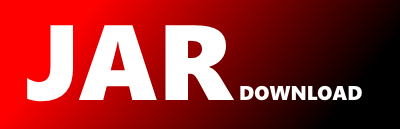
com.github.andyshao.data.structure.SingleLinked Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.data.structure;
import java.io.Serial;
import java.io.Serializable;
import java.util.ConcurrentModificationException;
import java.util.Iterator;
import java.util.Objects;
import java.util.function.Function;
/**
*
* Title: Single Linked interface
* Descript:
* Copyright: Copryright(c) Feb 8, 2015
* Encoding:UNIX UTF-8
*
* @author Andy.Shao
*
* @param data
*/
public class SingleLinked implements Linked> {
@Serial
private static final long serialVersionUID = -10212710722830693L;
/**
* Default iterator
*/
private class MyIterator implements Iterator, Serializable {
@Serial
private static final long serialVersionUID = -8175296928562043751L;
/**action count*/
private final long actionCount = SingleLinked.this.actionCount;
/**{@link CycleLinkedElmt}*/
private volatile CycleLinkedElmt index = SingleLinked.this.head();
@Override
public boolean hasNext() {
if (this.actionCount != SingleLinked.this.actionCount) throw new ConcurrentModificationException();
return this.index != null;
}
@Override
public D next() {
CycleLinkedElmt result = this.index;
this.index = this.index.next();
return result.data();
}
}
/**
* Get the default {@link SingleLinked}
* @return {@link SingleLinked}
* @param data type
*/
public static SingleLinked defaultSingleLinked() {
return SingleLinked.defaultSingleLinked(CycleLinkedElmt::defaultElmt);
}
/**
* Get the default {@link SingleLinked}
* @param cycleLinkedElmt {@link CycleLinkedElmt} factory
* @return {@link SingleLinked}
* @param data type
*/
public static SingleLinked defaultSingleLinked(Function> cycleLinkedElmt) {
return new SingleLinked(cycleLinkedElmt);
}
/**action count*/
private long actionCount = 0;
/**{@link CycleLinkedElmt} factory*/
private final Function> cycleLinkedElmtFactory;
/**head of the elements*/
private CycleLinkedElmt head;
/**size*/
private int size = 0;
/**tail of the elements*/
private CycleLinkedElmt tail;
/**
* Build the {@link SingleLinked}
* @param cycleLinkedElmtFactory the {@link CycleLinkedElmt} factory
*/
public SingleLinked(Function> cycleLinkedElmtFactory) {
this.cycleLinkedElmtFactory = cycleLinkedElmtFactory;
}
@Override
public void clear() {
do
if (this.size == 0) return;
else if (this.size == 1) {
this.head.free();
this.head = null;
this.tail = null;
this.size = 0;
return;
} else this.remNext(this.head);
while (this.size != 0);
}
@SuppressWarnings("unchecked")
@Override
public boolean equals(Object obj) {
SingleLinked that;
if (obj instanceof SingleLinked) {
that = (SingleLinked) obj;
return this.size() == that.size() && Objects.equals(this.head() , that.head()) && Objects.equals(this.tail() , that.tail());
} else return false;
}
@Override
public Function> getElmtFactory() {
return this.cycleLinkedElmtFactory;
}
@Override
public int hashCode() {
return Objects.hash(this.size() , this.head() , this.tail());
}
@Override
public CycleLinkedElmt head() {
return this.head;
}
@Override
public void insNext(CycleLinkedElmt element , final D data) {
CycleLinkedElmt new_element = this.cycleLinkedElmtFactory.apply(data);
if (element == null) {
//Handle insertion at the head of the list.
if (this.size() == 0) this.tail = new_element;
new_element.setNext(this.head);
this.head = new_element;
} else {
//Handle insertion somewhere other than at the head.
if (element.next() == null) this.tail = new_element;
new_element.setNext(element.next());
element.setNext(new_element);
}
//Adjust the size of the list to account for the inserted element.
this.size++;
this.actionCount++;
}
@Override
public Iterator iterator() {
return this.new MyIterator();
}
@Override
public D remNext(CycleLinkedElmt element) {
CycleLinkedElmt old_element = this.cycleLinkedElmtFactory.apply(null);
D data = null;
if (this.size() == 0) throw new LinkedOperationException("Do not allow removal from an empty list.");
//Remove the element from the list.
if (element == null) {
//Handle removal from the head of the list.
data = this.head.data();
old_element = this.head;
this.head = this.head.next();
if (this.size() == 1) this.tail = null;
} else {
if (element.next() == null) return null;
data = element.next().data();
old_element = element.next();
element.setNext(element.next().next());
if (element.next() == null) this.tail = element;
}
old_element.free();
//Adjust the size of the list of account for the removed element.
this.size--;
this.actionCount++;
return data;
}
@Override
public int size() {
return this.size;
}
@Override
public CycleLinkedElmt tail() {
return this.tail;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy