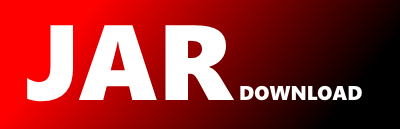
com.github.andyshao.lang.StringOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.lang;
import com.github.andyshao.reflect.ArrayOperation;
import java.util.Comparator;
import java.util.Objects;
/**
*
* Title: some usefu tools of java.lang.String
* Descript:
* Copyright: Copryright(c) Apr 28, 2014
* Encoding:UNIX UTF-8
*
* @author Andy.Shao
*
*/
public final class StringOperation {
/**Default String {@link java.util.Comparator}*/
public static final Comparator COMPARATOR = Comparator.comparing(it -> it);
/**
* flip string
* @param str a string which should be flipped
* @return A string which has flipped.
*/
public static String flipString(String str) {
return new String(ArrayOperation.flipArray(str.toCharArray()));
}
/**
* is empty or null
* @param str a string which should be tested.
* @return if the str is null or "", return the true
*/
public static boolean isEmptyOrNull(String str) {
return str == null || str.isEmpty();
}
/**
* is trim empty or null
* @param str a string which should be tested
* @return if str is null or after {@link String#trim()} and {@link String#isEmpty()} return true
*/
public static boolean isTrimEmptyOrNull(String str) {
return str == null || str.trim().isEmpty();
}
/**
* more efficient
* replace the string
*
* @param str the string which will be process
* @param padding the right words will be inputed
* @param index the right words will be inputed
* @param length the length of string which should be replaced
* @return the end of the string
*/
static String replace(String str , String padding , int index , int length) {
String result;
if (index == -1) result = str;
else if (index == 0) result = padding + str.substring(length);
else if (index + 1 >= str.length()) result = str.substring(0 , index) + padding;
else result = str.substring(0 , index) + padding + str.substring(index + length);
return result;
}
/**
* more efficient
* replace all of the key words
*
* @param str the string which will be process
* @param key the key words will be instead
* @param padding the right words will be inputed
* @return the end of the string
*/
public static String replaceAll(String str , String key , String padding) {
if(Objects.equals(key , padding)) return str;
while (str.indexOf(key) != -1)
str = StringOperation.replaceFirst(str , key , padding);
return str;
}
/**
* more efficient
* only replace the first time occur
*
* @param str the string which will be process
* @param key the key words will be insted
* @param padding the right words will be inputed
* @return the end of the string
*/
public static String replaceFirst(String str , String key , String padding) {
return StringOperation.replace(str , padding , str.indexOf(key) , key.length());
}
/**
* more efficient
* only replace the last time occur
*
* @param str the string which will be process
* @param key the key words will be insted
* @param padding the right words will be inputed
* @return the end of the string
*/
public static String replaceLast(String str , String key , String padding) {
return StringOperation.replace(str , padding , str.lastIndexOf(key) , key.length());
}
/**
* more efficient
* split the string by separator
*
* @param str the string which will be process
* @param separator the str will be splited by it
* @return the end of string
*/
public static String[] split(String str , String separator) {
String[] result = new String[0];
for (int index ; (index = str.indexOf(separator)) != -1 ;) {
result = ArrayOperation.mergeArray(String[].class , result , new String[] { str.substring(0 , index) });
str = str.substring(index + separator.length());
}
if (str.length() != 0) result = ArrayOperation.mergeArray(String[].class , result , new String[] { str });
return result;
}
private StringOperation() {
throw new AssertionError("Not allowed to instance " + StringOperation.class.getName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy