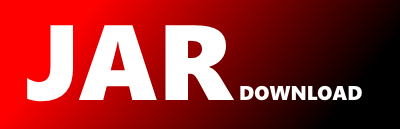
com.github.GBSEcom.api.AuthenticationApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.api;
import com.github.GBSEcom.client.ApiCallback;
import com.github.GBSEcom.client.ApiClient;
import com.github.GBSEcom.client.ApiException;
import com.github.GBSEcom.client.ApiResponse;
import com.github.GBSEcom.client.Configuration;
import com.github.GBSEcom.client.Pair;
import com.github.GBSEcom.client.ProgressRequestBody;
import com.github.GBSEcom.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.github.GBSEcom.model.AccessTokenResponse;
import com.github.GBSEcom.model.ErrorResponse;
import com.github.GBSEcom.model.TransactionResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AuthenticationApi {
private ApiClient apiClient;
public AuthenticationApi() {
this(Configuration.getDefaultApiClient());
}
public AuthenticationApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for v1AuthenticationAccessTokensPost
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call v1AuthenticationAccessTokensPostCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/authentication/access-tokens";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
if (clientRequestId != null)
localVarHeaderParams.put("Client-Request-Id", apiClient.parameterToString(clientRequestId));
if (apiKey != null)
localVarHeaderParams.put("Api-Key", apiClient.parameterToString(apiKey));
if (timestamp != null)
localVarHeaderParams.put("Timestamp", apiClient.parameterToString(timestamp));
if (messageSignature != null)
localVarHeaderParams.put("Message-Signature", apiClient.parameterToString(messageSignature));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call v1AuthenticationAccessTokensPostValidateBeforeCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling v1AuthenticationAccessTokensPost(Async)");
}
// verify the required parameter 'clientRequestId' is set
if (clientRequestId == null) {
throw new ApiException("Missing the required parameter 'clientRequestId' when calling v1AuthenticationAccessTokensPost(Async)");
}
// verify the required parameter 'apiKey' is set
if (apiKey == null) {
throw new ApiException("Missing the required parameter 'apiKey' when calling v1AuthenticationAccessTokensPost(Async)");
}
// verify the required parameter 'timestamp' is set
if (timestamp == null) {
throw new ApiException("Missing the required parameter 'timestamp' when calling v1AuthenticationAccessTokensPost(Async)");
}
// verify the required parameter 'messageSignature' is set
if (messageSignature == null) {
throw new ApiException("Missing the required parameter 'messageSignature' when calling v1AuthenticationAccessTokensPost(Async)");
}
com.squareup.okhttp.Call call = v1AuthenticationAccessTokensPostCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, progressListener, progressRequestListener);
return call;
}
/**
* Generate an access token for user authentication
* This is the access token generation call for authorizing subsequent financial transactions. A valid access token is required for web client requests.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @return AccessTokenResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public AccessTokenResponse v1AuthenticationAccessTokensPost(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature) throws ApiException {
ApiResponse resp = v1AuthenticationAccessTokensPostWithHttpInfo(contentType, clientRequestId, apiKey, timestamp, messageSignature);
return resp.getData();
}
/**
* Generate an access token for user authentication
* This is the access token generation call for authorizing subsequent financial transactions. A valid access token is required for web client requests.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @return ApiResponse<AccessTokenResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse v1AuthenticationAccessTokensPostWithHttpInfo(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature) throws ApiException {
com.squareup.okhttp.Call call = v1AuthenticationAccessTokensPostValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Generate an access token for user authentication (asynchronously)
* This is the access token generation call for authorizing subsequent financial transactions. A valid access token is required for web client requests.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call v1AuthenticationAccessTokensPostAsync(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = v1AuthenticationAccessTokensPostValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy