apply(null);
DATA data = null;
//Do not allow removal from an empty list.
if (this.size == 0) throw new LinkedOperationException("Do not allow removal from an empty list.");
if (element == null) element = this.head;
//Remove the element from the list.
data = element.next().data();
if (element.next() == element) {
//Handle removing the last element.
old_element = element.next();
this.head = null;
} else {
//Handle removing other than the last element.
old_element = element.next();
element.setNext(element.next().next());
if (old_element == this.head) this.head = old_element.next();
}
old_element.free();
//Adjust the size of the list to account for the removed element.
this.size--;
this.actionAccount++;
return data;
}
@Override
public int size() {
return this.size;
}
@Override
public CycleLinkedElmt tail() {
return this.head;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy