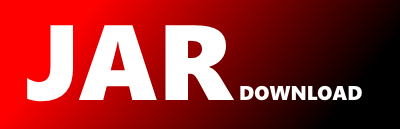
com.github.BaicProject.NowPaySdk Maven / Gradle / Ivy
package com.github.BaicProject;
import com.github.BaicProject.conf.Config;
import com.github.BaicProject.util.HttpClientUtil;
public class NowPaySdk {
public static String getToken(String sdkId,String appId) {
return HttpClientUtil.sendGet(Config.GET_TOKEN_URL,
"sdkId="+sdkId+"&appKey="+appId);
}
public static String getPorder(String sdkId,
String orderAmount,
String orderNo,
String currencyType,
String token) {
return HttpClientUtil.sendPost(Config.GET_PORDER_URL,"sdkId="+sdkId+
"&orderNo="+orderNo+
"&orderAmount="+orderAmount+
"¤cyType="+currencyType+
"&token="+token);
}
public static String selectOrderByNo(String transactionNo,
String sdkId,
String token
){
return HttpClientUtil.sendPost(Config.SELECT_BY_TRANSACTIONNO_URL,"sdkId="+sdkId+
"&transactionNo="+transactionNo+
"&token="+token);
}
public static String selectOrderByTime(String merchantId,
String beginTime,
String endTime,
String sdkId,
String token){
return HttpClientUtil.sendPost(Config.SELECT_ORDER_BY_TIME_URL,"sdkId="+sdkId+
"&beginTime="+beginTime+
"&endTime="+endTime+
"&merchantId="+merchantId+
"&token="+token);
}
public static String selectRefundByTransactionNo(String transactionNo,
String sdkId,
String token
){
return HttpClientUtil.sendPost(Config.SELECT_REFUND_BY_TRANSACTIONNO_URL,"sdkId="+sdkId+
"&transactionNo="+transactionNo+
"&token="+token);
}
public static String selectOrderByPage(Integer page,
Integer limit,
String sdkId,
String token
){
return HttpClientUtil.sendPost(Config.SELECT_ORDER_BY_PAGE_URL,"page="+page+
"&limit="+limit+
"&sdkId="+sdkId+
"&token="+token);
}
public static String selectRefundRecordByPage(Integer page,
Integer limit,
String sdkId,
String token){
return HttpClientUtil.sendPost(Config.SELECT_REFUND_RECORD_BY_PAGE_URL,"page="+page+
"&limit="+limit+
"&sdkId="+sdkId+
"&token="+token);
}
public static String refundRequest(String refundAmount,String merchantId,String transactionNo,String sdkId,String token) {
return HttpClientUtil.sendPost(Config.REFUND_REQUEST,
"refundAmount="+refundAmount+
"&merchantId="+merchantId+
"&transactionNo="+transactionNo+
"&token="+token+
"&sdkId="+sdkId);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy