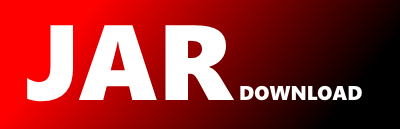
com.github.andyshao.util.function.ThrowableFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.util.function;
import com.github.andyshao.lang.Convert;
import com.github.andyshao.util.stream.ThrowableException;
import java.util.Objects;
import java.util.function.Function;
/**
*
*
* Title:
* Descript:
* Copyright: Copryright(c) May 27, 2019
* Encoding: UNIX UTF-8
*
* @author Andy.Shao
*
* @param argument type
* @param return type
* @see ExceptionableFunction
* @see Function
* @deprecated repeated
*/
@Deprecated(since = "5.0.0.RELEASE")
@FunctionalInterface
public interface ThrowableFunction {
/**
* apply operation
* @param t input
* @return ret
* @throws Throwable any error
*/
R apply(T t) throws Throwable;
/**
* to {@link ExceptionableConsumer}
* @return {@link ExceptionableConsumer}
* @param data type
* @param return type
*/
static Convert, ExceptionableFunction> toExceptionableFunction() {
return input -> {
return new ExceptionableFunction() {
@Override
public R apply(T t) throws Exception {
try {
return input.apply(t);
} catch (Throwable e) {
throw ThrowableException.convertToException(e);
}
}
};
};
}
/**
* compose operation
* @param before before {@link ThrowableFunction}
* @return a new {@link ThrowableFunction}
* @param data type
*/
default ThrowableFunction compose(ThrowableFunction super V, ? extends T> before) {
Objects.requireNonNull(before);
return v -> this.apply(before.apply(v));
}
/**
* and then
* @param after after {@link ThrowableFunction}
* @return a new {@link Throwable}
* @param data type
*/
default ThrowableFunction andThen(ThrowableFunction super R, ? extends V> after) {
Objects.requireNonNull(after);
return t -> after.apply(this.apply(t));
}
/**
* identity
* @return {@link ThrowableFunction}
* @param data type
*/
static ThrowableFunction identity() {
return t -> t;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy