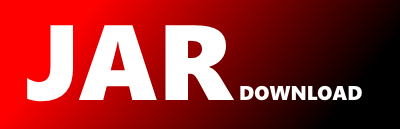
com.github.andyshao.util.function.ExceptionableBiPredicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.util.function;
import com.github.andyshao.lang.Convert;
import com.github.andyshao.util.stream.RuntimeExceptionFactory;
import java.util.Objects;
import java.util.function.BiPredicate;
/**
*
*
* Title:
* Descript:
* Copyright: Copryright(c) May 28, 2019
* Encoding: UNIX UTF-8
*
* @author Andy.Shao
*
* @param argument type one
* @param argument type two
* @see BiPredicate
*/
@FunctionalInterface
public interface ExceptionableBiPredicate {
/**
* test
* @param t left
* @param u right
* @return is pass the test
* @throws Throwable any error
*/
boolean test(T t, U u) throws Throwable;
/**
* to {@link BiPredicate}
* @param f exception convert factory
* @return {@link Convert}
* @param left type
* @param right type
*/
static Convert, BiPredicate> toBiPredicate(RuntimeExceptionFactory> f) {
return input -> {
return (t, u) -> {
try {
return input.test(t, u);
} catch (Throwable e) {
throw f.build(e);
}
};
};
}
/**
* to {@link BiPredicate}
* @return {@link Convert}
* @param left type
* @param right type
*/
static Convert, BiPredicate> toBiPredicate() {
return toBiPredicate(RuntimeExceptionFactory.DEFAULT);
}
/**
* and operation
* @param other another {@link ExceptionableBiPredicate}
* @return new {@link ExceptionableBiPredicate}
*/
default ExceptionableBiPredicate and(ExceptionableBiPredicate super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) && other.test(t, u);
}
/**
* negate operation
* @return {@link ExceptionableBiPredicate}
*/
default ExceptionableBiPredicate negate() {
return (T t, U u) -> !test(t, u);
}
/**
* negate operation
* @param predicate predicate operation
* @return {@link ExceptionableBiPredicate}
* @param left type
* @param right type
*/
static ExceptionableBiPredicate negate(ExceptionableBiPredicate predicate) {
return predicate.negate();
}
/**
* or operation
* @param other another {@link ExceptionableBiPredicate}
* @return new {@link ExceptionableBiPredicate}
*/
default ExceptionableBiPredicate or(ExceptionableBiPredicate super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) || other.test(t, u);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy