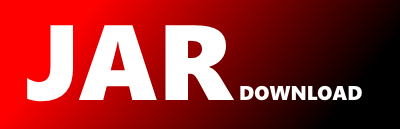
com.github.DNAProject.smartcontract.nativevm.Auth Maven / Gradle / Ivy
The newest version!
package com.github.DNAProject.smartcontract.nativevm;
import com.alibaba.fastjson.JSONObject;
import com.github.DNAProject.DnaSdk;
import com.github.DNAProject.account.Account;
import com.github.DNAProject.common.Address;
import com.github.DNAProject.common.ErrorCode;
import com.github.DNAProject.common.Helper;
import com.github.DNAProject.core.transaction.Transaction;
import com.github.DNAProject.io.BinaryReader;
import com.github.DNAProject.io.BinaryWriter;
import com.github.DNAProject.io.Serializable;
import com.github.DNAProject.sdk.exception.SDKException;
import com.github.DNAProject.smartcontract.nativevm.abi.NativeBuildParams;
import com.github.DNAProject.smartcontract.nativevm.abi.Struct;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class Auth {
private DnaSdk sdk;
private final String contractAddress = "0000000000000000000000000000000000000006";
public Auth(DnaSdk sdk) {
this.sdk = sdk;
}
public String getContractAddress() {
return contractAddress;
}
public String sendInit(String adminDnaId,String password,byte[] salt, String contractAddr,Account payerAcct,long gaslimit,long gasprice) throws Exception {
if(adminDnaId ==null || adminDnaId.equals("")){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
ByteArrayOutputStream bos = new ByteArrayOutputStream();
BinaryWriter bw = new BinaryWriter(bos);
bw.writeVarBytes(adminDnaId.getBytes());
Transaction tx = sdk.vm().makeInvokeCodeTransaction(contractAddr,"initContractAdmin",null, payerAcct.getAddressU160().toBase58(),gaslimit,gasprice);
sdk.signTx(tx,adminDnaId,password,salt);
sdk.addSign(tx,payerAcct);
boolean b = sdk.getConnect().sendRawTransaction(tx.toHexString());
if (!b) {
throw new SDKException(ErrorCode.SendRawTxError);
}
return tx.hash().toHexString();
}
/**
*
* @param adminDnaId
* @param password
* @param contractAddr
* @param newAdminDnaID
* @param keyNo
* @param payerAcct
* @param gaslimit
* @param gasprice
* @return
* @throws Exception
*/
public String sendTransfer(String adminDnaId, String password,byte[] salt,long keyNo, String contractAddr, String newAdminDnaID, Account payerAcct, long gaslimit, long gasprice) throws Exception {
if(adminDnaId ==null || adminDnaId.equals("") || contractAddr == null || contractAddr.equals("") || newAdminDnaID==null || newAdminDnaID.equals("")||payerAcct==null){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo <0 || gaslimit <0 || gasprice <0){
throw new SDKException(ErrorCode.ParamErr("keyNo or gaslimit or gasprice should not be less than 0"));
}
Transaction tx = makeTransfer(adminDnaId,contractAddr,newAdminDnaID,keyNo,payerAcct.getAddressU160().toBase58(),gaslimit,gasprice);
sdk.signTx(tx,adminDnaId,password,salt);
sdk.addSign(tx,payerAcct);
boolean b = sdk.getConnect().sendRawTransaction(tx.toHexString());
if (!b) {
throw new SDKException(ErrorCode.SendRawTxError);
}
return tx.hash().toHexString();
}
/**
*
* @param adminDnaID
* @param contractAddr
* @param newAdminDnaID
* @param keyNo
* @param payer
* @param gaslimit
* @param gasprice
* @return
* @throws SDKException
*/
public Transaction makeTransfer(String adminDnaID,String contractAddr, String newAdminDnaID,long keyNo,String payer,long gaslimit,long gasprice) throws SDKException {
if(adminDnaID ==null || adminDnaID.equals("") || contractAddr == null || contractAddr.equals("") || newAdminDnaID==null || newAdminDnaID.equals("")){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo <0 || gaslimit <0 || gasprice <0){
throw new SDKException(ErrorCode.ParamErr("keyNo or gaslimit or gasprice should not be less than 0"));
}
List list = new ArrayList();
list.add(new Struct().add(Helper.hexToBytes(contractAddr),newAdminDnaID.getBytes(),keyNo));
byte[] arg = NativeBuildParams.createCodeParamsScript(list);
Transaction tx = sdk.vm().buildNativeParams(new Address(Helper.hexToBytes(contractAddress)),"transfer",arg,payer,gaslimit,gasprice);
return tx;
}
/**
*
* @param dnaid
* @param password
* @param contractAddr
* @param funcName
* @param keyNo
* @return
* @throws Exception
*/
public String verifyToken(String dnaid,String password,byte[] salt,long keyNo, String contractAddr,String funcName) throws Exception {
if(dnaid ==null || dnaid.equals("") || password ==null || password.equals("")|| contractAddr == null || contractAddr.equals("") || funcName==null || funcName.equals("")){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo < 0){
throw new SDKException(ErrorCode.ParamErr("key or gaslimit or gas price should not be less than 0"));
}
Transaction tx = makeVerifyToken(dnaid,contractAddr,funcName,keyNo);
sdk.signTx(tx,dnaid,password,salt);
Object obj = sdk.getConnect().sendRawTransactionPreExec(tx.toHexString());
if (obj == null){
throw new SDKException(ErrorCode.OtherError("sendRawTransaction PreExec error: "));
}
return ((JSONObject)obj).getString("Result");
}
/**
*
* @param dnaid
* @param contractAddr
* @param funcName
* @param keyNo
* @return
* @throws SDKException
*/
public Transaction makeVerifyToken(String dnaid,String contractAddr,String funcName,long keyNo) throws SDKException {
if(dnaid ==null || dnaid.equals("")|| contractAddr == null || contractAddr.equals("") || funcName==null || funcName.equals("")){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo < 0){
throw new SDKException(ErrorCode.ParamErr("key or gaslimit or gas price should not be less than 0"));
}
List list = new ArrayList();
list.add(new Struct().add(Helper.hexToBytes(contractAddr),dnaid.getBytes(),funcName.getBytes(),keyNo));
byte[] arg = NativeBuildParams.createCodeParamsScript(list);
Transaction tx = sdk.vm().buildNativeParams(new Address(Helper.hexToBytes(contractAddress)),"verifyToken",arg,null,0,0);
return tx;
}
/**
*
* @param adminDnaID
* @param password
* @param contractAddr
* @param role
* @param funcName
* @param keyNo
* @param payerAcct
* @param gaslimit
* @param gasprice
* @return
* @throws Exception
*/
public String assignFuncsToRole(String adminDnaID,String password,byte[] salt, long keyNo,String contractAddr,String role,String[] funcName,Account payerAcct,long gaslimit,long gasprice) throws Exception {
if(adminDnaID ==null || adminDnaID.equals("") || contractAddr == null || contractAddr.equals("") || role==null || role.equals("") || funcName == null || funcName.length == 0||payerAcct==null){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo < 0 || gaslimit < 0 || gasprice < 0){
throw new SDKException(ErrorCode.ParamErr("keyNo or gaslimit or gas price should not be less than 0"));
}
Transaction tx = makeAssignFuncsToRole(adminDnaID,contractAddr,role,funcName,keyNo,payerAcct.getAddressU160().toBase58(),gaslimit,gasprice);
sdk.signTx(tx,adminDnaID,password,salt);
sdk.addSign(tx,payerAcct);
boolean b = sdk.getConnect().sendRawTransaction(tx.toHexString());
if(b){
return tx.hash().toHexString();
}
return null;
}
/**
*
* @param adminDnaID
* @param contractAddr
* @param role
* @param funcName
* @param keyNo
* @param payer
* @param gaslimit
* @param gasprice
* @return
* @throws SDKException
*/
public Transaction makeAssignFuncsToRole(String adminDnaID,String contractAddr,String role,String[] funcName,long keyNo,String payer,long gaslimit,long gasprice) throws SDKException {
if(adminDnaID ==null || adminDnaID.equals("") || contractAddr == null || contractAddr.equals("") || role==null || role.equals("") || funcName == null || funcName.length == 0
|| payer==null || payer.equals("")){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo < 0 || gaslimit < 0 || gasprice < 0){
throw new SDKException(ErrorCode.ParamErr("keyNo or gaslimit or gas price should not be less than 0"));
}
List list = new ArrayList();
Struct struct = new Struct();
struct.add(Helper.hexToBytes(contractAddr),adminDnaID.getBytes(),role.getBytes());
struct.add(funcName.length);
for (int i = 0; i < funcName.length; i++) {
struct.add(funcName[i]);
}
struct.add(keyNo);
list.add(struct);
byte[] arg = NativeBuildParams.createCodeParamsScript(list);
Transaction tx = sdk.vm().buildNativeParams(new Address(Helper.hexToBytes(contractAddress)),"assignFuncsToRole",arg,payer,gaslimit,gasprice);
return tx;
}
/**
*
* @param adminDnaId
* @param password
* @param contractAddr
* @param role
* @param dnaIDs
* @param keyNo
* @param payerAcct
* @param gaslimit
* @param gasprice
* @return
* @throws Exception
*/
public String assignDnaIdsToRole(String adminDnaId, String password, byte[] salt, long keyNo, String contractAddr, String role, String[] dnaIDs, Account payerAcct, long gaslimit, long gasprice) throws Exception {
if(adminDnaId == null || adminDnaId.equals("") || password==null || password.equals("") || contractAddr== null || contractAddr.equals("") ||
role == null || role.equals("") || dnaIDs==null || dnaIDs.length == 0){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo<0 || gaslimit < 0 || gasprice < 0){
throw new SDKException(ErrorCode.ParamErr("keyNo or gaslimit or gasprice should not be less than 0"));
}
Transaction tx = makeAssignDnaIDsToRole(adminDnaId,contractAddr,role,dnaIDs,keyNo,payerAcct.getAddressU160().toBase58(),gaslimit,gasprice);
sdk.signTx(tx,adminDnaId,password,salt);
sdk.addSign(tx,payerAcct);
boolean b = sdk.getConnect().sendRawTransaction(tx.toHexString());
if(b){
return tx.hash().toHexString();
}
return null;
}
/**
*
* @param adminDnaId
* @param contractAddr
* @param role
* @param dnaIDs
* @param keyNo
* @param payer
* @param gaslimit
* @param gasprice
* @return
* @throws SDKException
*/
public Transaction makeAssignDnaIDsToRole(String adminDnaId, String contractAddr, String role, String[] dnaIDs, long keyNo, String payer, long gaslimit, long gasprice) throws SDKException {
if(adminDnaId == null || adminDnaId.equals("") || contractAddr== null || contractAddr.equals("") ||
role == null || role.equals("") || dnaIDs==null || dnaIDs.length == 0){
throw new SDKException(ErrorCode.ParamErr("parameter should not be null"));
}
if(keyNo <0 || gaslimit < 0 || gasprice < 0){
throw new SDKException(ErrorCode.ParamErr("keyNo or gaslimit or gasprice should not be less than 0"));
}
byte[][] dnaId = new byte[dnaIDs.length][];
for(int i=0; i< dnaIDs.length ; i++){
dnaId[i] = dnaIDs[i].getBytes();
}
List list = new ArrayList();
Struct struct = new Struct();
struct.add(Helper.hexToBytes(contractAddr),adminDnaId.getBytes(),role.getBytes());
struct.add(dnaId.length);
for(int i =0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy