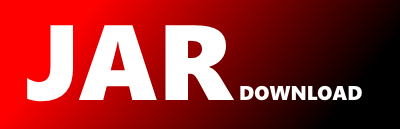
com.github.andyshao.util.stream.Either Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.util.stream;
import com.github.andyshao.util.function.ExceptionableFunction;
import lombok.AccessLevel;
import lombok.RequiredArgsConstructor;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
/**
*
*
* Title:
* Descript:
* Copyright: Copryright(c) May 27, 2019
* Encoding: UNIX UTF-8
*
* @author Andy.Shao
*
* @param left type
* @param right type
*/
@RequiredArgsConstructor(access = AccessLevel.PRIVATE)
public final class Either {
private final L left;
private final R right;
/**
* set left value
* @param value left value
* @return {@link Either}
* @param left type
* @param right type
*/
public static Either left(L value) {
return new Either(value, null);
}
/**
* set right value
* @param value right value
* @return {@link Either}
* @param left type
* @param right type
*/
public static Either right(R value) {
return new Either(null, value);
}
/**
* get left value
* @return left value
*/
public Optional getLeft() {
return Optional.ofNullable(this.left);
}
/**
* compute left if absence
* @param function default value function
* @return {@link Either#getLeft()} or default value
*/
public Optional computeLeftIfAbsence(Function, L> function) {
Optional ret = this.getLeft();
if(ret.isPresent()) return ret;
return Optional.ofNullable(function.apply(this));
}
/**
* get right value
* @return right value
*/
public Optional getRight() {
return Optional.ofNullable(this.right);
}
/**
* compute right if absence
* @param function default value function
* @return {@link Either#getRight()} or default value
*/
public Optional computeRightIfAbsence(Function, R> function) {
Optional ret = this.getRight();
if(ret.isPresent()) return ret;
return Optional.ofNullable(function.apply(this));
}
/**
* the left side has the value
* @return if it has then true
*/
public boolean isLeft() {
return Objects.nonNull(this.left);
}
/**
* the right side has the value
* @return if it has then true
*/
public boolean isRight() {
return Objects.nonNull(this.right);
}
/**
* map left
* @param mapper mapper function
* @return data
* @param data type
*/
public Optional mapLeft(Function super L, T> mapper) {
if(this.isLeft()) return Optional.of(mapper.apply(this.left));
return Optional.empty();
}
/**
* map left
* @param func {@link Function}
* @return {@link Either}
* @param data type
*/
public Either mapLft(Function super L, T> func) {
if(isLeft()) return Either.left(func.apply(this.left));
else return Either.right(this.right);
}
/**
* flat map left
* @param func {@link Function}
* @return {@link Either}
* @param data type
*/
public Either flatMapLft(Function super L, Either> func) {
if(isLeft()) return func.apply(this.left);
else return Either.right(this.right);
}
/**
* map right
* @param mapper {@link Function}
* @return {@link Either}
* @param data type
*/
public Optional mapRight(Function super R, T> mapper) {
if(this.isRight()) return Optional.of(mapper.apply(this.right));
return Optional.empty();
}
/**
* map right
* @param func {@link Function}
* @return {@link Either}
* @param data type
*/
public Either mapRht(Function super R, T> func) {
if(isRight()) return Either.right(func.apply(this.right));
else return Either.left(this.left);
}
/**
* flat map right
* @param func {@link Function}
* @return {@link Either}
* @param data type
*/
public Either flatMapRht(Function super R, Either> func){
if(isRight()) return func.apply(this.right);
else return Either.left(this.left);
}
/**
* fun expression
* @param function {@link ExceptionableFunction}
* @return {@link Function}
* @param input type
* @param right type
*/
public static Function> funExp(ExceptionableFunction function) {
return t -> {
try {
return Either.right(function.apply(t));
} catch (Throwable e) {
return Either.left(e);
}
};
}
/**
* fun expression with value
* @param function {@link ExceptionableFunction}
* @return {@link Function}
* @param input type
* @param right type
*/
public static Function, R>> funExpWithValue(ExceptionableFunction function) {
return t -> {
try {
return Either.right(function.apply(t));
} catch (Throwable e) {
return Either.left(Pair.of(e, t));
}
};
}
@Override
public String toString() {
if(this.isLeft()) return String.format("Left(%s)", this.left);
return String.format("Right(%s)", this.right);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy