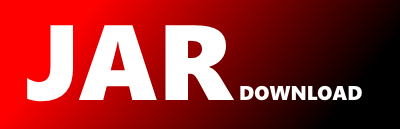
com.github.andyshao.lang.ArrayWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.lang;
import com.github.andyshao.reflect.ArrayOperation;
import java.io.Serializable;
import java.lang.reflect.Array;
import java.util.Iterator;
import java.util.Objects;
import java.util.stream.Stream;
/**
*
* Title:
* Descript:
* Copyright: Copryright(c) Oct 1, 2015
* Encoding:UNIX UTF-8
*
* @author Andy.Shao
* @see ByteArrayWrapper
* @see IntArrayWrapper
* @see CharArrayWrapper
* @see LongArrayWrapper
* @see ShortArrayWrapper
* @see FloatArrayWrapper
* @see DoubleArrayWrapper
* @see ObjectArrayWrapper
* @see NullArrayWrapper
*/
public interface ArrayWrapper extends Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy