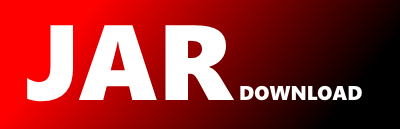
com.github.andyshao.data.structure.Linked Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.data.structure;
import com.github.andyshao.util.CollectionModel;
import java.io.Serializable;
import java.util.Collection;
import java.util.Objects;
import java.util.function.Function;
/**
*
* Title: Linked list interface
* Descript: Because of it is a linked list, you should use the {@link #clear()}
* method when you
* want to remove this list.
* Copyright: Copryright(c) Feb 8, 2015
* Encoding:UNIX UTF-8
*
* @author Andy.Shao
*
* @param data
* @param element type
*/
public interface Linked> extends CollectionModel, Serializable {
/**
* Link element
* @param data type
* @param element type
*/
public interface LinkedElmt> extends Serializable {
/**
* Get data
* @return the data
*/
public abstract DATA data();
/**
* Clean element data
*/
public default void free() {
this.setData(null);
this.setNext(null);
}
/**
* Get next {@link LinkedElmt}
* @return {@link LinkedElmt}
*/
public abstract T next();
/**
* Set data
* @param data the data
*/
public abstract void setData(DATA data);
/**
* Set next {@link LinkedElmt}
* @param next {@link LinkedElmt}
*/
public abstract void setNext(T next);
}
@Override
public default boolean add(D e) {
Linked.this.insNext(Linked.this.tail() , e);
return true;
}
/**
* Get {@link LinkedElmt} factory
* @return {@link LinkedElmt} factory
*/
public Function getElmtFactory();
/**
* The head {@link LinkedElmt}
* @return {@link LinkedElmt}
*/
public T head();
/**
* Insert next {@link LinkedElmt} from element
* @param element {@link LinkedElmt}
* @param data data
*/
public void insNext(T element , final D data);
/**
* Remove the next element.
* If the element is the tail, Then it won't remove anything.
*
* @param element the item of linked's
* @return if something is removed return data. If it doesn't return null.
* @throws LinkedOperationException the operation of remove node
*/
public D remNext(T element);
@Override
public default boolean remove(Object o) {
T prev = null;
for (T element = Linked.this.head() ; element != null ; element = element.next()) {
if (Objects.equals(element.data() , o)) {
Linked.this.remNext(prev);
break;
}
prev = element;
}
return true;
}
@Override
public default boolean retainAll(Collection> c) {
T prev = null;
for (T element = Linked.this.head() ; element != null ; element = element.next())
if (!c.contains(element.data())) Linked.this.remNext(prev);
else prev = element;
return true;
}
@Override
public int size();
/**
* The tail {@link LinkedElmt} of {@link Linked}
* @return {@link LinkedElmt}
*/
public T tail();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy