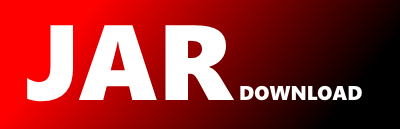
com.github.andyshao.util.function.ThrowablePredicate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.util.function;
import com.github.andyshao.lang.Convert;
import com.github.andyshao.util.stream.ThrowableException;
import java.util.Objects;
import java.util.function.Predicate;
/**
*
*
* Title:
* Descript:
* Copyright: Copryright(c) May 27, 2019
* Encoding: UNIX UTF-8
*
* @author Andy.Shao
*
* @param argument type
* @see ExceptionablePredicate
* @see Predicate
* @deprecated repeated
*/
@Deprecated(since = "5.0.0.RELEASE")
@FunctionalInterface
public interface ThrowablePredicate {
/**
* test operation
* @param t input
* @return ret
* @throws Throwable any error
*/
boolean test(T t) throws Throwable;
/**
* to {@link ExceptionablePredicate}
* @return {@link ExceptionablePredicate}
* @param data type
*/
static Convert, ExceptionablePredicate> toExceptionablePredicate() {
return input -> {
return new ExceptionablePredicate() {
@Override
public boolean test(T t) throws Exception {
try {
return input.test(t);
} catch (Throwable e) {
throw ThrowableException.convertToException(e);
}
}
};
};
}
/**
* and operation
* @param another {@link ThrowablePredicate}
* @return {@link ThrowablePredicate}
*/
default ThrowablePredicate and(ThrowablePredicate super T> another) {
Objects.requireNonNull(another);
return t -> this.test(t) && another.test(t);
}
/**
* negate operation
* @return {@link ThrowablePredicate}
*/
default ThrowablePredicate negate() {
return t -> !this.test(t);
}
/**
* negate operation
* @param predicate {@link ThrowablePredicate}
* @return {@link ThrowablePredicate}
* @param data type
*/
static ThrowablePredicate negate(ThrowablePredicate predicate) {
return predicate.negate();
}
/**
* or operation
* @param another {@link ThrowablePredicate}
* @return {@link ThrowablePredicate}
*/
default ThrowablePredicate or(ThrowablePredicate super T> another) {
Objects.requireNonNull(another);
return t -> this.test(t) || another.test(t);
}
/**
* is equal
* @param targetRef target
* @return is equal
* @param data type
*/
static ThrowablePredicate isEqual(Object targetRef) {
return (null == targetRef)
? Objects::isNull
: object -> targetRef.equals(object);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy