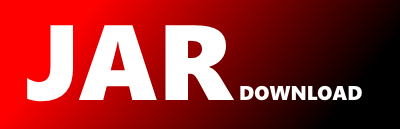
com.github.DNAProject.smartcontract.nativevm.abi.AbiInfo Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2018 The DNA Authors
* This file is part of The DNA library.
*
* The DNA is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* The DNA is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with The DNA. If not, see .
*
*/
package com.github.DNAProject.smartcontract.nativevm.abi;
import com.alibaba.fastjson.JSON;
import java.util.List;
/**
* smartcode abi information
*/
public class AbiInfo {
public String hash;
public String entrypoint;
public List functions;
public List events;
public String getHash() {
return hash;
}
public void setHash(String hash) {
this.hash = hash;
}
public String getEntrypoint() {
return entrypoint;
}
public void setEntrypoint(String entrypoint) {
this.entrypoint = entrypoint;
}
public List getFunctions() {
return functions;
}
public void getFunctions(List functions) {
this.functions = functions;
}
public List getEvents() {
return events;
}
public void getEvents(List events) {
this.events = events;
}
public AbiFunction getFunction(String name) {
for (AbiFunction e : functions) {
if (e.getName().equals(name)) {
return e;
}
}
return null;
}
public AbiEvent getEvent(String name) {
for (AbiEvent e : events) {
if (e.getName().equals(name)) {
return e;
}
}
return null;
}
public void clearFunctionsParamsValue() {
for (AbiFunction e : functions) {
e.clearParamsValue();
}
}
public void clearEventsParamsValue() {
for (AbiEvent e : events) {
e.clearParamsValue();
}
}
public void removeFunctionParamsValue(String name) {
for (AbiFunction e : functions) {
if (e.getName().equals(name)) {
e.clearParamsValue();
}
}
}
public void removeEventParamsValue(String name) {
for (AbiEvent e : events) {
if (e.getName().equals(name)) {
e.clearParamsValue();
}
}
}
@Override
public String toString() {
return JSON.toJSONString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy