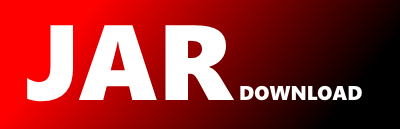
com.github.andyshao.util.stream.Try Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Gear Show documentation
Show all versions of Gear Show documentation
Enhance and formating the coding of JDK
The newest version!
package com.github.andyshao.util.stream;
import com.github.andyshao.util.ExceptionableComparator;
import com.github.andyshao.util.function.*;
import lombok.AccessLevel;
import lombok.Getter;
import lombok.RequiredArgsConstructor;
import java.util.Comparator;
import java.util.Optional;
import java.util.function.*;
/**
*
*
* Title:
* Description:
* Copyright: Copryright(c) May 27, 2019
* Encoding: UNIX UTF-8
*
* @author Andy.Shao
*
* @param argument type
* @param resource type
*/
@Getter
@RequiredArgsConstructor(access = AccessLevel.PRIVATE)
public final class Try {
private final Throwable failure;
private final T argument;
private final R success;
private final boolean isSuccess;
/**
* success operation
* @param argument parameter
* @param resource return value
* @return a success {@link Try}
* @param argument type
* @param resource type
*/
public static Try success(T argument, R resource) {
return new Try<>(null, argument, resource, true);
}
/**
* failure operation
* @param argument parameter
* @param e error
* @return a failure {@link Try}
* @param argument type
* @param resource type
*/
public static Try failure(T argument, Throwable e) {
return new Try<>(e, argument, null, false);
}
/**
* is success
* @return if it is then true
*/
public boolean isSuccess() {
return this.isSuccess;
}
/**
* is failure
* @return if it is then true
*/
public boolean isFailure() {
return !isSuccess();
}
/**
* get argument
* @return return type
*/
public Optional getArgumentOps() {
return Optional.ofNullable(this.argument);
}
/**
* compute if argument absence
* @param supplier {@link Supplier}
* @return argument
*/
public T computeIfArgumentAbsence(Supplier supplier) {
Optional ops = getArgumentOps();
return ops.isPresent() ? ops.get() : supplier.get();
}
/**
* get success result
* @return success result
*/
public Optional getSuccessOps() {
return Optional.ofNullable(this.success);
}
/**
* compute if success absence
* @param supplier {@link Supplier}
* @return success result or default
*/
public R computeIfSuccessAbsence(Supplier supplier) {
Optional successOps = this.getSuccessOps();
return successOps.isPresent() ? successOps.get() : supplier.get();
}
/**
* get failure
* @return the error
*/
public Optional getFailureOps() {
return Optional.ofNullable(this.failure);
}
/**
* compute if failure absence
* @param supplier {@link Supplier}
* @return the error
*/
public Throwable computeIfFailureAbsence(Supplier supplier) {
Optional failureOps = this.getFailureOps();
return failureOps.isPresent() ? failureOps.get() : supplier.get();
}
/**
* double function expression
* @param fun {@link ExceptionableToDoubleFunction}
* @param errorProcess error process
* @return {@link ToDoubleFunction}
* @param data type
*/
public static ToDoubleFunction doubleFunExp(ExceptionableToDoubleFunction fun,
Function, Double> errorProcess) {
return t -> {
try {
return fun.applyAsDouble(t);
} catch (Throwable e) {
return errorProcess.apply(Try.failure(t, e));
}
};
}
/**
* long function expression
* @param fun {@link ExceptionableToLongFunction}
* @param errorProcess error process
* @return {@link ToLongFunction}
* @param data type
*/
public static ToLongFunction longFunExp(ExceptionableToLongFunction fun,
Function, Long> errorProcess) {
return t -> {
try {
return fun.applyAsLong(t);
} catch (Throwable e) {
return errorProcess.apply(Try.failure(t, e));
}
};
}
/**
* int function expression
* @param fun function
* @param errorProcess error process
* @return {@link ToIntFunction}
* @param data type
*/
public static ToIntFunction intFunExp(ExceptionableToIntFunction fun,
Function, Integer> errorProcess) {
return t -> {
try {
return fun.applyAsInt(t);
} catch (Throwable e) {
return errorProcess.apply(Try.failure(t, e));
}
};
}
/**
* compute expression
* @param comp compute
* @param errorProcess error process
* @return {@link Comparator}
* @param data type
*/
public static Comparator compExp(ExceptionableComparator comp,
Function, Void>, Integer> errorProcess) {
return (o1, o2) -> {
try {
return comp.compare(o1, o2);
} catch (Throwable e) {
return errorProcess.apply(Try.failure(Pair.of(o1, o2), e));
}
};
}
/**
* sup expression
* @param supplier {@link Supplier}
* @return {@link Try}
* @param data type
*/
public static Supplier> supExp(ExceptionableSupplier supplier) {
return () -> {
try {
return Try.success(null, supplier.get());
} catch (Throwable e) {
return Try.failure(null, e);
}
};
}
/**
* function expression
* @param function {@link ExceptionableFunction}
* @return {@link Try}
* @param input type
* @param output type
*/
public static Function> funExp(ExceptionableFunction function) {
return t -> {
try {
return Try.success(t, function.apply(t));
} catch (Throwable e) {
return Try.failure(t, e);
}
};
}
/**
* binary function expression
* @param function {@link ExceptionableBiFunction}
* @return {@link Try}
* @param left argument
* @param right argument
* @param output type
*/
public static BiFunction, R>> biFunExp(ExceptionableBiFunction function) {
return (t,u) -> {
try {
return Try.success(Pair.of(t, u), function.apply(t, u));
} catch (Throwable e) {
return Try.failure(Pair.of(t, u), e);
}
};
}
/**
* consumer expression
* @param consumer {@link ExceptionableConsumer}
* @param errorProcess error process
* @return {@link Try}
* @param data type
*/
public static Consumer consumExp(ExceptionableConsumer consumer, Consumer> errorProcess) {
return it -> {
try {
consumer.accept((T) it);
} catch (Throwable e) {
errorProcess.accept(Try.failure(it, e));
}
};
}
/**
* binary consumer expression
* @param consumer {@link ExceptionableConsumer}
* @param errorProcess error process
* @return {@link BiConsumer}
* @param input type
* @param output type
*/
public static BiConsumer biConsumExp(ExceptionableBiConsumer consumer,
Consumer,Void>> errorProcess) {
return (t, u) -> {
try {
consumer.accept(t, u);
} catch (Throwable e) {
errorProcess.accept(Try.failure(Pair.of(t, u), e));
}
};
}
/**
* predicate expression
* @param predicate {@link ExceptionablePredicate}
* @param errorProcess error process
* @return {@link Predicate}
* @param data type
*/
public static Predicate predExp(ExceptionablePredicate predicate,
Function, Boolean> errorProcess) {
return t -> {
try {
return predicate.test(t);
} catch (Throwable e) {
return errorProcess.apply(Try.failure(t, e));
}
};
}
/**
* binary predicate expression
* @param predicate {@link ExceptionablePredicate}
* @param errorProcess error process
* @return {@link BiPredicate}
* @param input type
* @param output type
*/
public static BiPredicate biPredExp(ExceptionableBiPredicate predicate,
Function, Void>, Boolean> errorProcess) {
return (t, u) -> {
try {
return predicate.test(t, u);
} catch (Throwable e) {
return errorProcess.apply(Try.failure(Pair.of(t, u), e));
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy