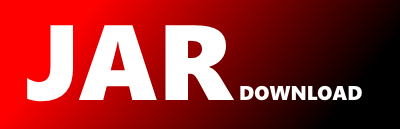
com.github.GBSEcom.api.PaymentApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of first-data-gateway Show documentation
Show all versions of first-data-gateway Show documentation
Java SDK to be used with a First Data Gateway account. This SDK has been created and packaged to offer the easiest way to integrate your application into the First Data Gateway. This SDK gives you the ability to run transactions such as sales, preauthorizations, postauthorizations, credits, voids, and returns; transaction inquiries; setting up scheduled payments and much more.
/*
* Payment Gateway API Specification
* Payment Gateway API for payment processing.
*
* OpenAPI spec version: 0.0.1
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.github.GBSEcom.api;
import com.github.GBSEcom.client.ApiCallback;
import com.github.GBSEcom.client.ApiClient;
import com.github.GBSEcom.client.ApiException;
import com.github.GBSEcom.client.ApiResponse;
import com.github.GBSEcom.client.Configuration;
import com.github.GBSEcom.client.Pair;
import com.github.GBSEcom.client.ProgressRequestBody;
import com.github.GBSEcom.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import com.github.GBSEcom.model.ErrorResponse;
import com.github.GBSEcom.model.PrimaryTransaction;
import com.github.GBSEcom.model.SecondaryTransaction;
import com.github.GBSEcom.model.TransactionResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PaymentApi {
private ApiClient apiClient;
public PaymentApi() {
this(Configuration.getDefaultApiClient());
}
public PaymentApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for performPaymentPostAuthorisation
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call performPaymentPostAuthorisationCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = payload;
// create path and map variables
String localVarPath = "/v1/payments/{transaction-id}/postauth"
.replaceAll("\\{" + "transaction-id" + "\\}", apiClient.escapeString(transactionId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (storeId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("storeId", storeId));
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
if (clientRequestId != null)
localVarHeaderParams.put("Client-Request-Id", apiClient.parameterToString(clientRequestId));
if (apiKey != null)
localVarHeaderParams.put("Api-Key", apiClient.parameterToString(apiKey));
if (timestamp != null)
localVarHeaderParams.put("Timestamp", apiClient.parameterToString(timestamp));
if (messageSignature != null)
localVarHeaderParams.put("Message-Signature", apiClient.parameterToString(messageSignature));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call performPaymentPostAuthorisationValidateBeforeCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling performPaymentPostAuthorisation(Async)");
}
// verify the required parameter 'clientRequestId' is set
if (clientRequestId == null) {
throw new ApiException("Missing the required parameter 'clientRequestId' when calling performPaymentPostAuthorisation(Async)");
}
// verify the required parameter 'apiKey' is set
if (apiKey == null) {
throw new ApiException("Missing the required parameter 'apiKey' when calling performPaymentPostAuthorisation(Async)");
}
// verify the required parameter 'timestamp' is set
if (timestamp == null) {
throw new ApiException("Missing the required parameter 'timestamp' when calling performPaymentPostAuthorisation(Async)");
}
// verify the required parameter 'messageSignature' is set
if (messageSignature == null) {
throw new ApiException("Missing the required parameter 'messageSignature' when calling performPaymentPostAuthorisation(Async)");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new ApiException("Missing the required parameter 'transactionId' when calling performPaymentPostAuthorisation(Async)");
}
// verify the required parameter 'payload' is set
if (payload == null) {
throw new ApiException("Missing the required parameter 'payload' when calling performPaymentPostAuthorisation(Async)");
}
com.squareup.okhttp.Call call = performPaymentPostAuthorisationCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId, progressListener, progressRequestListener);
return call;
}
/**
* Use this to capture/complete a transaction. Partial postauths are allowed.
* This can be used for postauth and partial postauths.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return TransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TransactionResponse performPaymentPostAuthorisation(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId) throws ApiException {
ApiResponse resp = performPaymentPostAuthorisationWithHttpInfo(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId);
return resp.getData();
}
/**
* Use this to capture/complete a transaction. Partial postauths are allowed.
* This can be used for postauth and partial postauths.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return ApiResponse<TransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse performPaymentPostAuthorisationWithHttpInfo(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId) throws ApiException {
com.squareup.okhttp.Call call = performPaymentPostAuthorisationValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Use this to capture/complete a transaction. Partial postauths are allowed. (asynchronously)
* This can be used for postauth and partial postauths.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call performPaymentPostAuthorisationAsync(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = performPaymentPostAuthorisationValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for primaryPaymentTransaction
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param payload Primary Transaction request (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call primaryPaymentTransactionCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, PrimaryTransaction payload, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = payload;
// create path and map variables
String localVarPath = "/v1/payments";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
if (clientRequestId != null)
localVarHeaderParams.put("Client-Request-Id", apiClient.parameterToString(clientRequestId));
if (apiKey != null)
localVarHeaderParams.put("Api-Key", apiClient.parameterToString(apiKey));
if (timestamp != null)
localVarHeaderParams.put("Timestamp", apiClient.parameterToString(timestamp));
if (messageSignature != null)
localVarHeaderParams.put("Message-Signature", apiClient.parameterToString(messageSignature));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call primaryPaymentTransactionValidateBeforeCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, PrimaryTransaction payload, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling primaryPaymentTransaction(Async)");
}
// verify the required parameter 'clientRequestId' is set
if (clientRequestId == null) {
throw new ApiException("Missing the required parameter 'clientRequestId' when calling primaryPaymentTransaction(Async)");
}
// verify the required parameter 'apiKey' is set
if (apiKey == null) {
throw new ApiException("Missing the required parameter 'apiKey' when calling primaryPaymentTransaction(Async)");
}
// verify the required parameter 'timestamp' is set
if (timestamp == null) {
throw new ApiException("Missing the required parameter 'timestamp' when calling primaryPaymentTransaction(Async)");
}
// verify the required parameter 'messageSignature' is set
if (messageSignature == null) {
throw new ApiException("Missing the required parameter 'messageSignature' when calling primaryPaymentTransaction(Async)");
}
// verify the required parameter 'payload' is set
if (payload == null) {
throw new ApiException("Missing the required parameter 'payload' when calling primaryPaymentTransaction(Async)");
}
com.squareup.okhttp.Call call = primaryPaymentTransactionCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, payload, progressListener, progressRequestListener);
return call;
}
/**
* Generate a primary transaction
* Use this to originate a financial transaction, like a sale, preauthorization, or credit.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param payload Primary Transaction request (required)
* @return TransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TransactionResponse primaryPaymentTransaction(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, PrimaryTransaction payload) throws ApiException {
ApiResponse resp = primaryPaymentTransactionWithHttpInfo(contentType, clientRequestId, apiKey, timestamp, messageSignature, payload);
return resp.getData();
}
/**
* Generate a primary transaction
* Use this to originate a financial transaction, like a sale, preauthorization, or credit.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param payload Primary Transaction request (required)
* @return ApiResponse<TransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse primaryPaymentTransactionWithHttpInfo(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, PrimaryTransaction payload) throws ApiException {
com.squareup.okhttp.Call call = primaryPaymentTransactionValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, payload, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Generate a primary transaction (asynchronously)
* Use this to originate a financial transaction, like a sale, preauthorization, or credit.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param payload Primary Transaction request (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call primaryPaymentTransactionAsync(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, PrimaryTransaction payload, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = primaryPaymentTransactionValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, payload, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for returnTransaction
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call returnTransactionCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = payload;
// create path and map variables
String localVarPath = "/v1/payments/{transaction-id}/return"
.replaceAll("\\{" + "transaction-id" + "\\}", apiClient.escapeString(transactionId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (storeId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("storeId", storeId));
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
if (clientRequestId != null)
localVarHeaderParams.put("Client-Request-Id", apiClient.parameterToString(clientRequestId));
if (apiKey != null)
localVarHeaderParams.put("Api-Key", apiClient.parameterToString(apiKey));
if (timestamp != null)
localVarHeaderParams.put("Timestamp", apiClient.parameterToString(timestamp));
if (messageSignature != null)
localVarHeaderParams.put("Message-Signature", apiClient.parameterToString(messageSignature));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call returnTransactionValidateBeforeCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling returnTransaction(Async)");
}
// verify the required parameter 'clientRequestId' is set
if (clientRequestId == null) {
throw new ApiException("Missing the required parameter 'clientRequestId' when calling returnTransaction(Async)");
}
// verify the required parameter 'apiKey' is set
if (apiKey == null) {
throw new ApiException("Missing the required parameter 'apiKey' when calling returnTransaction(Async)");
}
// verify the required parameter 'timestamp' is set
if (timestamp == null) {
throw new ApiException("Missing the required parameter 'timestamp' when calling returnTransaction(Async)");
}
// verify the required parameter 'messageSignature' is set
if (messageSignature == null) {
throw new ApiException("Missing the required parameter 'messageSignature' when calling returnTransaction(Async)");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new ApiException("Missing the required parameter 'transactionId' when calling returnTransaction(Async)");
}
// verify the required parameter 'payload' is set
if (payload == null) {
throw new ApiException("Missing the required parameter 'payload' when calling returnTransaction(Async)");
}
com.squareup.okhttp.Call call = returnTransactionCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId, progressListener, progressRequestListener);
return call;
}
/**
* Return/refund a transaction.
* Use this to return/refund an existing transaction. Partial returns are allowed.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return TransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TransactionResponse returnTransaction(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId) throws ApiException {
ApiResponse resp = returnTransactionWithHttpInfo(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId);
return resp.getData();
}
/**
* Return/refund a transaction.
* Use this to return/refund an existing transaction. Partial returns are allowed.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return ApiResponse<TransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse returnTransactionWithHttpInfo(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId) throws ApiException {
com.squareup.okhttp.Call call = returnTransactionValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Return/refund a transaction. (asynchronously)
* Use this to return/refund an existing transaction. Partial returns are allowed.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param payload (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call returnTransactionAsync(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, SecondaryTransaction payload, String storeId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = returnTransactionValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, payload, storeId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for transactionInquiry
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call transactionInquiryCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/payments/{transaction-id}"
.replaceAll("\\{" + "transaction-id" + "\\}", apiClient.escapeString(transactionId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (storeId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("storeId", storeId));
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
if (clientRequestId != null)
localVarHeaderParams.put("Client-Request-Id", apiClient.parameterToString(clientRequestId));
if (apiKey != null)
localVarHeaderParams.put("Api-Key", apiClient.parameterToString(apiKey));
if (timestamp != null)
localVarHeaderParams.put("Timestamp", apiClient.parameterToString(timestamp));
if (messageSignature != null)
localVarHeaderParams.put("Message-Signature", apiClient.parameterToString(messageSignature));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call transactionInquiryValidateBeforeCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling transactionInquiry(Async)");
}
// verify the required parameter 'clientRequestId' is set
if (clientRequestId == null) {
throw new ApiException("Missing the required parameter 'clientRequestId' when calling transactionInquiry(Async)");
}
// verify the required parameter 'apiKey' is set
if (apiKey == null) {
throw new ApiException("Missing the required parameter 'apiKey' when calling transactionInquiry(Async)");
}
// verify the required parameter 'timestamp' is set
if (timestamp == null) {
throw new ApiException("Missing the required parameter 'timestamp' when calling transactionInquiry(Async)");
}
// verify the required parameter 'messageSignature' is set
if (messageSignature == null) {
throw new ApiException("Missing the required parameter 'messageSignature' when calling transactionInquiry(Async)");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new ApiException("Missing the required parameter 'transactionId' when calling transactionInquiry(Async)");
}
com.squareup.okhttp.Call call = transactionInquiryCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId, progressListener, progressRequestListener);
return call;
}
/**
* Retrieve the state of a transaction
* Use this query to get the current state of an existing transaction.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return TransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TransactionResponse transactionInquiry(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId) throws ApiException {
ApiResponse resp = transactionInquiryWithHttpInfo(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId);
return resp.getData();
}
/**
* Retrieve the state of a transaction
* Use this query to get the current state of an existing transaction.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return ApiResponse<TransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse transactionInquiryWithHttpInfo(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId) throws ApiException {
com.squareup.okhttp.Call call = transactionInquiryValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Retrieve the state of a transaction (asynchronously)
* Use this query to get the current state of an existing transaction.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call transactionInquiryAsync(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = transactionInquiryValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for voidTransaction
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call voidTransactionCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/payments/{transaction-id}/void"
.replaceAll("\\{" + "transaction-id" + "\\}", apiClient.escapeString(transactionId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (storeId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("storeId", storeId));
Map localVarHeaderParams = new HashMap();
if (contentType != null)
localVarHeaderParams.put("Content-Type", apiClient.parameterToString(contentType));
if (clientRequestId != null)
localVarHeaderParams.put("Client-Request-Id", apiClient.parameterToString(clientRequestId));
if (apiKey != null)
localVarHeaderParams.put("Api-Key", apiClient.parameterToString(apiKey));
if (timestamp != null)
localVarHeaderParams.put("Timestamp", apiClient.parameterToString(timestamp));
if (messageSignature != null)
localVarHeaderParams.put("Message-Signature", apiClient.parameterToString(messageSignature));
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call voidTransactionValidateBeforeCall(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling voidTransaction(Async)");
}
// verify the required parameter 'clientRequestId' is set
if (clientRequestId == null) {
throw new ApiException("Missing the required parameter 'clientRequestId' when calling voidTransaction(Async)");
}
// verify the required parameter 'apiKey' is set
if (apiKey == null) {
throw new ApiException("Missing the required parameter 'apiKey' when calling voidTransaction(Async)");
}
// verify the required parameter 'timestamp' is set
if (timestamp == null) {
throw new ApiException("Missing the required parameter 'timestamp' when calling voidTransaction(Async)");
}
// verify the required parameter 'messageSignature' is set
if (messageSignature == null) {
throw new ApiException("Missing the required parameter 'messageSignature' when calling voidTransaction(Async)");
}
// verify the required parameter 'transactionId' is set
if (transactionId == null) {
throw new ApiException("Missing the required parameter 'transactionId' when calling voidTransaction(Async)");
}
com.squareup.okhttp.Call call = voidTransactionCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId, progressListener, progressRequestListener);
return call;
}
/**
* Reverse a previous action on an existing transaction
* Use this to reverse a postauth/completion, credit, preauth, or sale.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return TransactionResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TransactionResponse voidTransaction(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId) throws ApiException {
ApiResponse resp = voidTransactionWithHttpInfo(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId);
return resp.getData();
}
/**
* Reverse a previous action on an existing transaction
* Use this to reverse a postauth/completion, credit, preauth, or sale.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @return ApiResponse<TransactionResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse voidTransactionWithHttpInfo(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId) throws ApiException {
com.squareup.okhttp.Call call = voidTransactionValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* Reverse a previous action on an existing transaction (asynchronously)
* Use this to reverse a postauth/completion, credit, preauth, or sale.
* @param contentType content type (required)
* @param clientRequestId A client-generated ID for request tracking and signature creation, unique per request. This is also used for idempotency control. We recommend 128-bit UUID format. (required)
* @param apiKey (required)
* @param timestamp Epoch timestamp in milliseconds in the request from a client system. Used for Message Signature generation and time limit (5 mins). (required)
* @param messageSignature Used to ensure the request has not been tampered with during transmission. The Message-Signature is the Base64 encoded HMAC hash (SHA256 algorithm with the API Secret as the key.) For more information, refer to the supporting documentation on the Developer Portal. (required)
* @param transactionId Gateway transaction identifier as returned in the parameter ipgTransactionId (required)
* @param storeId an optional outlet id for clients that support multiple store in the same developer app (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call voidTransactionAsync(String contentType, String clientRequestId, String apiKey, Long timestamp, String messageSignature, String transactionId, String storeId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = voidTransactionValidateBeforeCall(contentType, clientRequestId, apiKey, timestamp, messageSignature, transactionId, storeId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy