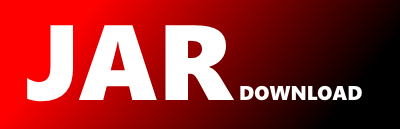
com.github.andyshao.util.ObjectOperation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.util;
import com.github.andyshao.util.stream.Pair;
import java.util.Iterator;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
*
* Title:
* Descript:
* Copyright: Copryright(c) Jan 26, 2016
* Encoding:UNIX UTF-8
*
* @author Andy.Shao
*
*/
public final class ObjectOperation {
/**
* function not null or default
* @param t input
* @param function {@link Function}
* @param def default
* @return function result or default value
* @param input type
* @param output type
*/
public static final R functionNonNullOrDefault(T t, Function function, R def) {
return functionNonNullOrElseGet(t, function, () -> def);
}
/**
* function not null or else get
* @param t input
* @param function {@link Function}
* @param supplier default factory
* @return function result or default value
* @param input type
* @param output type
*/
public static final R functionNonNullOrElseGet(T t, Function function, Supplier supplier) {
return functionAfterTestOrElseGet(t, it -> Objects.nonNull(t), function, supplier);
}
/**
* function after test or else get
* @param t input
* @param predicate {@link Predicate}
* @param function {@link Function}
* @param supplier default factory
* @return function result or default value
* @param input type
* @param output type
*/
public static final R functionAfterTestOrElseGet(
T t, Predicate predicate, Function function, Supplier supplier) {
if(predicate.test(t)) return function.apply(t);
return supplier.get();
}
/**
* is same object
* @param thiz object A
* @param that object B
* @return if it is then true
*/
public static final boolean isSameObj(Object thiz, Object that) {
return thiz == that;
}
/**
* is pass equals and hashCode
* @param thiz Object A
* @param that Object B
* @return if it is then true
*/
public static final boolean isPassEqualsAndHashCode(Object thiz, Object that) {
if(thiz == null || that == null) return false;
return thiz.equals(that) && thiz.hashCode() == that.hashCode();
}
/**
* value or default
* @param value value
* @param def default value
* @return value or default
* @param data type
*/
public static final T valueOrDefault(T value , T def) {
return Objects.isNull(value) ? def : value;
}
/**
* equals any one return item
* @param value value
* @param compareList compare list
* @return compare result
*/
public static final Pair
© 2015 - 2025 Weber Informatics LLC | Privacy Policy